7 Powerful Ways to Master Scraping VK with Python in 2025
Introduction to Scraping VK with Python
For developers and data professionals, Scraping VK with Python unlocks a wealth of insights from one of the largest social networks in the Russian-speaking world. Whether you’re analyzing user trends, gathering market data, or building innovative tools, this guide offers practical techniques to navigate VK’s complexities. Designed for professionals globally, it blends hands-on advice with expert strategies to ensure your scraping projects are efficient, ethical, and impactful.
Python’s flexibility makes it ideal for web scraping, and VK’s dynamic platform presents unique opportunities alongside challenges. From configuring your environment to tackling rate limits, we’ll cover everything you need to succeed. Let’s explore why VK is a valuable data source and how to scrape it responsibly.
VK, often compared to Facebook, hosts millions of public posts, groups, and profiles ripe for analysis. But scraping isn’t just about grabbing data—it’s about doing so thoughtfully. This article equips you with the tools and knowledge to start small and scale up, all while staying compliant with VK’s terms.
Whether you’re a data scientist, marketer, or developer, you’ll find actionable tips here. We’ll walk through setup, basic methods, advanced techniques, and troubleshooting, ensuring you’re ready for any VK scraping project. Let’s get started.
Why Scrape VK? Understanding the Platform’s Value
VK (VKontakte) commands over 100 million monthly active users, making it a goldmine for data-driven projects. Its open structure—unlike many social platforms—allows access to public posts, groups, and profiles, perfect for market research, sentiment analysis, or competitive intelligence. For professionals, scraping VK reveals trends in sectors like gaming, music, or e-commerce, with a strong foothold in Eastern Europe and beyond.
The platform’s data fuels machine learning models, academic studies, or business strategies. A 2023 Statista report noted VK’s dominance in Russia’s social media scene, with 73% of internet users engaging daily. Scraping public group posts can uncover consumer preferences, while profile data (where permitted) informs demographic studies. But VK’s API and terms set strict boundaries—ignoring them risks bans.
Scraping VK isn’t just about volume; it’s about precision. You can extract targeted insights, like how gaming communities discuss new releases or how brands engage audiences. This section sets the foundation for why VK matters and how Python makes scraping accessible.
Still, responsibility is key. Overloading VK’s servers or scraping private data violates ethics and laws. By focusing on public, authorized data, you tap into VK’s potential safely. Let’s look at the tools that make this possible.
Essential Tools and Libraries for Scraping VK
Python’s ecosystem powers efficient web scraping, and choosing the right tools is critical for VK scraping. Below, we outline the top libraries and their roles, tailored for professionals seeking streamlined workflows.
Tool/Library | Purpose | Why Use It? |
---|---|---|
Requests | HTTP requests | Simple API calls to VK’s endpoints. |
BeautifulSoup | HTML parsing | Extracts data from VK’s web pages easily. |
Selenium | Browser automation | Handles dynamic content like infinite scroll. |
vk_api | VK API interaction | Official access to VK data, reducing risks. |
Pandas | Data handling | Organizes scraped data for analysis. |
These tools cover most scraping needs. For instance, Requests fetches API data, while BeautifulSoup parses HTML from public pages. Selenium shines for JavaScript-heavy content, and vk_api ensures compliance with VK’s terms. Pandas ties it all together, turning raw data into actionable insights.
Mix and match based on your goals. If you’re pulling group posts, vk_api is enough. For scraping public profiles without API access, combine Requests and BeautifulSoup. Always check VK’s API docs for updates, as endpoints shift. Next, we’ll tackle the ethical side to keep your projects safe.
Pro tip: Install these libraries in a virtual environment to avoid conflicts. We’ll cover setup details soon, ensuring you’re equipped for success.
Ethical and Legal Considerations
Scraping VK requires navigating a minefield of ethical and legal rules. VK’s terms of service encourage API use for authorized data, but aggressive scraping can trigger IP bans or worse. Professionals must focus on public data—like group posts or open profiles—while steering clear of personal or private information.
Global regulations add complexity. GDPR governs EU users, while Russia’s data laws protect VK’s local base. A 2024 DataReportal study found 62% of social media users demand transparency in data handling. Respecting these expectations builds trust and keeps your projects compliant.
