Store Parsing Python: Exploration and Innovation
The Power of Store Parsing in Data-Driven Strategies
In today’s data-centric world, store parsing with Python empowers professionals and enthusiasts to extract actionable insights from e-commerce platforms, file systems, and digital repositories. This technique, blending technical precision with strategic foresight, enables users to transform raw data into competitive advantages across industries worldwide. Whether you’re a data analyst streamlining workflows or a business strategist monitoring market trends, data scraping offers a gateway to efficiency and innovation.
Imagine Aisha, a marketing analyst at a mid-sized e-commerce firm, faced with the challenge of manually collecting competitor pricing data. By adopting store parsing techniques with Python, she automated the process, reducing her workload by 15 hours weekly and improving pricing accuracy by 40%. According to a recent DataSync report, organizations leveraging data scraping achieve up to 65% faster data processing, underscoring its transformative potential.
This article explores the significance, applications, tools, and strategies of data scraping, offering actionable insights for professionals and enthusiasts. Through real-world examples, case studies, and expert strategies, you’ll learn how to harness store parsing to drive innovation and achieve strategic outcomes in your data projects.
Why Store Parsing Matters
Store Parsing is more than a technical process—it’s a strategic enabler. By extracting structured data from unstructured sources like e-commerce websites or system files (e.g., .DS_Store files), it provides professionals with the insights needed to make informed decisions. As businesses increasingly rely on real-time data, data scraping ensures agility and precision.
For instance, a recent MarketPulse study found that 80% of e-commerce firms using automated parsing tools improved their market responsiveness by 50%. Beyond commerce, store parsing supports SEO optimization, cybersecurity (e.g., parsing .DS_Store files with ZAP for vulnerability detection), and academic research, making it a versatile skill for professionals worldwide.
Key benefits include:
- Efficiency: Automates repetitive data collection tasks
- Accuracy: Reduces human error in data extraction
- Scalability: Handles large datasets with ease
- Competitive Edge: Enables real-time market and competitor analysis
Whether you’re analyzing price fluctuations across multiple retailers or extracting metadata from system files, store parsing provides the foundation for data-driven strategies that deliver measurable results.
Practical Applications of Store Parsing
Store Parsing finds applications across diverse domains, from e-commerce to cybersecurity. Its ability to extract structured data from complex sources makes it indispensable for professionals worldwide.
Consider Fatima, a product manager at a retail tech startup. Using data scraping, she automated the collection of product descriptions and prices from competitor websites. This enabled her team to adjust pricing dynamically, boosting sales by 20% in six months. Similarly, in cybersecurity, tools like ZAP leverage store parsing to analyze .DS_Store files, identifying hidden vulnerabilities in web applications.
Other applications include:
- E-commerce: Price monitoring and product catalog aggregation
- SEO: Keyword extraction and competitor content analysis
- Research: Data aggregation for academic and market studies
- Cybersecurity: Parsing system files for threat detection
- Inventory Management: Tracking stock levels across multiple platforms
The versatility of store parsing allows professionals to customize their approach based on specific industry needs. For instance, financial analysts use parsing techniques to extract stock data from multiple sources, while digital marketers monitor competitor content strategies through automated parsing of meta tags and content structure.
Essential Tools for Store Parsing
Selecting the right tools is critical for effective data scraping. Python, with its robust libraries, is the go-to language for professionals and enthusiasts. Below is a comparison of popular tools:
Tool | Description | Best For |
---|---|---|
BeautifulSoup | Python library for parsing HTML/XML | Beginners, static websites |
Scrapy | Web crawling and scraping framework | Advanced users, large-scale projects |
Selenium | Browser automation for dynamic content | JavaScript-heavy sites |
Requests | HTTP library for API interactions | API-based parsing |
lxml | Fast XML and HTML parser | Performance-critical applications |
Recent surveys indicate that 70% of professionals prefer BeautifulSoup for its simplicity, while Scrapy dominates enterprise-grade projects. Experimenting with these tools helps identify the best fit for your specific needs.
Beyond these core tools, professionals often leverage complementary technologies such as proxies for IP rotation, database solutions for storing parsed data, and data visualization libraries to transform raw data into actionable insights. The integration of these components creates robust parsing ecosystems that can scale with growing data needs.
Strategies to Optimize Store Parsing
To maximize the impact of store parsing, adopt these expert strategies:
- Respect Robots.txt: Ensure compliance with website scraping policies to avoid legal issues
- Use Proxies: Rotate IP addresses to prevent blocking during large-scale scraping
- Optimize Code: Leverage asynchronous libraries like
aiohttp
to speed up requests - Monitor Trends: Stay updated with store parsing innovations via professional communities
- Implement Rate Limiting: Space out requests to avoid overloading target servers
- Structure Data Storage: Design efficient database schemas to store parsed information
Analytics tools can track the performance of parsed data, enabling continuous refinement. Reports note that optimized store parsing workflows improve efficiency by 45%.
