Tags Parsing
Parsing tags in code is an essential skill for any developer working with marked up content. Whether you are extracting metadata from HTML, handling XML configuration files, or parsing document formats like Markdown or reStructuredText, having a solid understanding of common parsing techniques can save you time and headache. In this article, we will cover the basics of tag parsing in depth, looking at methods like regular expressions, parser libraries, and building your own custom parsers. Any competent developer should feel comfortable parsing tags in their code by the end of this guide.
Regular Expression Parsing
One of the most common ways to parse tags is using regular expressions (regex). Regex allows you to search for patterns and extract matches in strings. For example, say you wanted to get all <h1>
tags from an HTML document. Using JavaScript, you could do:
Here we are searching for an opening <h1>
tag, capturing any characters in between, and then matching the closing </h1>
tag. The .match()
method returns an array of matches, giving us the full extracted tags.
Regular expressions are powerful, but can get complex fast. You need to carefully craft the pattern to match your use case. They also do not offer much structure or context around the extracted tags. Still, for simple tag parsing they are quick and lightweight.
Using a Parser Library
To avoid reinventing the wheel, many developers rely on parser libraries to handle tag parsing for them. There are libraries available for most languages that can parse HTML, XML, and other markup languages.
For example, in Python there is the BeautifulSoup
library. It allows you to load a document and traverse/search the parse tree:
from bs4 import BeautifulSoup
html = """
Paragraph
"""
soup = BeautifulSoup(html, 'html.parser')
h1 = soup.find('h1')
print(h1.text) # Title
The benefit here is BeautifulSoup
handles all the underlying parsing and document structure. We can query elements directly without having to craft complex regex.
There are similar parsing libraries available across languages and platforms, like lxml
for Python, Nokogiri
for Ruby, or DOMParser
in JavaScript. Finding one that fits your needs can save time compared to hand-rolling your own parser.
Building a Custom Parser
For more advanced use cases, you may want full control over how your markup gets parsed. In these instances, building your own custom parser can be beneficial. You define the grammar and structuring rules, allowing your parser to understand your particular document format.
This is a complex endeavor, best served by breaking it down into phases:
1. Lexical Analysis
Break input into tokens. This could be looking for start/end tags, attribute strings, or other lexical elements.
2. Syntax Analysis
Group tokens and validate if they follow grammar rules. For example, ensure proper tag nesting.
3. Abstract Syntax Tree
Construct a tree representing the syntactic structure. Tags become nodes, attributes are branches.
4. Semantic Analysis
Further analyze the tree for logical errors. Do attribute values make sense? Required children present?
5. Evaluation
With a validated tree, execute handlers to extract data or transform to desired output structure.
Following these phases yields a robust, custom parser tuned exactly to your document format. For markup heavy applications, investing time in a custom parser can pay off long term in maintainability and performance.
Parsing Tag Attributes
A key part of parsing markup involves handling tag attributes. These are the properties attached to opening tags that provide metadata. For example:
The src
and alt
are attributes on the <img>
tag. To access these during parsing, we need to extract the attribute strings and parse into name/value pairs.
With regex, we could match the general pattern of attributes using a group:
const tagPattern = /<(\w+)(.*?)>/;
const match = ''.match(tagPattern);
const tagName = match[1]; // img
const attrsStr = match[2]; // src="photo.jpg" alt="A nice photo"
From there additional logic would be needed to split attrsStr
into individual name/value pairs.
When using a parsing library, we get access to built-in methods for handling attributes:
img = soup.find('img')
src = img['#']
alt = img.get('alt') # A nice photo
The library handles the underlying attribute parsing, exposing them as dictionary properties on the tag.
For custom parsers, attributes can be processed during syntax analysis. Tokens can be matched to an attribute pattern, with the lexer outputting distinct attribute tokens. These then get processed when constructing the syntax tree.
Regardless of approach, dealing with attributes is an important part of fully leveraging the metadata in tags.
Optimizing Tag Parsing Performance
Parsing markup and generating an abstract syntax tree can be resource intensive. Large or complex documents may contain thousands of tags and require many processing steps. Here are some techniques to optimize parser performance:
-
Streaming: Parse on-demand in a stream rather than loading an entire document upfront.
-
Caching: Store parsed trees to avoid re-parsing identical content.
-
Parallelism: Process independent subtrees concurrently across threads/processes.
-
Lazy evaluation: Only parse when output is actually needed rather than eagerly.
-
Compiled evaluators: Use bytecode or JIT compilation to evaluate parsers faster.
-
Memory management: Reuse existing memory and buffers where possible to reduce overhead.
Understanding these performance optimization patterns will allow you to parse tags quickly at scale across any language.
Conclusion
Parsing structured markup is a necessary skill for many different kinds of software development. In this article we explored common techniques used to extract and process tags, looking at regular expressions, libraries, and building custom parsers. We also covered important considerations like handling attributes and optimizing for performance. Tag parsing enables extracting metadata and building interpreters for markup languages. With the methods outlined here, you should feel empowered tackling tag parsing in your own projects.
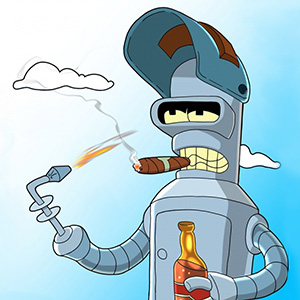
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.