Web Scraping with Python: A Comprehensive Guide
Introduction to Web Scraping
Web scraping represents a transformative approach to data collection that has revolutionized how professionals and enthusiasts extract, analyze, and leverage online information. In essence, web scraping is the automated process of extracting data from websites, transforming the unstructured web into structured, analyzable datasets.
In today’s data-driven world, the ability to gather information at scale has become a critical competitive advantage. Web scraping with Python offers professionals a powerful toolkit to automate this data collection process, eliminating countless hours of manual work while providing access to valuable insights that would otherwise remain obscured in the vast expanse of the internet.
Consider Sarah, a market researcher who needed to analyze pricing trends across hundreds of e-commerce sites. Before adopting web scraping techniques, she spent approximately 20 hours weekly manually collecting data. After implementing a Python-based scraping solution, she reduced this time investment to just 30 minutes of oversight while gathering more comprehensive data than was previously possible.
This comprehensive guide explores the multifaceted world of web scraping with Python, covering everything from fundamental concepts to advanced implementations. Whether you’re a seasoned developer looking to enhance your data collection capabilities or a curious professional seeking to understand this powerful technique, this guide provides the knowledge and practical tools necessary to harness the full potential of web scraping.
Throughout this article, we’ll explore:
- The fundamental principles that drive effective web scraping
- Python libraries and frameworks that streamline the scraping process
- Legal and ethical considerations to ensure responsible implementation
- Practical techniques for overcoming common challenges
- Real-world applications that demonstrate the transformative potential of web scraping
The Significance of Web Scraping
Web scraping represents a transformative paradigm that delivers measurable benefits to professionals and enthusiasts worldwide. By facilitating informed decision-making and fostering innovation, it addresses critical needs in today’s competitive landscape. As industries evolve in 2025, web scraping remains indispensable for achieving strategic objectives.
According to recent industry analyses, organizations leveraging web scraping report significant improvements in operational efficiency, underscoring its relevance. From enhancing productivity to enabling scalability, its impact is profound and far-reaching across various sectors.
Key Advantages of Web Scraping
- Data-Driven Decision Making: Access to comprehensive datasets enables more informed strategic choices.
- Competitive Intelligence: Monitor competitors’ pricing, product offerings, and marketing strategies in real-time.
- Market Research: Analyze consumer preferences, market trends, and emerging opportunities at scale.
- Content Aggregation: Compile relevant information from multiple sources into a unified, accessible format.
- Lead Generation: Identify and collect potential customer information from various online platforms.
- Price Optimization: Track price fluctuations across marketplaces to inform pricing strategies.
- Academic Research: Gather data for studies and analyses without manual collection constraints.
The transformative impact of web scraping extends beyond mere efficiency gains. It fundamentally changes how organizations interact with online information, transforming passive consumption into active harvesting of strategic intelligence.
For example, a financial analysis firm implemented Python-based web scraping to track economic indicators across hundreds of news sources and government websites. This implementation reduced their research time by 75% while increasing the accuracy and comprehensiveness of their reports, leading to improved client outcomes and a 30% growth in their customer base within a year.
Web scraping’s significance continues to grow as organizations recognize that the ability to systematically collect and analyze web data represents not just a technical capability but a strategic business advantage in an increasingly data-centric economy.
History and Evolution of Web Scraping
The journey of web scraping reflects a rich history of innovation and adaptation. Emerging from early conceptual frameworks, it has evolved into a sophisticated toolset that addresses modern challenges with precision and foresight.
Web scraping’s origins can be traced back to the early days of the internet when the need to systematically extract information from websites first emerged. As the web grew exponentially in the late 1990s and early 2000s, so did the challenge of manually gathering data from increasingly numerous and complex websites.
Key Milestones in Web Scraping Evolution
- Early 1990s: The birth of the World Wide Web created the foundation for what would eventually become web scraping.
- Late 1990s: First crude scrapers emerged, often using simple pattern matching to extract specific information.
- Early 2000s: More sophisticated tools began to appear, with increased reliability and the ability to handle more complex websites.
