Undetected Chromedriver in Python: Advanced Web Scraping Techniques
Introduction to Undetected Chromedriver
Undetected Chromedriver has emerged as an essential tool in the Python developer’s arsenal, particularly for those involved in web scraping, test automation, and data collection. In today’s digital landscape, websites employ increasingly sophisticated anti-bot mechanisms to detect and block automated browsers, making traditional web scraping approaches increasingly difficult.
Developed by the GitHub user “ultrafunkamsterdam,” undetected chromedriver provides a solution to this challenge by modifying the standard Selenium ChromeDriver to bypass modern bot detection systems. This approach enables developers to interact with websites programmatically while mimicking genuine human browsing patterns, significantly reducing the likelihood of being blocked.
Consider Sarah, a data analyst who needed to collect market research from e-commerce websites. Using standard automation tools, she encountered constant CAPTCHAs and IP blocks that made her project nearly impossible. After implementing undetected chromedriver, she could reliably gather the data she needed without triggering detection mechanisms, completing her project efficiently and accurately.
As websites continue to enhance their security measures, tools like undetected chromedriver have become indispensable for professionals who rely on web automation. Whether you’re conducting market research, testing applications, or gathering data for analysis, understanding how to effectively use undetected chromedriver can dramatically improve your success rates and productivity.
This comprehensive guide will delve into the multifaceted aspects of undetected chromedriver, covering its historical development, practical applications, implementation strategies, and best practices. We’ll examine common challenges and their solutions, compare alternative approaches, and provide actionable insights to help you maximize the effectiveness of this powerful tool in your Python projects.
Why Undetected Chromedriver Matters
Undetected chromedriver represents a significant advancement in web automation technology, addressing critical challenges that have long plagued developers and data professionals. Its importance extends beyond simple convenience, offering substantive benefits that directly impact project outcomes and capabilities.
Modern websites employ multiple layers of protection against automated access, including browser fingerprinting, behavior analysis, and JavaScript challenges. These mechanisms can identify and block traditional automation tools within seconds, rendering them ineffective for sustained interaction. Undetected chromedriver circumvents these obstacles by implementing several key modifications to the standard ChromeDriver implementation.
Key Benefits of Undetected Chromedriver
- Reduced Detection Rates: Modifies ChromeDriver signatures and behaviors that typically trigger anti-bot systems
- Persistent Sessions: Maintains browser state across sessions, simulating more natural user behavior
- Automatic Webdriver Patches: Self-updates to address new detection techniques
- Simplified Implementation: Requires minimal configuration changes from standard Selenium usage
- Compatibility: Works with existing Selenium-based code with minor modifications
According to web scraping professionals, projects using undetected chromedriver report success rates 30-50% higher than those using standard automation approaches. This translates directly to more reliable data collection, reduced maintenance overhead, and faster project completion times.
Note: While undetected chromedriver significantly improves automation reliability, it should be used responsibly and ethically. Always respect website terms of service, implement proper rate limiting, and consider the impact of your scraping activities.
The strategic value of undetected chromedriver becomes particularly evident in scenarios where consistent, uninterrupted access to web resources is critical. Research institutions, data analysis firms, and software testing teams increasingly rely on this technology to maintain operational efficiency in environments where traditional automation would fail.
History and Evolution of Undetected Chromedriver
The development of undetected chromedriver represents a fascinating chapter in the ongoing technological cat-and-mouse game between web automation tools and anti-bot systems. Understanding this evolution provides valuable context for appreciating its current capabilities and anticipating future developments.
Origins and Early Development
The story begins around 2019 when websites began implementing more sophisticated detection mechanisms that could identify the presence of automation frameworks like Selenium. These systems could detect telltale signatures of WebDriver, including specific JavaScript variables, timing patterns, and browser attributes that differed from regular user sessions.
In response to these challenges, GitHub user “ultrafunkamsterdam” released the first version of undetected-chromedriver, designed specifically to bypass these detection mechanisms. The initial release focused on removing the most obvious indicators of automation, such as the navigator.webdriver flag that Selenium adds to the browser environment.
