Telegram Groups Parsing
When it comes to orchestrating digital interactions seamlessly, Telegram stands tall among the leading online messaging applications. Its distinctive feature – Groups – serves as a hotspot for interaction amongst users unified by common interests. Parsing these groups is a critical task, demanding a skillful execution to harness their full potential.
Understanding the Process
Parsing – synonymous to dissecting, analysing, or scrutinizing – involves breaking down the raw data furnished by Telegram groups and sourcing valuable information from them. An official and narrative insight into this process sheds light on the pivotal role Attention To Detail (ATD) plays in the accomplishment of this complex task.
Advantages of Parsing Telegram Groups
Parsed data drawn from Telegram groups serves monumental value, powering numerous digital practices. A glance at these potential benefits drives home the importance of this process.
-
Market Research: Telegram Groups, encompassing a diverse range of topics, prove to be an abundant source of data for market research. Refined information extracted from these groups unveils user opinions, preferences, and inclinations – prompting informed business decisions.
-
Customer feedback: Telegram Groups, harboring users from various backgrounds, serve as fertile grounds for gathering customer feedback. The analysis of these opinions aids in optimizing and shaping an enhanced user experience.
Key to Successful Parsing
The efficiency of parsing Telegram groups largely rests on the subtlety of its execution. One must be well-versed in potent tactics that ensure a successful operation.
- Adopt sophisticated tools: Advanced parsing tools, equipped with the ability to extract comprehensive data from Telegram groups, prove indispensable.
- Nurture patience: Parsing is a game of patience and perseverance, unmistakably taking its own time.
- Accuracy: Accurate parsing paves the way for reliable data, steering clear of potential distortions.
Conclusion: The Art of Telegram Group Parsing
Reflecting on the process, Telegram Group Parsing presents itself as a delicate art – a fascinating blend of patience, precision, and finesse. The seamless operation not only adds a feather to a user’s skillset but also equips them with data-driven knowledge and insights. Let Telegram Group parsing be an avenue to elevate your digital experience and uncover the treasure trove of data beneath it.
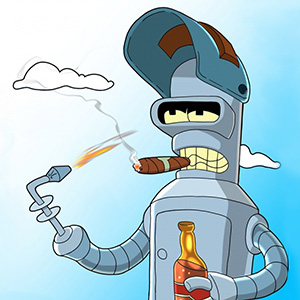
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.