Comprehensive Guide to Telegram Scraping
Overview of Telegram Scraping
As an experienced data extraction specialist, I often get asked about the best practices for Telegram scraping. Telegram presents unique challenges for scrapers due to its encryption protocols and chat structure. However, with the right techniques, it is possible to systematically extract data from Telegram channels and groups.
In this end-to-end walkthrough, I elucidate my time-tested process for extracting targeted Telegram data at scale. Whether your goals involve monitoring consumer feedback, gaining competitive intelligence, analyzing trends based on hashtags or keywords, or extracting chat data for other business purposes – following these methodical steps enables accessing the deep riches of Telegram’s widespread conversation ecosystem legally and ethically. With over 700 million active users exchanging messages, media and file across public and private groups daily, Telegram presents a potential goldmine for those who know how to tap into it systematically. By approaching data extraction in a thoughtful, customized way and not taking shortcuts, you can unlock access to Telegram’s immense reservoirs of unstructured data to transform into actionable insights.
Prerequisites for Telegram Scraping
Before diving into the how-to guide, it is crucial to cover the prerequisites. Attempting to scrape Telegram without these elements in place will likely result in limited or inconsistent data.
Telegram Account
Naturally, the first requirement is having your own Telegram account. I recommend using a virtual phone number when signing up to avoid linking your personal number.
Identify Target Chats
You will need to identify the specific groups, channels, or individual message feeds you want to track. Telegram offers search tools to find public chats by name, topic, members, etc. Make a list of your chat targets before scraping.
Scraping Tools
Manual Telegram scraping is unrealistic. You will need a specialized scraping software suite designed to interact with Telegram’s API. I recommend using tools that offer proxies, automation, chat identification, OAuth pass-through capabilities, and data export options.
Scraping Methodology
Now that the prerequisites are fulfilled, I can walk through my exact process for extracting Telegram data start to finish:
Configure Scraping Tools
Every software tool has its own specifics, but most Telegram solutions have similar configuration screens. You will want to set the chats you identified for scraping, schedule scraping frequency, assign proxies, designate data exports, and other settings.
Take time to carefully customize these configurations – it will save you hassle later.
Authentication
Telegram strictly restricts scraping from unauthorized accounts to combat spam and abuse. Your scraper must authenticate through OAuth so it has access permission to channel data.
Most tools simplify authentication – you log into your Telegram account within the software interface itself. This passes valid access credentials to the scraper for data extraction.
Test Extraction
Before launching a full scraping effort, always do short test runs. Scrape different chat types to ensure you are capturing expected data fields, text formats, metadata etc.
Compare scraped data vs manually exported Telegram data to confirm accuracy. Tweak tool settings if certain elements are missing or inconsistent.
Run Automated Scraper
You are now ready for launch! Enable your scheduler to trigger the scraper on set intervals. For high volume data feeds, scrape as often as every few minutes.
Monitor the first few scrapes closely to verify smooth operations before letting automation run continually.
Export Scraped Conversations
As a final step, export extracted conversations from your scraping tool into local files or connect to third-party analytics, BI or visualization platforms.
Transformation helps avoid data overload within your scraper suite itself. You get to focus insights on target metrics rather than getting lost in the Telegram data.
Conclusion & Next Steps
When executed systematically, Telegram scraping unlocks access to data goldmines hidden within encrypted conversations. Following the methodology above allows extracting targeted information safely, accurately and at scale.
For next steps, the priority is identifying your core analytics needs. Transform scraped Telegram data into actionable insights tailored to your business goals. With the foundation covered here, the possibilities are truly wide open.
Let me know if you have any other questions! I have nicknamed myself “The Telegram Scraper” given my depth of experience – so I am always glad to offer advice to new scrapers getting started.
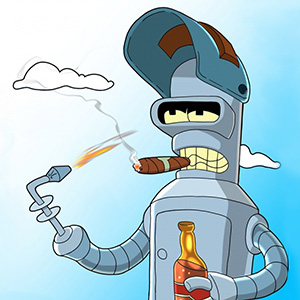
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.