Key Evolutionary Milestones
- 2019: Initial release focusing on basic WebDriver signature removal
- 2020: Added support for Chrome DevTools Protocol (CDP) for advanced browser manipulation
- 2021: Implemented automated patching systems to adapt to new Chrome versions
- 2022: Enhanced proxy support and user profile persistence
- 2023-2024: Integration with additional tools and frameworks, improved stability across platforms
With each iteration, undetected chromedriver has evolved to address new detection techniques. What began as a relatively simple patch has grown into a comprehensive solution that modifies numerous browser attributes, behaviors, and signatures to create a browsing environment virtually indistinguishable from manual use.
The project has benefited significantly from community contributions, with developers worldwide sharing detection bypass techniques and implementation improvements. This collaborative approach has enabled the tool to remain effective despite the rapid evolution of anti-bot systems.
Today, undetected chromedriver stands as one of the most widely used tools for reliable web automation, with thousands of stars on GitHub and an active community of contributors continuing to refine and enhance its capabilities.
How Undetected Chromedriver Works
Understanding the technical mechanisms behind undetected chromedriver provides valuable insights into its effectiveness and helps developers implement it optimally. At its core, this tool employs several sophisticated techniques to evade detection while maintaining compatibility with the Selenium framework.
Core Technology and Detection Evasion
Undetected chromedriver modifies the standard ChromeDriver in several key ways:
- Cloaking WebDriver Properties: Removes or modifies the telltale navigator.webdriver property that websites use to detect automation
- Randomized User-Agent Strings: Generates plausible, current browser identifiers that match the Chrome version being used
- Custom Chrome Launch Parameters: Adds specific flags to Chrome that disable automation indicators
- WebDriver Protocol Modifications: Alters how WebDriver commands are transmitted to avoid detection patterns
- Stealth Extensions: Implements browser plugins that help normalize browser behavior
These modifications work in concert to create a browsing environment that closely mimics genuine human interaction. The tool continually adapts to new Chrome versions, automatically downloading appropriate driver binaries and applying the necessary patches.
Technical Architecture
# Simplified overview of undetected_chromedriver architecture
ChromeOptions
↓
Modified Launch Parameters
↓
Browser Profile Management
↓
WebDriver Protocol Layer
↓
Patching System
↓
Automation Client
One of the key architectural advantages is how undetected chromedriver integrates with the standard Selenium API. It subclasses the original ChromeDriver, overriding only the methods necessary for evasion while preserving full compatibility with existing Selenium code.
Technical Note: Undetected chromedriver works at multiple levels of the browser automation stack, from browser launch parameters to runtime JavaScript execution. This comprehensive approach is why it remains effective against sophisticated detection systems.
The tool also implements mechanisms to handle session persistence, allowing developers to save and restore browser states across automation runs. This feature further enhances the naturalness of the browsing patterns by maintaining cookies, local storage, and other session data that would normally persist in human browsing scenarios.
Installation and Setup
Getting started with undetected chromedriver is straightforward, requiring just a few steps to integrate it into your Python environment. This section provides a comprehensive guide to installing and configuring the tool for optimal performance.
Basic Installation
The most common approach is to install undetected chromedriver using pip, Python’s package manager:
# Install using pip
pip install undetected-chromedriver
For the latest development version with the most recent features and fixes, you can install directly from the GitHub repository:
# Install latest development version
pip install git+https://github.com/ultrafunkamsterdam/undetected-chromedriver.git
Prerequisites
Before using undetected chromedriver, ensure you have the following dependencies:
- Python 3.6 or higher
- Google Chrome browser installed
- Selenium package (usually installed automatically as a dependency)
Unlike standard Selenium setups, you typically don’t need to download the ChromeDriver binary separately, as undetected chromedriver handles this automatically, downloading the appropriate version for your installed Chrome browser.
