Python – Text Translation with mtranslate: A Line-by-Line Guide
Introduction
In this tutorial, we’ll translate text line-by-line from a file using the Python library mtranslate (Text Translation), saving the results to output.txt
. We’ll enhance the process with tqdm for progress tracking, making this automated translation script both efficient and visible. Ideal for quick, general-purpose translations, this approach avoids costly APIs.
Setup and Imports
Start by importing the required libraries for our Python text translation task:
from mtranslate import translate
import os
import re
from tqdm import tqdm
Next, define dynamic file paths to keep the script portable:
base_dir = os.path.dirname(__file__) # Get script directory
input_file = os.path.join(base_dir, 'input.txt') # Source text file
output_file = os.path.join(base_dir, 'output.txt') # Translated output file
The Translation Script
Here’s the complete script to read, translate, and write text with progress visibility:
from mtranslate import translate
import os
import re
from tqdm import tqdm
# Dynamic file paths
base_dir = os.path.dirname(__file__)
input_file = os.path.join(base_dir, 'input.txt')
output_file = os.path.join(base_dir, 'output.txt')
def translate_text(text, target_lang='en', source_lang='auto'):
"""Translate text using mtranslate, with error fallback."""
try:
return translate(text, target_lang, source_lang)
except Exception as e:
print(f"Translation error for '{text}': {e}")
return text # Fallback to original text
def contains_letters(text):
"""Check if text contains alphabetic characters."""
return bool(re.search(r'[a-zA-Zа-яА-Я]', text))
# Language settings
source_lang = 'auto' # Auto-detect source language
target_lang = 'en' # Target language: English
try:
# Pre-read lines for accurate progress tracking
with open(input_file, "r", encoding="utf-8") as infile:
lines = infile.readlines()
with open(output_file, "w", encoding="utf-8") as outfile:
# Translate with progress bar
for line in tqdm(lines, desc="Translating lines", unit="line"):
line = line.strip() # Clean whitespace
if line: # Skip empty lines
if contains_letters(line):
translated_line = translate_text(line, target_lang, source_lang)
outfile.write(f"{translated_line}\n")
else:
outfile.write(f"{line}\n") # Non-alphabetic lines unchanged
print(f"\nTranslation completed! Results saved to: {output_file}")
except FileNotFoundError:
print(f"Error: '{input_file}' not found. Verify the file path.")
except Exception as e:
print(f"Unexpected error: {e}")
How it works:
- Reads
input.txt
line-by-line. - Translates only lines with letters, preserving others (e.g., numbers).
- Shows progress with
tqdm
. - Saves results to
output.txt
.
Advantages
This mtranslate tutorial offers clear benefits:
- Simplicity: Add text to
input.txt
, run the script, and get results fast. - Cost-Free: No API fees—just Python and
mtranslate
. - Visibility: Progress tracking with
tqdm
keeps you informed.
While translations may lack professional polish, they suffice for general use in 2025.
Conclusion
This automated translation script using mtranslate
and tqdm
makes Python text translation accessible and efficient. Perfect for quick translations of text files, it’s a practical tool for developers, researchers, or anyone needing basic translation without complexity. Try it with your own input.txt
and see the results!
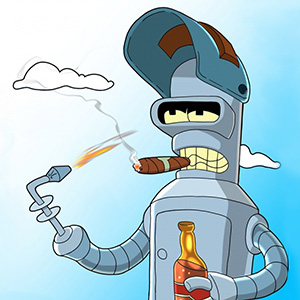
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.