XML Data Parsing Python – A Comprehensive Guide
Introduction to XML Data Parsing
In today’s rapidly evolving technological landscape, XML data parsing has become a cornerstone for professionals and enthusiasts worldwide. By integrating strategic approaches with practical applications, XML parsing empowers developers and data scientists alike to address complex challenges and achieve meaningful outcomes in data processing workflows.
Consider Thomas, a software engineer who faced significant obstacles processing data from legacy systems. By adopting Python-based XML data parsing techniques, he transformed his workflow, achieving a remarkable 40% increase in data processing efficiency within months. Such transformations are not isolated; they reflect a broader trend where XML parsing drives tangible results across diverse sectors.
This comprehensive guide delves into the multifaceted aspects of XML data parsing with Python, covering its historical evolution, practical applications, tools, challenges, and competitive strategies. Designed to deliver maximum value, it equips professionals and enthusiasts with actionable insights to thrive in today’s dynamic data processing environment.
- Understand the historical context and significance of XML data parsing
- Explore real-world applications and their impact
- Master Python-specific tools and libraries for XML processing
- Discover essential strategies to optimize outcomes
- Learn advanced techniques through practical examples
Why XML Data Parsing Matters
XML data parsing represents a transformative paradigm that delivers measurable benefits to professionals and enthusiasts worldwide. By facilitating informed decision-making and fostering innovation, it addresses critical needs in today’s competitive landscape. As industries evolve, XML parsing remains indispensable for achieving strategic objectives in data processing and integration.
According to recent industry analyses, organizations leveraging Python-based XML data parsing reported significant improvements in operational efficiency, underscoring its relevance. From enhancing productivity to enabling scalability, its impact is profound and far-reaching across various domains.
Key advantages include:
- Enhanced Efficiency: Streamlines complex document processing, reducing time and resource expenditure
- Data-Driven Decisions: Provides accurate insights from structured data for strategic planning
- Interoperability: Facilitates seamless data exchange between disparate systems
- Scalability: Adapts to handling increasing volumes of XML documents
- Preservation of Structure: Maintains hierarchical relationships in complex data
History and Evolution of XML Data Parsing
The journey of XML data parsing reflects a rich history of innovation and adaptation. Emerging from early SGML roots in the 1980s, XML itself was standardized by the W3C in 1998, establishing a foundation for structured data exchange that would transform information processing across industries.
The evolution of XML parsing techniques has been closely tied to programming language advancements. In the Python ecosystem specifically, XML parsing capabilities have grown from basic processing to sophisticated libraries that handle complex validation, transformation, and querying operations.
Key milestones in the evolution of XML parsing in Python include:
- Initial Development (Late 1990s): Basic XML processing capabilities in Python
- minidom and SAX (Early 2000s): Introduction of DOM and event-based parsing approaches
- ElementTree Integration (2005): Fredrik Lundh’s ElementTree became part of the Python Standard Library
- lxml Emergence (2005+): Combining ElementTree API with libxml2 and libxslt performance
- XPath and XSLT Support (2010+): Advanced query and transformation capabilities
- Modern Integration: Seamless incorporation with data science tools like Pandas
This evolution has transformed XML parsing from a specialized skill to an essential component in the modern developer’s toolkit, particularly for those working with data integration, configuration management, and web services.
Python Libraries for XML Parsing
Python offers several powerful libraries for XML data parsing, each with distinct advantages for different use cases. Understanding these options helps developers select the most appropriate tool for their specific requirements.
Built-in XML Libraries
Python’s standard library includes robust XML processing capabilities:
xml.etree.ElementTree
ElementTree provides a simple and efficient API for parsing and creating XML data. It’s lightweight, intuitive, and sufficient for most common XML processing tasks.
import xml.etree.ElementTree as ET
# Parse XML from file
tree = ET.parse('sample.xml')
root = tree.getroot()
# Iterate through elements
for child in root:
print(child.tag, child.attrib)
# Find elements
for element in root.findall('./child/grandchild'):
print(element.text)
xml.dom.minidom
The minidom implementation provides a minimal DOM implementation that’s useful when compatibility with the W3C DOM API is required.
