tqdm in Python: Enhancing Progress Visualization
Understanding tqdm: A Progress Bar Library
tqdm, pronounced “taqaddum” (Arabic for progress), serves as a powerful Python library designed to effortlessly create progress bars. This versatile tool proves invaluable when executing time-consuming operations, offering users real-time feedback on task completion. By seamlessly integrating with various Python constructs, tqdm elevates code readability and user experience.
Installation and Basic Usage
To harness the capabilities of tqdm, developers must first install it using pip, Python’s package installer. Execute the following command in your terminal:
pip install tqdm
Once installed, importing tqdm into your Python script is straightforward:
from tqdm import tqdm
With the library imported, you can now wrap any iterable with tqdm to generate a progress bar. Consider this example:
for item in tqdm(range(100)):
# Your processing logic here
pass
This code snippet will display a progress bar that updates as the loop iterates through the range.
Customizing tqdm Progress Bars
tqdm offers extensive customization options, allowing developers to tailor the appearance and behavior of progress bars to suit their specific needs. Let’s explore some of these customization features:
Modifying Bar Appearance
You can alter the visual representation of the progress bar by adjusting various parameters:
from tqdm import tqdm
import time
for i in tqdm(range(100), desc="Processing", unit="item", colour="green"):
time.sleep(0.1)
In this example, we’ve added a description, specified the unit of progress, and changed the bar color to green.
Controlling Update Frequency
For resource-intensive operations, you might want to reduce the update frequency to minimize overhead:
from tqdm import tqdm
import time
for i in tqdm(range(100), desc="Processing", unit="item", colour="green"):
time.sleep(0.1)
Here, miniters
sets the minimum number of iterations between updates, while mininterval
specifies the minimum time between updates in seconds.
Advanced tqdm Features
tqdm’s functionality extends beyond simple progress bars. Let’s delve into some of its more advanced features:
Nested Progress Bars
When dealing with nested loops or multi-stage processes, tqdm enables the creation of nested progress bars:
from tqdm import tqdm
import time
for i in tqdm(range(10), desc="Outer Loop"):
for j in tqdm(range(100), desc="Inner Loop", leave=False):
time.sleep(0.01)
This code will display two progress bars: one for the outer loop and another for the inner loop.
Manual Control
For scenarios where automatic iteration isn’t suitable, tqdm allows manual control of the progress bar:
from tqdm import tqdm
import time
for i in tqdm(range(10), desc="Outer Loop"):
for j in tqdm(range(100), desc="Inner Loop", leave=False):
time.sleep(0.01)
In this example, we manually update the progress bar by 10 units in each iteration.
Integration with Popular Libraries
tqdm seamlessly integrates with numerous popular Python libraries, enhancing their functionality with progress visualization:
Pandas Integration
When working with large datasets in Pandas, tqdm can provide progress feedback for time-consuming operations:
from tqdm import tqdm
import time
with tqdm(total=100) as pbar:
for i in range(10):
time.sleep(0.1)
pbar.update(10)
This code applies a function to each element of a Pandas Series, displaying a progress bar during the operation.
Concurrent Processing with tqdm
tqdm also supports concurrent processing scenarios, such as those involving multiprocessing:
import pandas as pd
from tqdm import tqdm
tqdm.pandas()
df = pd.DataFrame({'A': range(1000)})
df['B'] = df['A'].progress_apply(lambda x: x**2)
from multiprocessing import Pool
from tqdm import tqdm
def process_item(item):
# Simulating a time-consuming operation
time.sleep(0.1)
return item * 2
with Pool(4) as p:
results = list(tqdm(p.imap(process_item, range(100)), total=100))
This example demonstrates how to use tqdm with multiprocessing to visualize progress in parallel computations.
Best Practices and Performance Considerations
While tqdm proves immensely useful, it’s crucial to consider its impact on performance, especially in high-performance computing scenarios. Here are some best practices to optimize tqdm usage:
- Adjust update frequency: For very large iterations, increase
miniters
ormininterval
to reduce overhead. - Use
leave=False
for nested bars: This prevents cluttering the output with multiple completed progress bars. - Leverage context managers: Utilize the
with
statement to ensure proper cleanup of resources. - Monitor memory usage: Be mindful of memory consumption when using tqdm with large datasets or long-running processes.
Conclusion
tqdm stands out as an indispensable tool in the Python ecosystem, offering developers a straightforward yet powerful means to visualize progress in their code. By providing real-time feedback, it enhances user experience and aids in debugging and optimizing time-consuming operations. Whether you’re processing large datasets, performing complex computations, or executing long-running tasks, tqdm’s versatility and ease of use make it an excellent choice for progress visualization in Python projects.
As you incorporate tqdm into your workflows, remember to explore its extensive documentation for additional features and customization options. With practice and experimentation, you’ll find tqdm becoming an integral part of your Python development toolkit, significantly improving the clarity and user-friendliness of your applications.
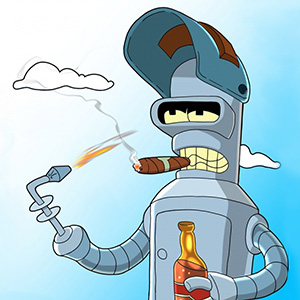
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.