Use VK’s API whenever possible—it’s the safest route. If you must scrape web pages, mimic human behavior with delays and randomized headers. For example, scraping public event pages for attendance trends is fine, but harvesting private messages isn’t. Ethical scraping prioritizes user privacy and platform rules.
Document your process, too. If you’re working for a client or employer, clear records of what you scraped and why demonstrate accountability. This approach minimizes risks and aligns with professional standards worldwide.
Setting Up Your Python Environment
A solid Python setup is the backbone of any VK scraping project. For professionals, a clean, secure environment saves time and prevents errors. This section guides you through installing Python, creating a virtual environment, and adding libraries for scraping VK, ensuring global applicability.
Start with Python 3.8 or higher—VK’s API and libraries demand it. Download from python.org if needed. Then, set up a virtual environment to isolate dependencies:
python -m venv vk_scraper_env
source vk_scraper_env/bin/activate # On Windows: vk_scraper_env\Scripts\activate
With the environment active, install key libraries:
pip install requests beautifulsoup4 vk_api pandas python-dotenv selenium
This covers API access (vk_api), web scraping (Requests, BeautifulSoup), dynamic pages (Selenium), and data handling (Pandas). Python-dotenv secures credentials. If using Selenium, download a WebDriver like ChromeDriver compatible with your browser version.
Secure your VK API token in a `.env` file. Install python-dotenv first, then create the file:
VK_TOKEN=your_vk_api_token_here
Load it in your script:
from dotenv import load_dotenv
import os
load_dotenv()
vk_token = os.getenv('VK_TOKEN')
Test your setup by importing each library in a Python script. No errors? You’re ready. This configuration supports both API and web scraping, giving you flexibility for any project, from small experiments to large-scale data collection.
One tip: keep your environment updated. Run `pip list –outdated` monthly to spot old packages. An up-to-date setup ensures compatibility with VK’s evolving platform.
With your tools in place, let’s move to scraping techniques that deliver results.
Basic VK Scraping Techniques
Let’s hit the ground running with basic VK scraping methods. These techniques are perfect for professionals starting out, focusing on public data like group posts or page titles using Python’s vk_api and web scraping tools. They’re simple, effective, and globally relevant.
The VK API, accessed via vk_api, is your safest bet. Get a token from VK’s developer portal—create a standalone app, enable permissions (e.g., “wall”), and copy the token. Here’s a script to fetch 10 recent posts from a public group:
import vk_api
from dotenv import load_dotenv
import os
load_dotenv()
vk_token = os.getenv('VK_TOKEN')
vk_session = vk_api.VkApi(token=vk_token)
vk = vk_session.get_api()
group_id = '-123456' # Negative for groups
posts = vk.wall.get(owner_id=group_id, count=10)
for post in posts['items']:
print(f"Post ID: {post['id']}, Text: {post['text'][:50]}...")
This pulls post IDs and text snippets, respecting VK’s terms. Adjust `count` or add filters (e.g., `filter=’photo’`) for specific data. It’s low-risk and ideal for quick insights.
For non-API scraping, use Requests and BeautifulSoup to grab public page data, like a group’s title:
import requests
from bs4 import BeautifulSoup
url = 'https://vk.com/public123456'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) Chrome/91.0.4472.124'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.find('title').text
print(f"Group Title: {title}")
This targets static HTML elements. VK’s dynamic pages may require Selenium for deeper scraping, but we’ll cover that later. For now, focus on simple tags like titles or post containers.
Save your data with Pandas for analysis:
import pandas as pd
data = [{'id': post['id'], 'text': post['text']} for post in posts['items']]
df = pd.DataFrame(data)
df.to_csv('vk_posts.csv', index=False)
print("Data saved to vk_posts.csv")
This creates a CSV you can explore with tools like Excel or Python. These methods—API calls and light web scraping—lay a solid foundation. They’re easy to tweak for projects like trend analysis or content audits.
One caveat: always add delays (e.g., `time.sleep(1)`) for web scraping to avoid overwhelming VK’s servers. Respect rate limits to stay in the clear.
Mastered the basics? Let’s level up with advanced strategies for tougher tasks.
Advanced Scraping Strategies
Once you’ve nailed basic VK scraping, it’s time to tackle complex projects. Advanced techniques let professionals extract richer data—like user interactions, multimedia, or historical posts—while navigating VK’s dynamic structure. These methods, built for global use, blend API power with web scraping finesse.