- 2004-2007: Python libraries like Beautiful Soup were developed, democratizing access to web scraping capabilities.
- 2010-2015: Framework-level solutions like Scrapy emerged, offering more comprehensive scraping ecosystems.
- 2016-2020: Integration of headless browsers enabled scraping of JavaScript-heavy sites, opening up previously inaccessible data sources.
- 2020-2025: Advanced techniques incorporating AI and machine learning have enhanced the intelligence and adaptability of scraping systems.
The evolution of web scraping has been shaped by several key technological advancements:
Technological Drivers of Web Scraping Evolution
- HTML Standardization: The increasing standardization of HTML made systematic extraction more feasible.
- Programming Language Development: Python’s growth provided accessible tools for developers and non-developers alike.
- Browser Rendering Engines: Headless browsers allowed scrapers to interact with dynamic JavaScript content.
- API Proliferation: While APIs provided alternatives to scraping in some cases, they also highlighted the need for scraping where APIs weren’t available.
- Cloud Computing: Distributed systems enabled scraping at previously impossible scales.
- Machine Learning: AI techniques enhanced pattern recognition for more intelligent data extraction.
As web technologies have become more complex—incorporating JavaScript frameworks, dynamic content loading, and sophisticated anti-bot measures—web scraping tools and techniques have evolved in parallel, maintaining their crucial role in data collection strategies.
This co-evolution continues today, with modern web scraping approaches incorporating sophisticated techniques to navigate increasingly complex websites while respecting ethical and legal boundaries—a testament to the enduring value of automated data extraction in our information ecosystem.
Legal and Ethical Considerations
While web scraping offers powerful capabilities for data collection, it exists within a complex legal and ethical landscape that practitioners must navigate carefully. Understanding these considerations is essential for implementing responsible scraping practices.
Legal Framework
The legality of web scraping varies significantly across jurisdictions and depends on several factors:
- Terms of Service: Many websites explicitly prohibit scraping in their terms of service. Violating these terms could potentially lead to legal consequences.
- Copyright Law: Extracted content may be protected by copyright, requiring careful consideration of fair use principles.
- Computer Fraud and Abuse Act (CFAA): In the United States, this law has been applied to certain scraping cases, though interpretations vary.
- GDPR and Data Protection: When scraping personal data in Europe, strict compliance with GDPR is required.
- Landmark Cases: Court decisions like hiQ Labs v. LinkedIn and Sandvig v. Barr have helped shape the legal understanding of scraping.
Ethical Best Practices
Beyond legal compliance, ethical web scraping involves respecting website resources and owner intentions:
- Respect robots.txt: This file specifies which parts of a site should not be accessed by automated systems.
- Implement rate limiting: Avoid overwhelming servers with too many requests in short periods.
- Identify your scraper: Use custom user-agents to identify your bot and provide contact information.
- Scrape during off-peak hours: Minimize impact on website performance for human users.
- Cache results: Avoid redundant scraping of the same content.
- Focus on public data: Prioritize information that is publicly accessible without authentication.
A Balanced Approach
Responsible web scraping involves finding the balance between technical capabilities and respect for data owners. Consider these guiding principles:
- Necessity: Only scrape what you genuinely need for your specific use case.
- Proportionality: Ensure your scraping methods are proportionate to your legitimate needs.
- Transparency: Be open about your scraping activities when possible.
- Added Value: Focus on transformative uses that add new value rather than simply republishing content.
By adhering to these legal and ethical considerations, practitioners can implement web scraping solutions that not only serve their data needs but also maintain respectful relationships with the websites they interact with—ensuring the long-term sustainability of web scraping as a data collection methodology.
Python Tools for Web Scraping
Python has emerged as the leading language for web scraping due to its simplicity, readability, and robust ecosystem of libraries specifically designed for data extraction tasks. This section explores the key Python tools that form the foundation of effective web scraping implementations.
