Comprehensive Guide to XML Parsing
XML parsing refers to the process of analyzing and extracting data from XML documents. As a fundamental capability enabling utilization of XML data, parsing is a vital competency for software engineers and technology specialists across sectors. This definitive reference thoroughly covers XML parsing, encompassing core concepts, available techniques, common difficulties, and recommended procedures.
Understanding XML Parsing
XML parsing involves traversing an XML document, identifying its elements and attributes, and extracting relevant data for further processing. The parser analyzes the document’s structure according to XML syntax rules and breaks it into manageable chunks of information.
Key aspects of XML parsing include:
- Extracting data – Retrieving specific values and text content from elements and attributes in an XML document.
- Validating syntax – Checking that the document adheres to XML well-formedness rules and confirming the use of valid XML markup.
- Supporting namespaces – Handling XML namespaces by associating elements and attributes with namespace URIs.
- Navigating structure – Traversing the tree-based hierarchical structure of XML data through various parsing techniques.
Robust and efficient parsing is essential for unlocking the value of XML data across applications like web services, business-to-business (B2B) data transfers, and content management systems. The approach to XML parsing depends on the use case and other technical factors.
XML Parsing Approaches
There are two main strategies for parsing XML:
Tree-Based Parsing
Tree-based APIs represent an XML document as a tree data structure, with various methods available for navigating and searching. This allows easy exploration of the element hierarchy. Examples include:
- Document Object Model (DOM) – Platform-independent standard for representing XML documents for dynamic scripting.
- Simple API for XML (SAX) – Event-driven sequential access parsing. Efficient for large files or streams.
- XML Pull Parsing – Pull-based stream parsing where the application (parser consumer) drives the process through iterations.
Tree-based parsing enables developers to traverse an XML document in any direction to lookup and extract data. However, it requires the entire XML structure to be loaded in memory which can limit scalability for large datasets.
Stream-Based Parsing
Stream-based parsing sequentially analyzes an XML document while allowing only limited lookahead and no retracing of steps. This method uses far less memory but only allows single-pass forward-only parsing. Examples include:
- StAX (Streaming API for XML) – Standard pull-parsing stream API integrated with the Java language.
- XML Event Streams – Forward-only stream reading with an event-driven interface.
For large volumes of XML data, the stream-based approach greatly reduces memory overhead and aims for better performance. However, it involves more complex programming, as developers cannot freely traverse the XML tree.
Hybrid Solutions
Hybrid XML parsing combines both tree-based and stream-based features for added flexibility. Libraries like VTD-XML allow indexed random access into streamed documents stored in memory for efficient data extraction without fully materializing the XML structure.
So when choosing a parsing technique, consider factors like XML dataset size, application requirements, efficiency needs and developer skills.
Challenges with XML Parsing
While XML provides a structured data format, real-world documents present many parsing intricacies:
- Invalid markup – Errors in document syntax, semantics or vocabulary usage lead to parser failures. Rigorous error checking is vital.
- Complex hierarchies – Deeply nested elements, large variations in structure, and namespace references significantly increase processing complexity.
- Streaming limitations – Forward-only approaches limit search capabilities and require retaining minimal document state.
- Performance overheads – Memory usage, I/O bottlenecks and CPU load need careful tuning for efficiency, especially on large datasets.
- Supporting evolution – New XML vocabularies and data sources may require changes to parse logic. Extensibility helps minimize rework.
By following XML and parsing best practices, these issues can be effectively managed.
Best Practices for XML Parsing
For smooth and reliable XML processing:
- Validate all documents against XML schemas to avoid unexpected errors.
- Use namespace-aware parsing routines for handling namespace prefix mappings.
- Employ streaming parsers like StAX for scalability on large documents and datasets.
- Implement efficient document indexing and query mechanisms for searchability.
- Design flexible parse handlers allowing easy extensions for new vocabularies.
- Analyze performance bottlenecks and tune configurations for optimal resource utilization.
Adhering to official XML specifications and leveraging mature parsing libraries reduces many coding complexities and reliability headaches.
Conclusion
Effective XML parsing requires understanding approaches like DOM, SAX, pull parsing and stream parsing to match solution requirements with technique capabilities and limitations. Planning for scalability, extensibility and reliability is key for maintaining robust XML processing capabilities. Mastering XML parsing best practices delivers efficiency along with the full power of structured data in XML format across today’s integration-driven digital landscape.
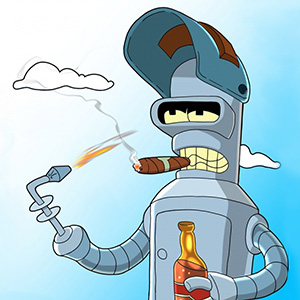
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.