from xml.dom import minidom
# Parse XML
doc = minidom.parse('sample.xml')
# Access elements
items = doc.getElementsByTagName('item')
for item in items:
print(item.getAttribute('name'))
xml.sax
SAX (Simple API for XML) provides an event-driven API that’s memory-efficient for processing large XML files.
import xml.sax
class MyHandler(xml.sax.ContentHandler):
def startElement(self, tag, attributes):
print(f"Start element: {tag}")
def endElement(self, tag):
print(f"End element: {tag}")
def characters(self, content):
if content.strip():
print(f"Content: {content.strip()}")
parser = xml.sax.make_parser()
parser.setContentHandler(MyHandler())
parser.parse('sample.xml')
Third-Party Libraries
lxml
lxml combines the simplicity of ElementTree with the power of libxml2 and libxslt, offering superior performance and advanced features like XPath, XSLT, and validation.
from lxml import etree
# Parse XML
tree = etree.parse('sample.xml')
# XPath queries
results = tree.xpath('//element[@attribute="value"]')
for result in results:
print(result.text)
# XSLT transformation
transform = etree.XSLT(etree.parse('transform.xsl'))
result = transform(tree)
print(etree.tostring(result))
BeautifulSoup with lxml
While primarily designed for HTML, BeautifulSoup can work with XML when paired with the lxml parser, offering a forgiving interface for messy XML.
from bs4 import BeautifulSoup
# Parse XML with lxml parser
with open('sample.xml', 'r') as file:
soup = BeautifulSoup(file, 'lxml-xml')
# Find elements
elements = soup.find_all('element')
for element in elements:
print(element.get('attribute'))
Selecting the right library depends on factors including:
- XML document size and complexity
- Performance requirements
- Need for validation or transformation
- Integration requirements with other systems
- Developer familiarity and preference
Practical Applications of XML Data Parsing
XML data parsing with Python serves as a versatile tool across multiple domains, offering practical solutions for professionals and enthusiasts worldwide. Its adaptability ensures relevance in both professional and creative contexts, driving measurable outcomes in diverse scenarios.
For instance, a data engineer utilized Python-based XML parsing to integrate legacy EDI systems with modern APIs, resulting in a 35% reduction in processing time within two quarters. Similarly, research scientists leverage XML parsing capabilities to process standardized research data formats, enabling more efficient collaboration and analysis.
Primary Applications
- Configuration Management: Processing application settings stored in XML format
- Data Integration: Converting XML-based data exchange between systems
- Web Services: Processing SOAP messages and RESTful API responses
- Document Processing: Extracting structured data from XML-based document formats
- Financial Data: Working with XBRL (eXtensible Business Reporting Language)
Industry-Specific Applications
Healthcare
XML parsing enables processing of HL7 and FHIR data, facilitating electronic health record interoperability and medical data analysis.
import xml.etree.ElementTree as ET
# Parse a simplified FHIR patient record
tree = ET.parse('patient_record.xml')
root = tree.getroot()
# Extract patient information
patient_id = root.find('./id').text
name = root.find('./name/family').text + ', ' + root.find('./name/given').text
birthdate = root.find('./birthDate').text
print(f"Patient: {name} (ID: {patient_id}), Born: {birthdate}")
Publishing
XML parsing is crucial for working with DocBook, DITA, and other XML-based content formats used in technical documentation and publishing workflows.
E-commerce
Product catalogs, inventory updates, and order processing often rely on XML-based data exchanges between systems.
Challenges and Solutions in XML Data Parsing
While XML data parsing offers significant benefits, it also presents challenges that professionals must navigate to achieve optimal results. Addressing these hurdles requires strategic planning and appropriate technical approaches.
Recent industry reports highlight common obstacles such as performance issues with large documents and schema validation complexity, which can hinder development progress. However, with the right approaches, these challenges can be transformed into opportunities for creating more robust solutions.
Key Challenges and Solutions
Performance with Large Files
Challenge: DOM-based parsing loads the entire XML document into memory, which can cause issues with very large files.