Configuration Options
When initializing undetected chromedriver, you can customize various aspects of its behavior:
import undetected_chromedriver as uc
import time
# Basic configuration
options = uc.ChromeOptions()
# Optional: Use headless mode (less detectable than standard headless)
# options.add_argument('--headless')
# Optional: Set user data directory for session persistence
# options.add_argument('--user-data-dir=./chrome_profile')
# Initialize the driver with options
driver = uc.Chrome(options=options)
# Test with a site that typically detects automation
driver.get('https://bot.sannysoft.com')
time.sleep(5)
driver.save_screenshot('test_result.png')
driver.quit()
Important: While undetected chromedriver supports headless mode, be aware that headless browsers have other fingerprinting characteristics that might trigger detection on sophisticated websites. For maximum undetectability, use non-headless mode when possible.
Verifying Installation
To confirm your setup is working correctly, try accessing a website that typically blocks automated browsers, such as bot detection test sites. If the page loads normally without being redirected to CAPTCHA or blockage pages, your installation is likely functioning as expected.
Basic Usage Guide
Once you’ve successfully installed undetected chromedriver, implementing it in your Python projects is remarkably similar to working with standard Selenium. This section covers the fundamental patterns and best practices for everyday usage scenarios.
Simple Automation Example
Here’s a basic script demonstrating how to use undetected chromedriver for web automation:
import undetected_chromedriver as uc
import time
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Initialize the driver
driver = uc.Chrome()
driver.get('https://example.com')
# Wait for elements to be available (explicit wait)
wait = WebDriverWait(driver, 10)
element = wait.until(EC.presence_of_element_located((By.ID, 'some-element-id')))
# Interact with the page
element.click()
search_box = driver.find_element(By.NAME, 'q')
search_box.send_keys('undetected chromedriver')
search_box.submit()
# Allow time for the search to complete
time.sleep(2)
# Extract data
results = driver.find_elements(By.CSS_SELECTOR, '.result-item')
for result in results[:5]:
print(result.text)
# Clean up
driver.quit()
Key Usage Patterns
The following patterns will help you get the most out of undetected chromedriver:
- Use Explicit Waits: Rather than fixed time.sleep() calls, use WebDriverWait for more reliable automation
- Session Persistence: Reuse user profiles to maintain login states and cookies
- Random Delays: Implement random timing between actions to further mimic human behavior
- Error Handling: Implement robust try/except blocks to manage potential issues
Working with User Profiles
One of the most powerful features of undetected chromedriver is the ability to persist browser state across sessions:
import undetected_chromedriver as uc
import os
# Define user data directory
user_data_dir = os.path.join(os.getcwd(), 'chrome_user_data')
# Configure options
options = uc.ChromeOptions()
options.add_argument(f'--user-data-dir={user_data_dir}')
# Initialize driver with the profile
driver = uc.Chrome(options=options)
# The browser will now use the saved state (cookies, localStorage, etc.)
driver.get('https://example.com')
Tip: Using persistent profiles is especially valuable for maintaining logged-in states on websites that require authentication, reducing the need to repeatedly log in during different automation sessions.
Working with Proxies
To further enhance anonymity or distribute requests across multiple IP addresses:
import undetected_chromedriver as uc
options = uc.ChromeOptions()
options.add_argument('--proxy-server=http://proxy-ip:port')
driver = uc.Chrome(options=options)
driver.get('https://httpbin.org/ip') # Check your IP
These basic usage patterns provide a foundation for building more complex automation workflows while maintaining a high level of undetectability.
Advanced Techniques
Once you’re comfortable with the basics of undetected chromedriver, exploring advanced techniques can significantly enhance your web automation capabilities. This section covers sophisticated strategies that experienced developers employ to maximize effectiveness and reliability.