First, maximize VK’s API for bulk data. The `execute` method runs multiple API calls in one request, boosting efficiency. For example, to fetch posts from multiple groups:
import vk_api
from dotenv import load_dotenv
import os
load_dotenv()
vk_token = os.getenv('VK_TOKEN')
vk_session = vk_api.VkApi(token=vk_token)
vk = vk_session.get_api()
code = '''
var groups = ["-123456", "-789012"];
var result = [];
var i = 0;
while (i < groups.length) {
result.push(API.wall.get({"owner_id": groups[i], "count": 5}));
i = i + 1;
}
return result;
posts = vk_session.method('execute', {'code': code})
for group_posts in posts:
for post in group_posts['items']:
print(f"Post ID: {post['id']}, Text: {post['text'][:50]}...")
This pulls posts from several groups at once, saving API calls. VK limits `execute` to 25 requests per batch, so plan carefully. Use it for tasks like comparing engagement across communities.
For dynamic content—like comments or infinite-scroll feeds—Selenium is your friend. It simulates a browser to load JavaScript-heavy pages. Here’s how to scrape comments from a post:
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
driver = webdriver.Chrome() # Ensure ChromeDriver is installed
driver.get('https://vk.com/wall-123456_789')
time.sleep(3) # Wait for page load
comments = driver.find_elements(By.CLASS_NAME, 'reply_text')
for comment in comments[:5]:
print(f"Comment: {comment.text[:50]}...")
driver.quit()
This grabs comment text, but VK’s class names change, so inspect elements regularly. Selenium is slower than Requests, so reserve it for tasks the API can’t handle, like scraping event RSVPs.
Handling multimedia? VK’s API exposes photo and video URLs in post attachments. Extract them like this:
posts = vk.wall.get(owner_id='-123456', count=10)
for post in posts['items']:
if 'attachments' in post:
for attachment in post['attachments']:
if attachment['type'] == 'photo':
url = attachment['photo']['sizes'][-1]['url']
print(f"Photo URL: {url}")
This retrieves high-resolution images. For videos, check `attachment['video']`. Save URLs to a database or download files with Requests for analysis, like studying visual trends.
Scale up with asynchronous scraping using `aiohttp` for speed. Here’s a quick example to fetch multiple pages concurrently:
import aiohttp
import asyncio
async def fetch_page(url, session):
async with session.get(url) as response:
return await response.text()
async def main():
urls = ['https://vk.com/public123456', 'https://vk.com/public789012']
async with aiohttp.ClientSession() as session:
tasks = [fetch_page(url, session) for url in urls]
pages = await asyncio.gather(*tasks)
for page in pages:
print(f"Page length: {len(page)}")
asyncio.run(main())
This cuts scraping time for large datasets. Install `aiohttp` with `pip install aiohttp` and combine with BeautifulSoup for parsing. It’s ideal for professionals scraping thousands of posts.
Advanced scraping demands care. Rotate user agents, use proxies, and monitor VK’s API limits (typically 5,000 calls daily). These strategies unlock deeper insights, but challenges like bans or CAPTCHAs can arise. Let’s tackle those next.
These methods push your projects further, but staying ethical is non-negotiable. Always align with VK’s rules.
Overcoming Common Scraping Challenges
Scraping VK isn’t always smooth sailing. Professionals face hurdles like rate limits, dynamic content, CAPTCHAs, and IP bans. This section offers practical solutions to keep your Python projects running globally, ensuring you stay productive and compliant.
Rate Limits: VK’s API caps requests (e.g., 3 per second, 5,000 daily). Exceeding this triggers errors or bans. Solution? Add delays:
import time
import vk_api
vk_session = vk_api.VkApi(token='your_token')
vk = vk_session.get_api()
for i in range(10):
posts = vk.wall.get(owner_id='-123456', count=10, offset=i*10)
print(f"Fetched batch {i+1}")
time.sleep(0.34) # Stay under 3 requests/second
This paces API calls safely. For web scraping, delays like `time.sleep(random.uniform(1, 3))` mimic human behavior.