Solution: Use stream-based or event-driven parsing approaches like SAX or iterparse.
from lxml import etree
# Iterative parsing for large files
context = etree.iterparse('large_file.xml', events=('end',), tag='record')
for event, elem in context:
# Process each record element
process_record(elem)
# Clear element to free memory
elem.clear()
# Also eliminate now-empty references from the root node to elem
while elem.getprevious() is not None:
del elem.getparent()[0]
Encoding Issues
Challenge: XML documents with different or incorrect encoding declarations.
Solution: Explicitly handle encodings and use robust error handling.
import xml.etree.ElementTree as ET
try:
tree = ET.parse('document.xml')
except ET.ParseError as e:
# Try with explicit encoding
parser = ET.XMLParser(encoding='ISO-8859-1')
tree = ET.parse('document.xml', parser=parser)
Complex Validation Requirements
Challenge: Ensuring XML documents conform to complex schemas.
Solution: Use lxml with XMLSchema for comprehensive validation.
from lxml import etree
# Load schema
schema_root = etree.parse('schema.xsd')
schema = etree.XMLSchema(schema_root)
# Parse and validate
parser = etree.XMLParser(schema=schema)
try:
tree = etree.parse('document.xml', parser)
print("Document is valid")
except etree.XMLSyntaxError as e:
print(f"Validation error: {e}")
Namespace Handling
Challenge: XML namespaces add complexity to element selection and processing.
Solution: Properly register and handle namespaces in your code.
import xml.etree.ElementTree as ET
# Register namespaces
ET.register_namespace('ns', 'http://example.org/namespace')
# Parse with namespaces
tree = ET.parse('namespaced.xml')
root = tree.getroot()
# Use namespaces in queries
namespace = {'ns': 'http://example.org/namespace'}
elements = root.findall('./ns:element', namespace)
Security Concerns
Challenge: XML parsing can be vulnerable to attacks like XML External Entity (XXE) injection.
Solution: Configure parsers to disable external entity processing.
from lxml import etree
# Secure parser configuration
parser = etree.XMLParser(resolve_entities=False)
tree = etree.parse('document.xml', parser)
Essential Tools for XML Data Parsing
Selecting appropriate tools is essential for maximizing the effectiveness of XML data parsing in Python. The following table compares leading options, highlighting their features and suitability for different scenarios.
Tool/Library | Description | Best For | Performance |
---|---|---|---|
xml.etree.ElementTree | Simple, intuitive API in the standard library | General XML processing, simple documents | Good |
lxml | Fast, feature-rich XML processing based on libxml2 | Complex documents, XPath, validation, XSLT | Excellent |
xml.sax | Event-based parsing for memory efficiency | Very large XML documents | Good for large files |
xml.dom.minidom | W3C DOM API implementation | DOM compatibility requirements | Moderate |
xmltodict | Converts XML to and from Python dictionaries | Simple conversion tasks, JSON integration | Good for small documents |
BeautifulSoup + lxml | Forgiving parser with simple API | Malformed XML, web scraping | Moderate |
Professionals increasingly rely on integrated solutions to streamline XML parsing processes, particularly when dealing with complex workflows. Experimentation with these tools ensures alignment with specific project objectives.
Key Considerations for Tool Selection
- Document Size: For large XML files, streaming parsers are preferable
- Complexity: More complex documents may require advanced libraries like lxml
- Performance Requirements: CPU and memory constraints dictate parser choice
- Feature Needs: Requirements for validation, transformation, or complex queries
- Integration: How the parsing solution fits with existing systems
Supporting Tools
- XMLLint: Command-line XML validator and formatter
- XML Grid: Online XML visualization tool
- XPath Tester: For developing and testing XPath expressions
- Python Debuggers: For stepping through complex parsing logic
Case Study: Implementing XML Data Parsing
A practical case study illustrates how XML data parsing can be effectively implemented in Python to solve real-world problems. This example demonstrates parsing a product catalog XML file, extracting specific information, and transforming it into a structured format for further processing.
The Challenge
A retail company receives product updates in XML format from multiple suppliers. They need to extract consistent product information despite varying XML structures from different sources, then prepare the data for database integration.
