Tqdm Python: Practical Implementation and Customization
Understanding Tqdm and Its Importance
When working with Python scripts, especially those involving extensive data processing, it’s crucial to have a visual representation of execution progress. The tqdm library provides an elegant and efficient way to display progress bars, making it easier to track loop execution and monitor script performance.
- Understanding Tqdm and Its Importance
- Installing Tqdm
- Basic Usage of Tqdm
- Customizing the Progress Bar
- Displaying Dynamic Information
- Using Tqdm with Pandas DataFrames
- Enable tqdm for pandas operations
- Create a sample DataFrame
- Define a function to apply
- Apply the function with progress tracking
- Configure logging
- Wrap tqdm with logging
This tool is particularly useful in scenarios such as long-running computations, data loading, file processing, and any task that requires real-time feedback on execution status.
Installing Tqdm
Before using tqdm, you need to install it in your Python environment. This can be done using pip, the standard package manager for Python:
pip install tqdm
Once installed, you can import tqdm and integrate it into your scripts seamlessly.
Basic Usage of Tqdm
The simplest way to implement tqdm is by wrapping an iterable inside the tqdm()
function. This automatically generates a progress bar that updates as the loop iterates. Here’s an example:
from tqdm import tqdm
import time
for i in tqdm(range(100)):
time.sleep(0.1)
In this example:
tqdm(range(100))
tracks the progress of the loop.time.sleep(0.1)
simulates a process that takes time, allowing you to observe the updating progress bar.
Customizing the Progress Bar
One of the strengths of tqdm is its flexibility. You can modify the appearance, add descriptions, and adjust behavior to match your requirements. Consider the following example:
from tqdm import tqdm
import time
for i in tqdm(range(100), desc="Processing", ascii=True, ncols=80):
time.sleep(0.1)
Here’s what each parameter does:
desc="Processing"
sets a custom label for the progress bar.ascii=True
forces the bar to use ASCII characters (useful in some terminals).ncols=80
sets the bar width to 80 characters.
These customizations make tqdm adaptable to different terminal settings and use cases.
Displaying Dynamic Information
Sometimes, it’s helpful to display real-time updates about specific variables during execution. Tqdm allows you to do this by using set_postfix()
, which dynamically updates additional information next to the progress bar.
from tqdm import tqdm
import time
import random
with tqdm(total=100, desc="Progress") as pbar:
for _ in range(10):
value = random.randint(1, 10)
time.sleep(0.3)
pbar.update(value)
pbar.set_postfix({"Current Value": value})
This script:
- Initializes a progress bar with
total=100
. - Uses
pbar.update(value)
to increment the progress dynamically. - Displays additional information (
"Current Value"
) that changes in real time.
Using Tqdm with Pandas DataFrames
When processing large datasets with pandas, tqdm can be integrated to monitor progress while applying functions to DataFrame columns. The progress_apply()
method enables this functionality:
import pandas as pd
from tqdm import tqdm
import time
# Initialize tqdm for pandas
tqdm.pandas()
# Create a sample DataFrame
df = pd.DataFrame({'A': range(100)})
# Define a sample function to apply
def square(x):
time.sleep(0.1)
return x ** 2
# Apply the function with progress tracking
df['B'] = df['A'].progress_apply(square)
Key aspects of this implementation:
tqdm.pandas()
enables progress tracking within pandas operations.- The
compute_square()
function applies an operation to each row, with progress displayed dynamically.
This approach is invaluable when dealing with large-scale data transformations.
Implementing Nested Progress Bars
For operations that involve multiple levels of iteration, tqdm supports nested progress bars, allowing users to track both inner and outer loops effectively. Here’s an example of how to implement nested progress bars:
from tqdm import tqdm
import time
outer_iterations = 5
inner_iterations = 10
with tqdm(total=outer_iterations, desc='Outer Loop') as pbar_outer:
for i in range(outer_iterations):
with tqdm(total=inner_iterations, desc='Inner Loop', leave=False) as pbar_inner:
for j in range(inner_iterations):
time.sleep(0.1)
pbar_inner.update(1)
pbar_outer.update(1)
Explanation:
- The outer loop tracks major iterations.
- The inner loop has a separate progress bar that updates more frequently.
leave=False
ensures that completed inner progress bars are cleared from the console.
Using Tqdm with Asynchronous Tasks
Tqdm can also be used in asynchronous applications, where tasks run concurrently. The tqdm_asyncio
module helps integrate progress tracking into asynchronous workflows:
import asyncio
from tqdm.asyncio import tqdm_asyncio
async def process_task(index):
await asyncio.sleep(0.5)
return index
async def main():
tasks = [process_task(i) for i in range(10)]
results = await tqdm_asyncio.gather(*tasks)
asyncio.run(main())
In this example:
tqdm_asyncio.gather(*tasks)
executes tasks asynchronously while displaying progress.- This is useful for applications involving I/O-bound operations, such as web scraping or API requests.
Logging Progress with Tqdm
For applications where logging is essential, tqdm can be combined with the logging
module to display progress in log files or terminal outputs:
import logging
from tqdm import tqdm
import time
# Configure logging
logging.basicConfig(level=logging.INFO, format="%(asctime)s - %(message)s")
# Wrap tqdm with logging
for i in tqdm(range(10), desc="Processing"):
time.sleep(0.5)
logging.info(f"Iteration {i} completed")
This approach ensures that progress updates are logged while still displaying real-time progress in the console.
Conclusion
The tqdm library is a highly efficient and customizable tool for tracking the progress of loops, data processing tasks, and asynchronous operations. Its ability to integrate with pandas, handle nested iterations, and support real-time logging makes it invaluable for Python developers.
By leveraging tqdm, you can significantly enhance the usability, performance monitoring, and debugging capabilities of your Python applications.
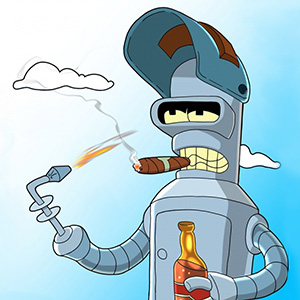
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.