JavaScript Execution for Enhanced Interactions
Executing JavaScript directly in the browser context can provide more natural interactions that are harder to detect as automated:
# Using JavaScript to scroll naturally
driver.execute_script("""
const scrollHeight = document.body.scrollHeight;
const totalSteps = 10;
let currentStep = 0;
function smoothScroll() {
if (currentStep >= totalSteps) return;
const scrollPosition = (scrollHeight / totalSteps) * currentStep;
window.scrollTo(0, scrollPosition);
currentStep++;
setTimeout(smoothScroll, 100 + Math.random() * 200);
}
smoothScroll();
""")
# Using JavaScript to interact with elements more naturally
element = driver.find_element(By.ID, 'target-button')
driver.execute_script("arguments[0].click();", element)
Managing Browser Fingerprinting
Browser fingerprinting is a sophisticated technique used to identify users based on browser characteristics. To further reduce detectability:
options = uc.ChromeOptions()
# Adjust timezone to match proxy location (if using proxies)
options.add_argument('--timezone="America/New_York"')
# Match language settings to intended region
options.add_argument('--lang=en-US')
# Set specific window dimensions to avoid unusual sizes
options.add_argument('--window-size=1920,1080')
driver = uc.Chrome(options=options)
Using CDP (Chrome DevTools Protocol)
CDP provides powerful capabilities for manipulating the browser at a low level:
# Set geolocation using CDP
driver.execute_cdp_cmd("Emulation.setGeolocationOverride", {
"latitude": 40.7128,
"longitude": -74.0060,
"accuracy": 100
})
# Modify navigator properties using CDP
driver.execute_cdp_cmd("Page.addScriptToEvaluateOnNewDocument", {
"source": """
Object.defineProperty(navigator, 'hardwareConcurrency', {
get: () => 8
});
"""
})
Handling Sophisticated CAPTCHAs
Even with undetected chromedriver, you may occasionally encounter CAPTCHAs. Advanced strategies include:
- CAPTCHA Solving Services: Integration with services like 2Captcha or Anti-Captcha
- Audio CAPTCHA Solutions: Using speech recognition for audio CAPTCHA challenges
- Human-in-the-loop: Pausing automation for manual CAPTCHA solving when necessary
# Pseudocode for CAPTCHA handling
def check_for_captcha():
try:
captcha_element = driver.find_element(By.ID, 'captcha-container')
if captcha_element.is_displayed():
# Option 1: Use a solving service
captcha_solution = solve_with_service(captcha_element)
# Option 2: Notify human operator
notify_operator_and_wait()
except NoSuchElementException:
# No CAPTCHA found, continue
pass
Distributed Scraping Architecture
For large-scale projects, distributing requests across multiple instances of undetected chromedriver can improve reliability and throughput:
This approach typically involves:
- Multiple worker instances with different browser profiles
- Rotation of proxies, user-agents, and other fingerprinting attributes
- Centralized task queue and result storage
- Sophisticated rate limiting and retry logic
These advanced techniques, when implemented correctly, can dramatically improve the effectiveness of your automation projects while maintaining a high level of undetectability.
Challenges and Solutions
Despite its effectiveness, working with undetected chromedriver presents certain challenges that developers should anticipate and address. This section outlines common obstacles and proven solutions to overcome them.
Common Challenges
Challenge | Description | Solution Approach |
---|---|---|
Version Compatibility | Chrome updates can temporarily break functionality | Pin Chrome versions in production environments |
Headless Limitations | Headless mode has additional detection vectors | Use non-headless mode with virtual displays |
Resource Consumption | Chrome instances can be memory-intensive | Implement proper resource management and cleanup |
Advanced Bot Detection | Some sites use behavioral analysis | Implement human-like interaction patterns |
IP-Based Blocking | Repeated access from same IP gets flagged | Utilize rotating proxies and session management |
Version Management Strategies
Chrome updates frequently, which can sometimes introduce compatibility issues with undetected chromedriver. To manage this:
- Version Pinning: In production environments, pin Chrome to a specific version
- Testing Pipeline: Implement automated testing for new Chrome versions
- Fallback Mechanisms: Create systems to detect and handle version mismatches
def create_driver_with_fallback():
try:
# Try with latest version
driver = uc.Chrome()
return driver
except Exception as e:
print(f"Error with latest version: {e}")
# Fallback to specific version
options = uc.ChromeOptions()
options.add_argument('--chrome-version=96.0.4664.45') # Example version
driver = uc.Chrome(options=options)
return driver
Handling Detection Events
Even with undetected chromedriver, some sophisticated sites may occasionally detect automation. Implementing proper detection handlers is essential:
def check_for_detection(driver):
# Common detection indicators
detection_signals = [
"//div[contains(text(), 'unusual traffic')]",
"//div[contains(text(), 'robot')]",
"//h1[contains(text(), 'Access Denied')]",
"//iframe[@title='recaptcha']"
]
for xpath in detection_signals:
try:
element = driver.find_element(By.XPATH, xpath)
if element.is_displayed():
return True
except:
pass
return False
# Usage
driver = uc.Chrome()
driver.get("https://example.com")
if check_for_detection(driver):
# Implement recovery strategy
# e.g., rotate IP, clear cookies, try different profile
print("Detection occurred - implementing recovery")
Warning: Some websites employ increasingly sophisticated detection methods that combine multiple signals. Regular testing and adaptation of your approach may be necessary to maintain effectiveness.