Dynamic Content: VK’s infinite scroll loads posts via JavaScript, invisible to Requests. Use Selenium to scroll and capture data:
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
driver = webdriver.Chrome()
driver.get('https://vk.com/public123456')
for _ in range(3):
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
time.sleep(2) # Wait for load
posts = driver.find_elements(By.CLASS_NAME, 'post_text')
for post in posts[:5]:
print(f"Post: {post.text[:50]}...")
driver.quit()
This scrolls three times, grabbing visible posts. Update class names via browser inspection, as VK’s HTML evolves.
CAPTCHAs: Heavy scraping triggers VK’s CAPTCHA walls. Avoid them with proxies and user-agent rotation:
import requests
from itertools import cycle
proxies = ['http://proxy1:port', 'http://proxy2:port']
proxy_pool = cycle(proxies)
user_agents = ['Mozilla/5.0 (Windows NT 10.0; Win64; x64) Chrome/91.0.4472.124', '...']
ua_pool = cycle(user_agents)
url = 'https://vk.com/public123456'
for _ in range(5):
proxy = next(proxy_pool)
headers = {'User-Agent': next(ua_pool)}
response = requests.get(url, headers=headers, proxies={'http': proxy})
print(f"Status: {response.status_code}")
Use services like Bright Data or Smartproxy for reliable proxies. Free proxies are risky—stick to paid ones for stability.
IP Bans: VK blocks IPs after excessive requests. Beyond proxies, respect limits and monitor usage. A 2024 scraping survey by WebScrapingAPI found 68% of bans stem from missing delays. Log requests to track patterns:
import logging
logging.basicConfig(filename='scrape.log', level=logging.INFO)
logging.info('Fetching posts...')
posts = vk.wall.get(owner_id='-123456', count=10)
logging.info('Fetch complete.')
Logs help diagnose ban triggers. If banned, switch IPs and pause scraping for 24 hours.
Data Inconsistencies: VK’s API occasionally returns partial data (e.g., missing attachments). Cross-check with web scraping or retry calls. For example, verify post counts:
posts = vk.wall.get(owner_id='-123456', count=10)
if posts['count'] < 10:
print("Warning: Incomplete data. Retrying...")
time.sleep(1)
posts = vk.wall.get(owner_id='-123456', count=10)
These fixes keep your scraper robust. Test small batches before scaling, and always store backups—Pandas to CSV or SQLite works well.
With these solutions, you’re equipped to handle VK’s quirks, ensuring your projects thrive.
Frequently Asked Questions
Is it legal to scrape VK with Python?
Scraping public data within VK’s terms and local laws is generally allowed. Use the API for safety and avoid private information.
What’s the best library for VK scraping?
It varies—vk_api excels for API access, Selenium for dynamic pages. Combine tools based on your project’s needs.
How do I avoid getting banned while scraping VK?
Add delays, rotate IPs, and respect API limits. Mimic human behavior to stay under VK’s radar.
Can I scrape VK without an API token?
Yes, with tools like BeautifulSoup for public pages, but it’s riskier. Use delays and proxies to minimize bans.
How do I handle VK’s CAPTCHAs?
Use proxies and rotate user agents. Paid proxy services reduce CAPTCHA triggers compared to free options.
Conclusion
Scraping VK with Python is more than a technical skill—it’s a strategic gateway to one of the world’s most vibrant social platforms. From public posts to multimedia trends, VK’s data fuels innovation, research, and business growth. But success hinges on blending expertise with ethics. By using Python’s tools thoughtfully—vk_api for compliance, Selenium for depth, proxies for stability—you unlock insights while respecting VK’s rules and user privacy.
This guide aimed to empower professionals globally, offering a roadmap from setup to advanced techniques. Whether you’re analyzing gaming communities or tracking e-commerce trends, these methods adapt to your goals. The key? Start small, test often, and prioritize responsibility. Scraping VK isn’t just about data—it’s about building smarter, more informed projects that stand the test of time.
Keep exploring VK’s possibilities. Update your scripts as the platform evolves, and share your findings with the community. Python and VK together offer endless potential—use it wisely.
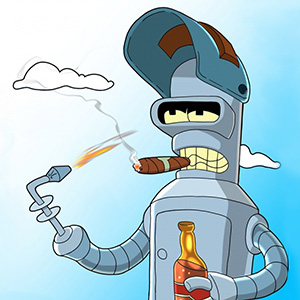
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.