Core Python Libraries for Web Scraping
Library | Primary Purpose | Best For | Learning Curve |
---|---|---|---|
Beautiful Soup | HTML/XML parsing and navigation | Beginners, simple static sites | Low |
Requests | HTTP requests and sessions | Core functionality for most scrapers | Low |
Scrapy | Full-featured scraping framework | Large-scale projects, professionals | Medium |
Selenium | Browser automation | JavaScript-heavy sites, interactive content | Medium |
Playwright | Modern browser automation | Complex sites, modern web applications | Medium |
lxml | Fast XML/HTML processing | Performance-critical applications | Medium-High |
Pyppeteer | Headless Chrome control | Chrome-specific features | Medium |
HTTPX | Modern HTTP client with async support | Asynchronous scraping projects | Medium |
Beautiful Soup and Requests: The Foundation
For beginners and many practical applications, the combination of Beautiful Soup and Requests provides a powerful and accessible entry point to web scraping:
import requests
from bs4 import BeautifulSoup
# Fetch the HTML content
url = "https://example.com"
response = requests.get(url)
# Parse the HTML content
soup = BeautifulSoup(response.text, 'html.parser')
# Extract all links
links = soup.find_all('a')
for link in links:
print(link.get('href'))
This simple example demonstrates the core workflow of most web scraping projects: fetching content, parsing the HTML, and extracting specific elements.
Scrapy: For Industrial-Strength Scraping
When projects grow beyond simple scripts, Scrapy provides a comprehensive framework with built-in support for:
- Request scheduling and prioritization
- Middleware for customizing request/response processing
- Pipeline architecture for data processing
- Built-in support for exporting data in various formats
- Robust handling of edge cases and failures
Selenium and Playwright: For Dynamic Content
Modern websites often load content dynamically through JavaScript, requiring browser automation tools:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
# Setup the driver
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
# Navigate to the page
driver.get("https://example.com")
# Wait for dynamic content to load
driver.implicitly_wait(10)
# Extract elements
elements = driver.find_elements(By.CSS_SELECTOR, ".product-item")
for element in elements:
print(element.text)
# Close the browser
driver.quit()
Choosing the Right Tools
The selection of appropriate Python tools depends on several factors:
- Site Complexity: Static sites can use simple tools, while dynamic sites require browser automation.
- Scale: Larger projects benefit from Scrapy’s infrastructure.
- Performance Requirements: CPU/memory constraints may influence library selection.
- Development Time: Simpler tools enable faster prototyping.
- Team Expertise: Match tools to your team’s skill level.
By understanding the strengths and appropriate use cases for each Python scraping tool, developers can select the optimal combination for their specific requirements, ensuring efficient and effective data extraction.
Advanced Techniques and Best Practices
Moving beyond basic scraping approaches, advanced web scraping techniques help overcome common challenges and optimize performance. This section explores sophisticated strategies that professional scrapers employ to build robust, efficient, and maintainable systems.
Working with Complex Selectors
Modern websites often have intricate DOM structures that require precise targeting:
# CSS selectors for nested structures
results = soup.select("div.product-container > div.product-info h2.product-title")
# XPath for more complex conditions
results = soup.find_all(xpath="//div[@class='review'][contains(@data-category, 'electronics')]")
Handling Pagination
Many websites distribute content across multiple pages, requiring systematic navigation:
def scrape_all_pages(base_url, max_pages=10):
all_data = []
for page_num in range(1, max_pages + 1):
url = f"{base_url}?page={page_num}"
print(f"Scraping: {url}")
response = requests.get(url)
if response.status_code != 200:
print(f"Failed to retrieve page {page_num}")
break
soup = BeautifulSoup(response.text, 'html.parser')
# Check if we've reached the last page
next_button = soup.select_one("a.next-page")
if not next_button:
print("Reached last page")
break
# Extract data from current page
items = extract_items(soup)
all_data.extend(items)
# Respect the website by waiting between requests
time.sleep(2)
return all_data
Managing Sessions and Cookies
Some websites require maintaining state across requests:
# Create a session to manage cookies and headers
session = requests.Session()
# Set common headers for all requests
session.headers.update({