Scaling Challenges and Solutions
Scaling undetected chromedriver for large-scale web scraping or automation projects introduces complexities related to resource management, reliability, and detection avoidance. Below are key challenges and strategies to address them effectively.
Challenge: Resource Overload
Running multiple instances of Chrome can consume significant CPU and memory resources, especially in environments with limited hardware capabilities.
Solutions:
- Instance Pooling: Use a pool of Chrome instances managed by a library like
concurrent.futures
or a task queue system to limit the number of concurrent browsers. - Resource Cleanup: Ensure proper termination of Chrome processes using
driver.quit()
and monitor for orphaned processes. - Containerization: Deploy instances in Docker containers with resource limits to isolate and manage resource usage.
from concurrent.futures import ThreadPoolExecutor import undetected_chromedriver as uc def scrape_page(url): driver = uc.Chrome() try: driver.get(url) # Perform scraping tasks return driver.page_source finally: driver.quit() # Manage multiple instances urls = ['https://example.com/page1', 'https://example.com/page2'] with ThreadPoolExecutor(max_workers=3) as executor: results = list(executor.map(scrape_page, urls))
Challenge: Detection at Scale
Running multiple instances increases the risk of detection due to repetitive patterns, shared IP addresses, or excessive request rates.
Solutions:
- Proxy Rotation: Use a pool of residential or high-quality proxies and rotate them for each instance to avoid IP-based bans.
- Fingerprint Diversification: Randomize browser attributes like user-agents, screen resolutions, and plugins across instances.
- Rate Limiting: Implement randomized delays and rate limits to mimic human browsing patterns.
import undetected_chromedriver as uc import random import time def get_driver_with_proxy(proxy_list): options = uc.ChromeOptions() proxy = random.choice(proxy_list) options.add_argument(f'--proxy-server={proxy}') options.add_argument(f'--window-size={random.randint(1200, 1920)},{random.randint(800, 1080)}') return uc.Chrome(options=options) proxies = ['http://proxy1:port', 'http://proxy2:port'] driver = get_driver_with_proxy(proxies) driver.get('https://example.com') time.sleep(random.uniform(1, 3)) # Random delay driver.quit()
Challenge: Task Coordination
Coordinating tasks across multiple instances, such as distributing URLs to scrape or aggregating results, can become complex.
Solutions:
- Task Queues: Use systems like Celery or RabbitMQ to manage task distribution and ensure fault tolerance.
- Centralized Storage: Store scraped data in a database (e.g., MongoDB, PostgreSQL) or distributed file system for easy aggregation.
- Monitoring: Implement logging and monitoring to track instance performance and detect failures early.
from celery import Celery import undetected_chromedriver as uc app = Celery('scraper', broker='redis://localhost:6379/0') @app.task def scrape_task(url): driver = uc.Chrome() try: driver.get(url) # Extract data data = driver.find_element(By.CSS_SELECTOR, '.content').text return {'url': url, 'data': data} finally: driver.quit()
Tip: When scaling, consider cloud-based solutions like AWS EC2 or Google Cloud Compute Engine to distribute instances across multiple servers, improving redundancy and performance.