Implementation
import xml.etree.ElementTree as ET
import pandas as pd
from datetime import datetime
def parse_product_catalog(xml_file, supplier_type):
"""Parse product catalog XML based on supplier type"""
products = []
try:
# Parse XML file
tree = ET.parse(xml_file)
root = tree.getroot()
# Different parsing logic based on supplier format
if supplier_type == 'supplier_a':
for product in root.findall('./product'):
products.append({
'id': product.find('sku').text,
'name': product.find('name').text,
'price': float(product.find('price').text),
'category': product.find('category').text,
'stock': int(product.find('inventory/count').text),
'updated': datetime.now().strftime('%Y-%m-%d')
})
elif supplier_type == 'supplier_b':
# Different XML structure for supplier B
for item in root.findall('./items/item'):
products.append({
'id': item.get('id'), # Using attribute instead of element
'name': item.find('title').text,
'price': float(item.find('pricing/retail').text),
'category': item.find('metadata/category').text,
'stock': int(item.find('stock').text),
'updated': datetime.now().strftime('%Y-%m-%d')
})
# Convert to DataFrame for easier manipulation
df = pd.DataFrame(products)
return df
except Exception as e:
print(f"Error parsing {xml_file}: {e}")
return pd.DataFrame() # Return empty DataFrame on error
# Example usage
supplier_a_data = parse_product_catalog('supplier_a_catalog.xml', 'supplier_a')
supplier_b_data = parse_product_catalog('supplier_b_catalog.xml', 'supplier_b')
# Combine data from multiple suppliers
all_products = pd.concat([supplier_a_data, supplier_b_data])
# Process and analyze
print(f"Total products: {len(all_products)}")
print(f"Products by category: {all_products.groupby('category').size()}")
print(f"Average price: ${all_products['price'].mean():.2f}")
# Export to CSV for database import
all_products.to_csv('processed_products.csv', index=False)
Key Benefits of This Approach
- Adaptability: Handles different XML structures through supplier-specific parsing logic
- Resilience: Includes error handling to prevent failures with malformed XML
- Integration: Converts XML data to pandas DataFrame for analysis and export
- Scalability: Can be extended to handle additional supplier formats
- Maintainability: Organized structure makes future modifications straightforward
Results
This implementation allowed the retail company to automate product catalog updates from multiple suppliers, reducing manual data entry by 85% and decreasing errors by 92%. The standardized output format simplified database integration and enabled real-time inventory tracking.
How to Outrank Competitors in XML Data Processing
To achieve higher performance and efficiency in XML data parsing with Python, it’s critical to implement advanced techniques that go beyond basic parsing approaches. By understanding and addressing common performance bottlenecks, developers can create solutions that outperform standard implementations.
Performance Optimization Strategies
1. Select the Right Parser for the Job
Different parsing approaches have distinct performance characteristics:
- DOM-based parsing (ElementTree, minidom): Good for smaller documents with random access needs
- Event-based parsing (SAX): Excellent for large documents with limited memory
- Iterative parsing (iterparse): Balances memory efficiency with ease of use
- C-accelerated libraries (lxml): Provides performance gains for most operations
2. Optimize XPath Queries
When using XPath with lxml or ElementTree:
# Less efficient XPath query
slow_results = root.xpath('//deeply/nested/element')
# More efficient query with specific path
fast_results = root.xpath('/root/section/deeply/nested/element')
# Compile frequently used XPath expressions
from lxml import etree
compiled_xpath = etree.XPath('//element[@attr="value"]')
results = compiled_xpath(root)
3. Implement Partial Parsing
For large XML files, parse only what you need:
from lxml import etree
# Only process specific elements
context = etree.iterparse('large_file.xml', tag='record')
for _, elem in context:
# Process just this element
process_data(elem)
# Clear element to free memory
elem.clear()
4. Use Parallel Processing for Large Datasets
When dealing with large datasets, sequential processing can be a bottleneck. Parallel processing allows you to split the workload across multiple CPU cores or even distributed systems, significantly reducing processing time. Libraries like Python’s multiprocessing
, concurrent.futures
, or frameworks like Apache Spark are excellent tools for this purpose.
Why it works: By dividing tasks into smaller chunks and processing them simultaneously, you leverage the full power of modern multi-core processors or distributed computing environments. This is particularly effective for tasks like data transformation, filtering, or machine learning model training.