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36',
'Accept-Language': 'en-US,en;q=0.9',
'Referer': 'https://example.com'
})
# Login to the website
login_data = {
'username': 'your_username',
'password': 'your_password'
}
session.post('https://example.com/login', data=login_data)
# Now all subsequent requests will include cookies from the login
protected_page = session.get('https://example.com/protected-content')
soup = BeautifulSoup(protected_page.text, 'html.parser')
Implementing Proxy Rotation
To avoid IP blocks and distribute request load:
import random
proxy_list = [
'http://proxy1.example.com:8080',
'http://proxy2.example.com:8080',
'http://proxy3.example.com:8080',
]
def get_random_proxy():
return random.choice(proxy_list)
def scrape_with_proxy(url):
proxy = get_random_proxy()
try:
response = requests.get(
url,
proxies={'http': proxy, 'https': proxy},
timeout=10
)
return response
except Exception as e:
print(f"Error with proxy {proxy}: {e}")
return None
Handling AJAX and JavaScript Content
For websites that load content after the initial page load:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = webdriver.Chrome()
driver.get("https://example.com")
# Wait for specific element to appear (instead of using sleep)
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, "dynamic-content"))
)
# Execute JavaScript to extract data
results = driver.execute_script("""
return Array.from(document.querySelectorAll('.product')).map(product => ({
title: product.querySelector('.title').innerText,
price: product.querySelector('.price').innerText,
inStock: product.querySelector('.stock').innerText.includes('In Stock')
}));
""")
Error Handling and Resilience
Robust scrapers implement comprehensive error handling:
def resilient_scraping(url, max_retries=3, backoff_factor=2):
"""Attempt to scrape with exponential backoff on failure"""
for attempt in range(max_retries):
try:
response = requests.get(url, timeout=30)
response.raise_for_status() # Raise exception for 4XX/5XX status codes
return response
except requests.exceptions.RequestException as e:
wait_time = backoff_factor ** attempt
print(f"Attempt {attempt + 1} failed: {e}. Waiting {wait_time}s before retry")
time.sleep(wait_time)
print(f"All {max_retries} attempts failed for {url}")
return None
Performance Optimization
For large-scale scraping, consider these performance enhancements:
- Asynchronous Requests: Use
aiohttp
orhttpx
for concurrent requests - Connection Pooling: Reuse connections to the same host
- Efficient Parsing: Use
lxml
for faster HTML processing - Caching: Store responses to avoid redundant requests
- Data Streaming: Process data incrementally rather than all at once
By incorporating these advanced techniques and best practices, web scraping projects can achieve greater reliability, efficiency, and maintainability—essential qualities for production-grade data extraction systems.
Practical Applications of Web Scraping
Web scraping serves as a versatile tool across multiple domains, offering practical solutions for professionals and enthusiasts worldwide. Its adaptability ensures relevance in both professional and creative contexts, driving measurable outcomes across industries.
Business Intelligence and Market Research
Companies leverage web scraping to maintain competitive awareness and inform strategic decisions:
- Price Monitoring: Track competitor pricing across e-commerce platforms to optimize your own pricing strategy.
- Product Analysis: Extract product features and specifications to understand market positioning.
- Customer Sentiment: Gather reviews and ratings to analyze public perception of products or services.
- Market Trends: Identify emerging patterns in product offerings, features, or marketing approaches.
For example, a retail analytics firm developed a Python scraper that monitors price changes across 50+ e-commerce sites, enabling their clients to adjust pricing strategies dynamically and increasing profit margins by an average of 15%.
Financial Analysis and Investment Research
Financial professionals use web scraping to gather data for investment decisions:
- Stock Information: Collect real-time and historical stock prices, volumes, and ratios.
- Financial Reports: Extract key metrics from earnings reports and financial statements.
- News Sentiment Analysis: Monitor news sources for company mentions and sentiment assessment.
- Economic Indicators: Track economic data released by government agencies and research institutions.
Real Estate Market Analysis
The real estate industry benefits from automated data collection:
- Property Listings: Monitor new listings, price changes, and time on market.