Essential Tools and Extensions
To maximize the effectiveness of undetected chromedriver, integrating complementary tools and extensions can streamline workflows and enhance capabilities. This section highlights key tools that pair well with undetected chromedriver.
Proxy Management Tools
Proxies are critical for avoiding IP-based bans and simulating diverse user locations.
- ProxyMesh: Offers rotating residential proxies with high reliability.
- Smartproxy: Provides a large pool of residential IPs with easy integration.
- Luminati (Bright Data): Comprehensive proxy management with advanced targeting options.
CAPTCHA Solving Services
When CAPTCHAs are unavoidable, these services can automate or assist with solving them.
- 2Captcha: Affordable CAPTCHA-solving service with API integration.
- Anti-Captcha: Supports various CAPTCHA types, including reCAPTCHA and image-based challenges.
- DeathByCaptcha: Reliable service with high success rates for automated CAPTCHA solving.
Browser Fingerprinting Tools
These tools help analyze and modify browser fingerprints to avoid detection.
- FingerprintJS: Open-source library for generating and analyzing browser fingerprints.
- Chameleon: Browser extension to spoof browser attributes and reduce fingerprint uniqueness.
Automation Frameworks
Combining undetected chromedriver with other automation frameworks can enhance functionality.
- Scrapy: A powerful web scraping framework that can integrate with undetected chromedriver for dynamic content.
- Playwright: A modern automation library that supports stealth features and can complement undetected chromedriver.
- Puppeteer (via Pyppeteer): Python wrapper for Puppeteer, useful for lightweight automation tasks.
# Example: Integrating Scrapy with undetected chromedriver from scrapy.spiders import Spider import undetected_chromedriver as uc class DynamicSpider(Spider): name = 'dynamic_spider' start_urls = ['https://example.com'] def __init__(self): self.driver = uc.Chrome() def parse(self, response): self.driver.get(response.url) # Extract dynamic content yield {'content': self.driver.find_element(By.CSS_SELECTOR, '.dynamic').text} def closed(self, reason): self.driver.quit()
Monitoring and Logging Tools
Monitoring automation performance and logging errors are essential for large projects.
- Sentry: Real-time error tracking and performance monitoring.
- Prometheus: Time-series database for monitoring instance metrics.
- Loguru: Simple and powerful logging library for Python.
Tip: Combine these tools strategically to create a robust automation pipeline. For example, use Scrapy for crawling, undetected chromedriver for rendering dynamic content, and 2Captcha for handling occasional CAPTCHAs.
Alternatives and Comparisons
While undetected chromedriver is a leading solution for bypassing bot detection, several alternatives exist. This section compares undetected chromedriver with other tools and frameworks to help you choose the best option for your needs.
Key Alternatives
Tool | Description | Strengths | Weaknesses |
---|---|---|---|
Playwright | Modern automation library with built-in stealth features | Cross-browser support, lightweight, active development | Less mature than Selenium, steeper learning curve |
Puppeteer | Node.js-based automation library for Chrome | Fast, lightweight, excellent for headless tasks | Node.js ecosystem, limited Python integration |
Selenium with Stealth | Standard Selenium with custom stealth patches | Familiar API, widely used | Requires manual configuration, less robust than undetected chromedriver |
Cloud-Based Solutions (e.g., Bright Data, Zyte) | Commercial scraping platforms with built-in detection evasion | Enterprise-grade, fully managed | Expensive, less customizable |
Comparison with Undetected Chromedriver
Undetected Chromedriver Advantages:
- Seamless integration with existing Selenium codebases
- Automatic patching for new Chrome versions
- Strong community support and frequent updates
- Highly effective against modern bot detection systems
Undetected Chromedriver Limitations:
- Relies on Chrome, limiting cross-browser capabilities
- Resource-intensive compared to lightweight alternatives like Puppeteer
- May require additional tools for advanced fingerprinting evasion
When to Choose Undetected Chromedriver
Opt for undetected chromedriver if:
- You’re already using Selenium and want minimal code changes
- You need robust detection evasion for Chrome-based scraping
- Community-driven updates and open-source solutions are preferred
Consider alternatives if:
- You need cross-browser support (Playwright)
- Lightweight or headless-only automation is critical (Puppeteer)
- Budget allows for fully managed commercial solutions
Case Studies
Real-world applications of undetected chromedriver demonstrate its value across industries. Below are three case studies showcasing its impact.
