ZennoPoster Scripts: Mastering Automation for Digital Tasks
Understanding ZennoPoster Scripts
ZennoPoster scripts are powerful automation tools that enable users to create sophisticated sequences for performing various digital tasks. These scripts serve as the backbone for automating web interactions, data extraction, and complex online processes. By leveraging ZennoPoster’s scripting capabilities, users can significantly reduce manual effort and increase efficiency in their digital workflows.
- Understanding ZennoPoster Scripts
- The Anatomy of ZennoPoster Scripts
- Advanced Techniques in ZennoPoster Scripting
- Optimizing ZennoPoster Scripts for Performance
- Integrating External Libraries and APIs
- Best Practices for ZennoPoster Script Development
- Conclusion: Harnessing the Full Potential of ZennoPoster Scripts
The Anatomy of ZennoPoster Scripts
ZennoPoster scripts are composed of several key elements that work together to create effective automation sequences. Understanding these components is crucial for developing robust and efficient scripts:
Variables: Dynamic Data Storage
Variables act as containers for storing and manipulating data within scripts. They play a vital role in making scripts flexible and adaptable to different scenarios. For example:
string searchTerm = "ZennoPoster automation";
project.Variables["SearchQuery"] = searchTerm;
This code snippet demonstrates how to declare a variable and store it in the project’s variable collection for later use.
Actions: Building Blocks of Automation
Actions are predefined operations that perform specific tasks within the script. ZennoPoster offers a wide range of actions, including:
- Browser navigation
- Element interaction (clicking, typing, selecting)
- Data extraction
- File operations
Example of a navigation action:
instance.NavigateURL("https://example.com");
This line instructs the browser to navigate to the specified URL.
Conditions: Decision-Making in Scripts
Conditions allow scripts to make decisions based on certain criteria. They enable the creation of branching logic, making scripts more intelligent and adaptable. For instance:
if (instance.WaitElement("//button[@id='submit']", 10000))
{
instance.Click("//button[@id='submit']");
}
else
{
project.SendInfoToLog("Submit button not found", true);
}
This code waits for a submit button to appear and clicks it if found, or logs an error message if not found within the specified timeout.
Advanced Techniques in ZennoPoster Scripting
To create more powerful and efficient ZennoPoster scripts, consider implementing these advanced techniques:
Loops: Repeating Actions Efficiently
Loops allow for the repetition of actions, which is particularly useful for processing multiple items or paginated content. Example:
for (int i = 0; i < 5; i++)
{
instance.Click("//button[@class='next-page']");
project.Sleep(2000);
// Perform actions on each page
}
This loop navigates through five pages, clicking the “next” button and waiting between each click.
Functions: Modular Code Organization
Functions help organize code into reusable blocks, improving script maintainability and readability. For example:
void ExtractProductInfo()
{
string productName = instance.FindElement("//h1[@class='product-title']").Text;
string productPrice = instance.FindElement("//span[@class='price']").Text;
project.Variables["ProductData"] += productName + "," + productPrice + Environment.NewLine;
}
// Usage
ExtractProductInfo();
This function extracts product information and can be called multiple times throughout the script.
Error Handling: Ensuring Script Robustness
Implementing error handling mechanisms helps scripts recover from unexpected situations and continue execution. Example:
try
{
instance.Click("//button[@id='risky-action']");
}
catch (Exception ex)
{
project.SendWarningToLog("Error occurred: " + ex.Message);
// Perform alternative action or continue script
}
This try-catch block attempts to perform a potentially risky action and logs a warning if an error occurs.
Optimizing ZennoPoster Scripts for Performance
To enhance the efficiency of your ZennoPoster scripts, consider these optimization strategies:
- Minimize Wait Times: Use dynamic waits instead of fixed delays when possible.
- Utilize Caching: Store frequently accessed data in variables to reduce redundant operations.
- Implement Parallel Processing: Use multiple instances to perform tasks concurrently when appropriate.
- Optimize Selectors: Use efficient and specific element selectors to improve script speed and reliability.
Example of optimized element selection:
// Less efficient
instance.FindElement("//div/span[contains(@class, 'price')]");
// More efficient
instance.FindElement("//span[@class='product-price']");
Integrating External Libraries and APIs
ZennoPoster scripts can be extended by integrating external libraries and APIs, expanding their capabilities beyond built-in functions. This allows for more complex operations such as advanced data processing or interaction with external services.
Example of using an external HTTP library:
using System.Net.Http;
// ...
async Task<string> FetchDataFromAPI(string apiUrl)
{
using (var client = new HttpClient())
{
HttpResponseMessage response = await client.GetAsync(apiUrl);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
}
// Usage
string apiData = await FetchDataFromAPI("https://api.example.com/data");
project.Variables["APIResponse"] = apiData;
This example demonstrates how to make an asynchronous API call and store the response in a project variable.
Best Practices for ZennoPoster Script Development
To ensure the creation of high-quality, maintainable ZennoPoster scripts, adhere to these best practices:
- Modular Design: Break down complex tasks into smaller, reusable functions.
- Consistent Naming: Use clear and consistent naming conventions for variables and functions.
- Comprehensive Logging: Implement detailed logging to facilitate debugging and monitoring.
- Version Control: Utilize version control systems to track changes and collaborate with team members.
- Regular Testing: Continuously test scripts in various scenarios to ensure reliability.
Example of implementing comprehensive logging:
void LogScriptProgress(string message, string level = "Info")
{
string timestamp = DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss");
string logMessage = $"[{timestamp}] [{level}] {message}";
project.SendInfoToLog(logMessage, true);
if (level == "Error")
{
project.SendErrorToLog(logMessage);
}
}
// Usage
LogScriptProgress("Starting product extraction process");
// ... perform extraction ...
LogScriptProgress("Extraction complete. Items processed: " + itemCount);
This function provides a standardized way to log messages with timestamps and severity levels.
Conclusion: Harnessing the Full Potential of ZennoPoster Scripts
Mastering ZennoPoster scripts opens up a world of automation possibilities, enabling users to tackle complex digital tasks with efficiency and precision. By understanding the core components, implementing advanced techniques, and following best practices, developers can create powerful, scalable, and maintainable automation solutions. As the digital landscape continues to evolve, the ability to craft effective ZennoPoster scripts remains an invaluable skill for professionals seeking to streamline their online operations and drive productivity to new heights.
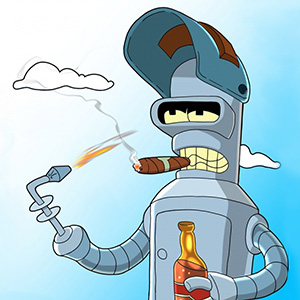
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.