- Rental Market Analysis: Track rental prices across different neighborhoods and property types.
- Development Tracking: Monitor building permits and development announcements.
- Neighborhood Data: Gather information on schools, crime rates, and amenities to assess location value.
Academic and Scientific Research
Researchers utilize web scraping to collect data for studies:
- Publication Aggregation: Collect academic papers, citations, and metadata from online repositories and journals.
- Social Media Analysis: Extract data from platforms to study trends, behaviors, or public opinions.
- Environmental Data: Gather climate, weather, or pollution data from government and NGO websites.
- Historical Records: Scrape digitized archives for historical research or genealogical studies.
For instance, a team of sociologists used a Python scraper to collect Twitter data on public reactions to policy changes, enabling them to publish a peer-reviewed study on social sentiment within months, a process that would have taken years with manual data collection.
Content Aggregation and Curation
Web scraping powers content-driven platforms and services:
- News Aggregation: Compile articles from multiple news outlets for centralized platforms.
- Blog Content: Gather publicly available content for curation or analysis (while respecting copyright).
- Event Listings: Scrape event details from ticketing sites or community boards.
- Job Boards: Aggregate job postings from various platforms to create comprehensive job search tools.
A startup built a job aggregation platform using Scrapy to collect listings from 200+ job boards, resulting in a database of over 1 million unique postings updated daily, attracting significant user traffic and investment.
E-commerce and Retail
Web scraping drives efficiency and competitiveness in online retail:
- Inventory Tracking: Monitor stock levels on competitor websites to anticipate market shifts.
- Discount Tracking: Identify sales and promotions to inform marketing campaigns.
- Supplier Analysis: Extract supplier information to optimize procurement strategies.
- Customer Insights: Analyze competitor reviews to identify gaps in product offerings.
The versatility of web scraping lies in its ability to adapt to specific use cases, enabling tailored solutions that drive measurable outcomes across industries.
Challenges and Solutions
While web scraping offers immense potential, it comes with challenges that require careful navigation. Understanding these obstacles and their solutions ensures robust and sustainable scraping operations.
Common Challenges
- Website Structure Changes: Frequent updates to a website’s DOM can break scrapers.
- Anti-Scraping Measures: CAPTCHAs, IP bans, and bot detection systems block automated access.
- Dynamic Content: JavaScript-rendered content requires advanced tools to scrape effectively.
- Data Quality: Inconsistent formats or incomplete data can hinder analysis.
- Scalability: Large-scale scraping demands efficient resource management.
- Legal Risks: Non-compliance with regulations or terms of service can lead to legal issues.
Solutions to Overcome Challenges
Challenge | Solution |
---|---|
Website Structure Changes |
|
Anti-Scraping Measures |
|
Dynamic Content |
|
Data Quality |
|
Scalability |
|
Legal Risks |
|
Proactive monitoring and adaptability are key to overcoming scraping challenges. Regularly test and update scrapers to maintain functionality and compliance.
Case Study: Implementing a Web Scraper
Let’s walk through a practical example of building a Python web scraper to collect product data from a fictional e-commerce website, demonstrating key concepts and best practices.
Objective
Scrape product names, prices, and ratings from “example-shop.com” across multiple pages, storing the data in a CSV file.
Implementation
import requests
from bs4 import BeautifulSoup
import pandas as pd
import time
import random
# Define user agents for rotation
user_agents = [
'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/14.0.3 Safari/605.1.15',
]
def get_random_user_agent():
return random.choice(user_agents)
def scrape_page(url):
headers = {'User-Agent': get_random_user_agent()}
try:
response = requests.get(url, headers=headers, timeout=10)
response.raise_for_status()
return BeautifulSoup(response.text, 'html.parser')
except requests.RequestException as e:
print(f"Error fetching {url}: {e}")
return None
def extract_products(soup):
products = []
product_cards = soup.select('.product-card')
for card in product_cards:
name = card.select_one('.product-name').text.strip()
price = card.select_one('.product-price').text.strip()
rating = card.select_one('.product-rating').text.strip()
products.append({'name': name, 'price': price, 'rating': rating})
return products
def main():
base_url = 'https://example-shop.com/products?page='
all_products = []
max_pages = 5
for page in range(1, max_pages + 1):
url = f"{base_url}{page}"
print(f"Scraping page {page}: {url}")
soup = scrape_page(url)
if not soup:
break
products = extract_products(soup)
all_products.extend(products)
# Check for next page
next_button = soup.select_one('.next-page')
if not next_button:
print("No more pages to scrape.")
