Tqdm Python Guide: Enhance Your Scripts with Progress Bars
Understanding Tqdm and Its Importance
When running Python scripts, especially those handling extensive data processing, tracking progress is essential. The tqdm library offers an elegant solution with customizable progress bars, giving you a clear, visual way to monitor loop execution and script performance. Whether you’re a beginner or a seasoned developer, tqdm Python simplifies the task of keeping tabs on long-running operations.
This tool shines in scenarios like data loading, file processing, or complex computations, providing real-time feedback that boosts usability and debugging. Let’s explore how tqdm transforms your coding experience.
Installing Tqdm
To start using the tqdm library, install it in your Python environment with pip, the standard package manager:
pip install tqdm
Once installed, import it into your scripts with from tqdm import tqdm
. It’s lightweight and integrates seamlessly, ready to enhance your projects.
Basic Usage of Tqdm
The simplest way to use tqdm is by wrapping an iterable with the tqdm()
function. This instantly adds a progress bar that updates as your loop runs. Here’s an example:
from tqdm import tqdm
import time
for i in tqdm(range(100)):
time.sleep(0.1)
What’s Happening:
tqdm(range(100))
: Wraps the loop to track progress.time.sleep(0.1)
: Simulates a time-consuming task, letting you see the bar in action.
This basic setup is perfect for quick monitoring of any iterative task.
Customizing the Progress Bar
Tqdm is highly flexible, letting you tweak its look and behavior. Here’s an example with custom options:
from tqdm import tqdm
import time
for i in tqdm(range(100), desc="Processing", ascii=True, ncols=80):
time.sleep(0.1)
Customization Options:
desc="Processing"
: Adds a label to the bar.ascii=True
: Uses ASCII characters for compatibility.ncols=80
: Sets the bar width to 80 characters.
These tweaks make tqdm adaptable to any project or terminal setup.
Displaying Dynamic Information
Need to show real-time data? Use set_postfix()
to add dynamic updates next to the bar:
from tqdm import tqdm
import time
import random
with tqdm(total=100, desc="Progress") as pbar:
for _ in range(10):
value = random.randint(1, 10)
time.sleep(0.3)
pbar.update(value)
pbar.set_postfix({"Current Value": value})
How It Works:
total=100
: Sets the progress goal.pbar.update(value)
: Increments the bar dynamically.set_postfix
: Displays changing values, like “Current Value”.
This is great for tracking variables during execution.
Using Tqdm with Pandas DataFrames
For large datasets in Pandas, tqdm integrates with progress_apply()
to monitor column operations:
import pandas as pd
from tqdm import tqdm
import time
# Enable tqdm for pandas
tqdm.pandas()
# Sample DataFrame
df = pd.DataFrame({'A': range(100)})
# Function to apply
def square(x):
time.sleep(0.1)
return x ** 2
# Apply with progress bar
df['B'] = df['A'].progress_apply(square)
This is a lifesaver for data-heavy tasks, keeping you informed every step of the way.
Implementing Nested Progress Bars
For multi-level loops, tqdm supports nested bars:
from tqdm import tqdm
import time
outer_iterations = 5
inner_iterations = 10
with tqdm(total=outer_iterations, desc='Outer Loop') as pbar_outer:
for i in range(outer_iterations):
with tqdm(total=inner_iterations, desc='Inner Loop', leave=False) as pbar_inner:
for j in range(inner_iterations):
time.sleep(0.1)
pbar_inner.update(1)
pbar_outer.update(1)
leave=False
keeps the console clean by removing completed inner bars.
Using Tqdm with Asynchronous Tasks
For async workflows, tqdm_asyncio
tracks concurrent tasks:
import asyncio
from tqdm.asyncio import tqdm_asyncio
async def process_task(index):
await asyncio.sleep(0.5)
return index
async def main():
tasks = [process_task(i) for i in range(10)]
results = await tqdm_asyncio.gather(*tasks)
asyncio.run(main())
This is ideal for I/O-bound tasks like API calls or web scraping.
Logging Progress with Tqdm
Combine tqdm with logging
for detailed tracking:
import logging
from tqdm import tqdm
import time
logging.basicConfig(level=logging.INFO, format="%(asctime)s - %(message)s")
for i in tqdm(range(10), desc="Processing"):
time.sleep(0.5)
logging.info(f"Iteration {i} completed")
This logs progress while displaying it live—perfect for debugging.
Conclusion
The tqdm library is a must-have for Python developers, offering an easy, customizable way to add progress bars to scripts. From basic loops to Pandas data processing, nested iterations, and async tasks, tqdm Python enhances usability and performance tracking. Start using it today to make your scripts more interactive and efficient!
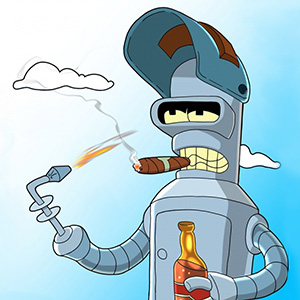
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.