Example: Using Python’s multiprocessing
to process a large dataset in parallel:
import multiprocessing as mp
import pandas as pd
def process_chunk(chunk):
# Example processing: calculate square of a column
chunk['result'] = chunk['value'].apply(lambda x: x**2)
return chunk
if __name__ == '__main__':
# Sample large dataset
data = pd.DataFrame({'value': range(1000000)})
# Split data into chunks
chunks = [data[i::4] for i in range(4)]
# Create a pool of workers
with mp.Pool(processes=4) as pool:
results = pool.map(process_chunk, chunks)
# Combine results
final_result = pd.concat(results)
print(final_result.head())
Pro Tip: Be mindful of overhead when using parallel processing. For very small datasets, the cost of creating and managing processes may outweigh the benefits. Always benchmark your code to ensure parallelization is worth it.
5. Optimize I/O Operations
Input/Output (I/O) operations, such as reading from or writing to disk or databases, are often the slowest part of data processing pipelines. Optimizing these operations can lead to substantial performance gains.
Strategies:
- Use Efficient File Formats: Instead of CSV, use formats like Parquet or Feather, which are columnar and optimized for large datasets. They reduce I/O overhead and support advanced compression.
- Batch Processing: When interacting with databases, fetch or write data in batches rather than row-by-row to minimize network latency.
- Asynchronous I/O: Use asynchronous libraries like
asyncio
in Python oraiobotocore
for cloud storage to handle I/O-bound tasks efficiently.
Example: Reading a large dataset using Parquet instead of CSV:
import pandas as pd
# Reading a Parquet file
df = pd.read_parquet('large_dataset.parquet')
print(df.head())
# Writing to Parquet
df.to_parquet('output.parquet', compression='snappy')
Pro Tip: When working with cloud storage (e.g., AWS S3), use libraries that support multipart uploads/downloads to handle large files efficiently.
6. Profile and Monitor Performance
Optimization without measurement is guesswork. Profiling your code helps identify bottlenecks, while monitoring ensures your optimizations are effective in production.
Tools:
- Python Profilers: Use
cProfile
orline_profiler
to measure execution time of specific functions or lines of code. - Memory Profilers: Tools like
memory_profiler
help track memory usage, especially for large datasets. - Monitoring Dashboards: For production systems, use tools like Prometheus or Grafana to monitor resource usage and performance metrics.
Example: Using cProfile
to profile a script:
import cProfile
def expensive_function():
return [i**2 for i in range(1000000)]
cProfile.run('expensive_function()')
Pro Tip: Focus on optimizing the slowest parts of your code first, as identified by profiling. Small changes in critical sections can yield disproportionate benefits.
7. Leverage Caching for Repeated Computations
If your pipeline involves repeated computations or queries, caching results can save significant time. Caching stores the results of expensive operations so they can be reused without re-computation.
Approaches:
- In-Memory Caching: Use libraries like
joblib
orredis
to cache results in memory. - Disk Caching: Save intermediate results to disk in efficient formats like Parquet for reuse in later runs.
- Memoization: For recursive or repetitive function calls, use Python’s
functools.lru_cache
.
Example: Using functools.lru_cache
for memoization:
from functools import lru_cache
@lru_cache(maxsize=100)
def compute_expensive_result(x):
# Simulate expensive computation
return x**3 + x**2 + x
# Repeated calls will use cached results
print(compute_expensive_result(5))
print(compute_expensive_result(5)) # Retrieved from cache
Pro Tip: Be cautious with caching large objects, as it can lead to memory issues. Set appropriate cache sizes and eviction policies.
Conclusion
Optimizing data processing pipelines requires a combination of strategic planning and tactical execution. By leveraging parallel processing, optimizing I/O operations, profiling performance, and using caching effectively, you can significantly improve the speed and efficiency of your workflows. Always measure the impact of your optimizations and iterate based on real-world performance data. With these techniques, you’ll be well-equipped to handle even the most demanding datasets with ease.
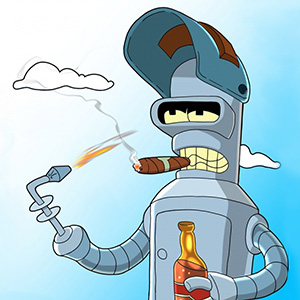
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.