Translating text line-by-line from a file
Today we will translate text line by line from a text file and output the translation similarly, export it to ‘output.txt’. We will use the python library – mtranslate, we will also add the overall progress of the task for general visibility.
First, let’s import all the necessary data to work with the code.
from mtranslate import translate
import os
import re
from tqdm import tqdm
Next, let’s set the paths to the input and output data so that if you move files, you don’t have to manually change them again.
base_dir = os.path.dirname(__file__)
input_file = os.path.join(base_dir, 'input.txt')
output_file = os.path.join(base_dir, 'output.txt')
# Function for text translation
def translate_text(text, target_lang='en', source_lang='auto'):
try:
return translate(text, target_lang, source_lang)
except Exception as e:
print(f"Error when translating a line: {text}\n{e}")
return text # Return the original text in case of an error
# Checking for letters in a string
def contains_letters(text):
return bool(re.search(r'[a-zA-Zа-яА-Я]', text))
# Source and target languages
source_lang = 'auto' # Automatic source language detection
target_lang = 'en' # Translation language
# Reading a file, translating lines and writing the result
try:
# Read all rows in advance to determine the total count
with open(input_file, "r", encoding="utf-8") as infile:
lines = infile.readlines()
with open(output_file, "w", encoding="utf-8") as outfile:
# Using tqdm to display progress
for line in tqdm(lines, desc="String translation", unit="line"):
line = line.strip() # Removing extra spaces and newline characters
if line: # Skip empty lines
if contains_letters(line):
# Translate only letter strings
translated_line = translate_text(line, target_lang, source_lang)
outfile.write(translated_line + "\n")
else:
# We just write down the strings without the letters
outfile.write(line + "\n")
print(f"\nTranslation complete! Results saved to file: {output_file}")
except FileNotFoundError:
print(f"The {input_file} file was not found. Check the path and file name.")
except Exception as e:
print(f"There was an error: {e}")
The advantages of this approach are obvious: you simply insert the text to be translated into the source file, then run the script and wait for the result in a few seconds or minutes (depending on the amount of text). There is no need to pay for separate translation services and API.
Of course, the translation will be mediocre rather than professional, but for general purposes it will be enough.
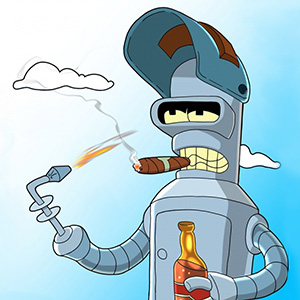
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.