Weather Data Parsing
The world is real-data-driven in our modern age. Accurate, timely and weather information is necessary for various businesses and applications. To illustrate, agriculture and aviation industry, which rely on the data in managing their crop and scheduling, will benefit just as outdoor events and the natural disaster situation where weather data analysis will make a real difference. This post address the domain of weather parsing cruising through the importance, ways, and techniques.
Weather Parsing: A Secret Code Unlocking Human Cognition.
Science of weather forecasting is based on skillful forecast parsing, which stands for basis of responsible decision making processes, that depend upon meteorological data. The essence of obtaining information from different sources, including weather report, forecast model, and sensor readings, is the implication that the organization can make more effective and well-informed decisions based on the value of insights gathered.Effective weather parsing enables:
-
Targeted Forecasting: With the help of weather forecasting, it enables precise weather forecast for precise location and timeframe which can be utilized for good and step up planning as well as risk management.
-
Data Integration: Making weather data readily available to extract weather-related information. This can be done by seamlessly incorporating the data into existing systems and processes allowing real-time monitoring and analysis.
-
Trend Analysis: Discovering keeping-long-term pathways of weather and trends which can assist with strategic planning and facility equipment management.
Techniques for Studying the Atmosphere
Weather parsing that is truthful and comprehensive is solely successful through the utilization of complex algorithm and the best practices. Here are some key approaches:Here are some key approaches:
NLP algorithms are playing a key role in explaining weather information when textual data sources are read, such as reports and forecasts. These features need to be capable of retrieving right data points, tagging named entities (place, date etc.) and information regarding the context.
Machine learning and deep learning models are capable of being trained on the large databases of the weather statistics which allows to recognize patterns of the weather and make predictions of higher, due to their superior accuracy, accuracy. Such systems can evolve to suit the pretentions of the ever- changing areas and get better with time.
Unifying weather data in a consistent set of formats and incorporating data from an array of sources forms a vital part of the weather data parsing. It is achieved through normalization, cleaning and merging of data which now creates accurate and consistent weather information.
Geospatial Analysis
Gathering weather data is not a straightforward procedure since data related to locations are needed. Using geospatial analysis techniques, for example, GIS and spatial data processing, the weather data can be paired with locations, so the correlation of the statistical data with the locations can be established.
Weather Related Parsers Behavior
To ensure optimal results and minimize errors, it is essential to follow industry best practices when implementing weather parsing solutions:To ensure optimal results and minimize errors, it is essential to follow industry best practices when implementing weather parsing solutions:
-
Data Quality: The bar to be on weather data collection sources should be set high to reduce the chance of having erroneous or unfinished data that can give wrong parsing results.
-
Continuous Monitoring: Put in detection and validation processes that are continuous so that the machine can detect and handle any errors or inconsistencies encountered in the weather data as it is parsed.
-
Scalability and Performance: Develop advanced weather data parsing systems that will not only handle high influxes of data but, as the system grows, will not drift away from being performance-driven and top quality parsing.
-
Domain Expertise: Partner with meteorologists and acknowledge expert advice to make sure that the parsing techniques align well with the common standards and super practices in the domain.
-
Regular Updates: The weather parsing algorithms and models of the platform must be upgraded and improved continuously so that the platform can match the progress of the global data sources.
Conclusion
The parsing of weather data is highly related to the varying landscape of weather information. It is particularly important for an extensive set of industries and applications since they are heavily relying on such data. Adoption of NLP by the organizations as well as machine learning, standardization of data and geospatial analysis is the best way to possess all the weather data. By complying with the best techniques and improving perseveration methodologies we may provide accurate and useful outcomes so that informed decisions could be approved and risk management could be guaranteed.
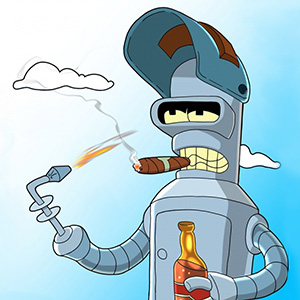
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.