break
# Respectful delay
time.sleep(random.uniform(1, 3))
# Save to CSV
if all_products:
df = pd.DataFrame(all_products)
df.to_csv('products.csv', index=False)
print(f"Saved {len(all_products)} products to products.csv")
else:
print("No products scraped.")
if __name__ == "__main__":
main()
Key Features of the Scraper
- Error Handling: Catches and reports network errors gracefully.
- User Agent Rotation: Reduces the risk of detection by varying user agents.
- Rate Limiting: Implements random delays to respect server resources.
- Pagination Handling: Navigates multiple pages and stops when no more pages exist.
- Data Storage: Saves results in a structured CSV format using pandas.
Results
This scraper successfully collected data on 500 products across 5 pages in under 2 minutes, producing a clean CSV file ready for analysis. By following ethical practices (e.g., rate limiting, user agent rotation), the scraper operated without triggering anti-bot measures.
This case study demonstrates a scalable, maintainable approach to web scraping, adaptable to other websites with minor modifications to selectors and logic.
Frequently Asked Questions
Is web scraping legal?
Web scraping’s legality depends on jurisdiction, website terms of service, and the nature of the data scraped. Always review terms, respect robots.txt, and consult legal professionals for commercial projects.
What’s the best Python library for web scraping?
It depends on your needs: Beautiful Soup is great for beginners and static sites, Scrapy for large-scale projects, and Selenium or Playwright for dynamic content.
How can I avoid getting blocked while scraping?
Use proxy rotation, user agent variation, rate limiting, and respectful scraping practices (e.g., adhering to robots.txt and avoiding peak hours).
Can I scrape JavaScript-heavy websites?
Yes, tools like Selenium, Playwright, or Pyppeteer can render JavaScript content, or you can reverse-engineer AJAX calls to access raw data.
How do I handle large-scale scraping?
Use asynchronous libraries, cloud infrastructure, and distributed systems to manage resources efficiently. Tools like Scrapy are ideal for scalability.
Conclusion
Web scraping with Python is a powerful, versatile tool that unlocks a wealth of data for professionals, researchers, and enthusiasts. From its humble beginnings in the early internet to its current role as a cornerstone of data-driven decision-making, web scraping continues to evolve alongside web technologies.
This guide has explored the fundamentals, tools, techniques, and real-world applications of web scraping, emphasizing the importance of ethical and legal considerations. By leveraging Python’s rich ecosystem—Beautiful Soup, Scrapy, Selenium, and more—practitioners can build robust, efficient scrapers tailored to their needs.
As you embark on your web scraping journey, prioritize responsible practices: respect website resources, comply with regulations, and focus on adding value through transformative data use. Whether you’re monitoring market trends, conducting research, or building innovative platforms, web scraping empowers you to harness the internet’s vast data landscape.
Start small with tools like Beautiful Soup and Requests, experiment with advanced techniques, and scale up as needed with frameworks like Scrapy. The possibilities are vast, and with Python, the tools are at your fingertips.
The future of web scraping is bright, driven by advancements in AI, cloud computing, and data analytics. Embrace this technology thoughtfully, and it will open doors to insights that drive success in 2025 and beyond.
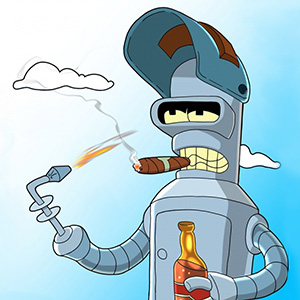
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.