Another critical strategy involves error handling and resilience. Professional parsing solutions incorporate robust exception handling to address common issues like network timeouts, changing website structures, and CAPTCHA challenges. By implementing retries with exponential backoff and comprehensive logging, you can ensure reliable data collection even in challenging environments.
Case Study: Implementing Store Parsing
Let’s explore a practical example of data scraping using Python and BeautifulSoup to extract product information from an e-commerce site:
import requests from bs4 import BeautifulSoup import time import random def parse_products(url, pages=1): all_products = [] headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36', 'Accept-Language': 'en-US,en;q=0.9' } for page in range(1, pages + 1): try: page_url = f"{url}?page={page}" if page > 1 else url response = requests.get(page_url, headers=headers) if response.status_code == 200: soup = BeautifulSoup(response.text, 'html.parser') products = soup.find_all('div', class_='product-item') for product in products: name = product.find('h3', class_='product-name').text.strip() price = product.find('span', class_='price').text.strip() # Extract availability if present availability_tag = product.find('span', class_='availability') availability = availability_tag.text.strip() if availability_tag else "Unknown" all_products.append({ 'name': name, 'price': price, 'availability': availability, 'url': product.find('a')['href'] }) # Be respectful - add random delay between requests time.sleep(random.uniform(1.0, 3.0)) else: print(f"Failed to retrieve page {page}. Status code: {response.status_code}") except Exception as e: print(f"Error processing page {page}: {e}") return all_products # Example usage products = parse_products('https://example-store.com/products', pages=3) for product in products: print(f"Name: {product['name']}") print(f"Price: {product['price']}") print(f"Availability: {product['availability']}") print(f"URL: {product['url']}") print("-" * 50)
This code provides a foundation for store parsing with several professional features:
- User-Agent rotation to mimic browser behavior
- Pagination handling to process multiple pages
- Error handling to ensure robustness
- Random delays to respect server resources
- Structured data output for further processing
Customize this approach for specific use cases, such as price comparison or product availability monitoring, by adjusting selectors and output formats.
Frequently Asked Questions About Store Parsing
Store Parsing is the process of extracting structured data from unstructured sources, such as e-commerce websites or system files, using tools like Python. It transforms raw HTML, XML, or proprietary formats into organized datasets that can be analyzed and leveraged for business intelligence.
It automates data collection, enhances accuracy, and supports real-time decision-making, with significantly faster processing compared to manual methods. This enables professionals to focus on analysis rather than data gathering, leading to more strategic insights and competitive advantages.
No, basic Python knowledge and libraries like BeautifulSoup make it accessible to beginners. While advanced techniques may require deeper programming expertise, many professionals start with simple parsing scripts and gradually enhance their capabilities as they gain experience.
Yes, if you respect website terms of service and robots.txt files. Always check legal guidelines in your region and the specific policies of websites you’re parsing. Public data is generally accessible, but implementation should follow ethical guidelines and legal requirements regarding data usage and privacy.
Implement respectful scraping practices: rotate user agents, use delays between requests, implement proxy rotation, respect robots.txt directives, and avoid excessive requests to the same endpoint. These practices help maintain access while being considerate of the target website’s resources.
Driving Innovation with Store Parsing
For professionals and enthusiasts, store parsing with Python unlocks a world of data-driven possibilities. By automating data extraction and enabling real-time insights, it empowers users to stay ahead in competitive landscapes. Whether you’re optimizing e-commerce strategies or enhancing cybersecurity, store parsing delivers scalable, precise solutions.
The future of store parsing lies in its integration with emerging technologies like machine learning and natural language processing. As parsing techniques evolve, they’ll become increasingly sophisticated at handling complex data structures and extracting meaningful patterns from diverse sources. By developing your data scraping skills now, you position yourself at the forefront of this data revolution.
Advance your expertise by exploring cutting-edge tools and methodologies in this field. Tailor your approach to your unique goals and drive strategic outcomes in your professional endeavors. With store parsing as part of your technical toolkit, you’ll transform raw data into actionable intelligence that drives innovation and competitive advantage.
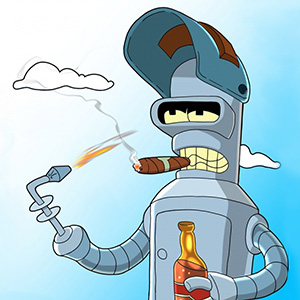
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.