Using tqdm with pandas: Enhance Data Processing with Progress Bars
Overview
The combination of tqdm with pandas brings together pandas
, a cornerstone Python library for data manipulation, and tqdm
, a versatile tool for displaying progress bars. This integration lets you monitor the progress of operations like apply()
, groupby()
, and iterrows()
on pandas DataFrames, offering transparency and a better user experience in your Python data processing tasks.
For data analysts and developers handling large datasets, this duo is a game-changer, turning silent, lengthy processes into visually trackable workflows.
Why Use tqdm with pandas?
Large datasets in pandas can lead to operations that take minutes or even hours. Without feedback, it’s hard to gauge how long a task will run or if it’s stuck. The tqdm
library solves this by adding a progress bar, giving you real-time insights into your Python data processing.
Key Benefits
- Real-time updates for long-running tasks, reducing uncertainty.
- Improved readability of loops and function calls, making code more intuitive.
- Seamless integration with
pandas
DataFrames, requiring minimal setup.
For example, applying a complex function to millions of rows becomes less daunting when you can see progress ticking along.
Installing tqdm for pandas
To use tqdm with pandas, install it via pip:
pip install tqdm
If pandas
isn’t installed yet, grab both in one command:
pip install pandas tqdm
As of March 07, 2025, this ensures you’re running the latest versions. Verify installation with pip show tqdm
and pip show pandas
to check versions and compatibility.
Using tqdm with pandas
Here’s how to integrate tqdm into common pandas
operations with practical examples:
1. Applying tqdm to apply()
The apply()
method is perfect for row-wise or column-wise operations. Add a progress bar using tqdm with pandas apply:
import pandas as pd
from tqdm import tqdm
# Enable tqdm for pandas
tqdm.pandas()
# Sample DataFrame with realistic data
df = pd.DataFrame({'A': range(1, 10001), 'Category': ['X']*5000 + ['Y']*5000})
# Apply function with progress bar
df['B'] = df['A'].progress_apply(lambda x: x * 2 + 3)
This example multiplies each value in ‘A’ by 2 and adds 3, showing progress for 10,000 rows.
2. Using tqdm in groupby()
Operations
For grouped data, track execution with:
result = df.groupby('Category').progress_apply(lambda x: x['A'].sum() * 1.5)
This computes 1.5 times the sum of ‘A’ for each category (‘X’ and ‘Y’), with a progress bar for the group operation.
3. tqdm with iterrows()
While iterrows()
is slower and less efficient, a progress bar can still be useful:
for index, row in tqdm(df.iterrows(), total=len(df), desc="Row Iteration"):
df.loc[index, 'C'] = row['A'] + row['B']
This adds ‘A’ and ‘B’ for each row, storing the result in a new column ‘C’, with progress displayed.
Customizing tqdm in pandas
Tailor the progress bar in pandas with these options:
df['B'] = df['A'].progress_apply(lambda x: x * 2, desc="Doubling Values", position=0, leave=True)
Key Customization Options
desc
: Adds a custom label (e.g., “Doubling Values”).position
: Sets the bar’s display position (0 for top, useful in multi-bar scenarios).leave
: Keeps the bar visible post-completion (True) or hides it (False).
For advanced use, try mininterval
to control update frequency (e.g., mininterval=0.5
for updates every half-second).
Performance Considerations
While pandas tqdm enhances visibility, it’s worth noting its impact:
- Overhead:
tqdm
adds slight overhead, especially withiterrows()
. For small datasets (<1000 rows), it might not be worth it. - Alternatives: For
apply()
, consider vectorized operations (e.g.,df['B'] = df['A'] * 2
) which are faster and don’t need progress bars. - Best Use Case: Use
tqdm
with complex, non-vectorizable functions or massive datasets where timing feedback is critical.
Tip: Profile your code with time.time()
or a library like line_profiler
to decide if tqdm
suits your task.
Conclusion
Integrating tqdm with pandas revolutionizes data processing by providing real-time progress feedback. Whether you’re using apply()
, groupby()
, or iterrows()
, this combo makes Python data workflows more efficient and engaging.
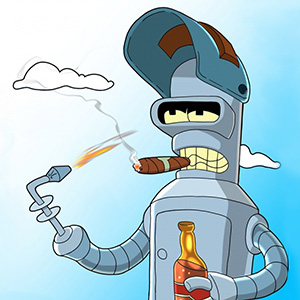
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.