Case Study 1: E-Commerce Price Monitoring
Scenario: A retail analytics firm needed to monitor prices across multiple e-commerce platforms daily. Standard Selenium was frequently blocked, requiring constant maintenance.
Solution: The firm switched to undetected chromedriver, implementing rotating proxies and randomized delays. They used persistent profiles to maintain login states, reducing CAPTCHA triggers.
Outcome: Price collection success rates increased from 60% to 95%, saving hours of manual intervention and enabling real-time market insights.
Case Study 2: Social Media Data Collection
Scenario: A marketing agency required public post data from a social media platform with strict anti-bot measures.
Solution: Using undetected chromedriver with CDP to spoof geolocation and browser attributes, the agency automated scrolling and data extraction while avoiding detection.
Outcome: The agency collected 10,000+ posts weekly with minimal interruptions, enabling comprehensive sentiment analysis for their clients.
Case Study 3: Web Application Testing
Scenario: A software company needed to test a web application behind a third-party authentication provider that blocked automated browsers.
Solution: The team integrated undetected chromedriver with their Selenium test suite, using JavaScript execution for natural interactions and persistent profiles for session management.
Outcome: Automated tests ran reliably, reducing testing time by 40% and improving release cycles.
Note: These case studies highlight the importance of tailoring undetected chromedriver configurations to specific use cases for optimal results.
Frequently Asked Questions
What is undetected chromedriver?
Undetected chromedriver is a modified version of Selenium’s ChromeDriver that bypasses bot detection systems, enabling reliable web scraping and automation.
Is undetected chromedriver legal to use?
Using undetected chromedriver is legal, but you must comply with website terms of service and applicable laws. Always scrape responsibly and ethically.
Can undetected chromedriver handle CAPTCHAs?
It reduces CAPTCHA triggers but doesn’t solve them directly. Integrate with CAPTCHA-solving services or implement human-in-the-loop strategies for handling CAPTCHAs.
How does undetected chromedriver differ from standard Selenium?
It modifies ChromeDriver to remove automation signatures, adds stealth features, and automatically patches for new Chrome versions, making it harder to detect.
Does undetected chromedriver work in headless mode?
Yes, but headless mode may increase detectability. Non-headless mode with virtual displays is recommended for maximum undetectability.
Tip: Check the GitHub repository (https://github.com/ultrafunkamsterdam/undetected-chromedriver) for additional FAQs and community support.
Conclusion
Undetected chromedriver is a game-changer for Python developers tackling web scraping and automation in an era of sophisticated bot detection. By seamlessly integrating with Selenium, offering robust detection evasion, and benefiting from an active community, it empowers professionals to collect data, test applications, and automate workflows with unprecedented reliability.
From its origins as a simple patch to its current status as a comprehensive automation solution, undetected chromedriver has evolved to meet the demands of modern web environments. Whether you’re a data analyst monitoring market trends, a developer testing web applications, or a researcher gathering public data, this tool provides the flexibility and power needed to succeed.
However, success with undetected chromedriver requires responsible usage, strategic configuration, and ongoing adaptation to new challenges. By combining it with complementary tools, implementing advanced techniques, and respecting ethical boundaries, you can unlock its full potential while maintaining compliance with website policies.
As web technologies continue to evolve, staying informed about updates to undetected chromedriver and emerging detection methods will be critical. Engage with the community, experiment with the techniques outlined in this guide, and leverage the case studies and tools provided to build robust, scalable automation solutions.
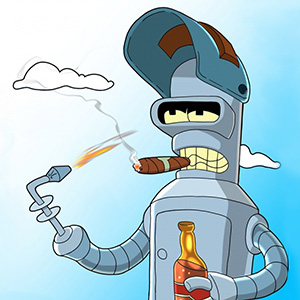
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.