Scraping Reddit Posts with Python: A Comprehensive Guide
Introduction
In today’s data-driven world, Reddit Scraping has become a powerful tool for professionals seeking to extract valuable insights from one of the internet’s largest communities. Reddit, often called the “front page of the internet,” hosts millions of posts across thousands of subreddits, making it a goldmine for market researchers, data analysts, and developers. Whether you’re tracking trends, analyzing sentiments, or gathering user feedback, scraping Reddit posts with Python offers a flexible and efficient solution. This guide equips you with the knowledge and tools to perform Reddit Scraping effectively, tailored for professionals worldwide.
Python’s simplicity and robust ecosystem make it the go-to choice for web scraping tasks. With libraries like PRAW and Scrapy, you can automate data collection, navigate Reddit’s API, and handle large datasets with ease. However, scraping Reddit comes with challenges, such as rate limits, ethical considerations, and dynamic content formats. This article addresses these hurdles, providing actionable techniques and best practices to ensure your scraping projects are both successful and compliant. From setting up your environment to deploying scalable solutions, we’ll cover everything you need to know.
Why is Reddit Scraping so valuable? According to a 2023 report by Statista, Reddit boasts over 1.5 billion monthly active users, generating vast amounts of user-generated content. Extracting this data can unlock insights into consumer behavior, emerging trends, and niche communities. Whether you’re a marketer analyzing brand sentiment or a researcher studying social dynamics, this guide offers practical examples and expert tips to elevate your work. Let’s explore the tools and techniques to master Reddit Scraping with Python.
Scraping Reddit is not just about collecting data; it’s about understanding the pulse of online communities. Python’s versatility allows you to tailor your scraping approach to specific goals, whether you’re targeting text posts, comments, or upvotes. This guide assumes basic familiarity with Python but caters to both beginners and advanced users by breaking down complex concepts. We’ll dive into the ethical implications of scraping, ensuring you respect Reddit’s terms of service and user privacy. By the end, you’ll have a clear roadmap to build robust scraping pipelines.
The global appeal of Reddit makes it a unique platform for cross-cultural analysis. From subreddits like r/technology to r/food, the diversity of topics allows professionals to explore virtually any industry or interest. Python’s open-source libraries, combined with Reddit’s accessible API, democratize data access for teams worldwide. This article also addresses common pain points, such as handling authentication errors or parsing nested comment threads, with step-by-step solutions. Our goal is to empower you to scrape Reddit efficiently while adhering to best practices.
Ready to unlock the potential of Reddit data? This guide provides a comprehensive toolkit, from basic setups to advanced techniques like sentiment analysis and data visualization. We’ll include code snippets, comparison tables, and real-world case studies to make the learning process hands-on. Whether you’re based in the US, EU, or Asia, the strategies here are designed for universal applicability. Let’s dive into the world of Reddit Scraping with Python and transform raw data into actionable insights.
Why Python for Reddit Scraping
Python is the preferred language for Reddit Scraping due to its simplicity, extensive library ecosystem, and community support. Its readability makes it accessible for beginners, while its powerful libraries like PRAW (Python Reddit API Wrapper) and Scrapy cater to advanced users. According to a 2024 Stack Overflow survey, 68% of developers use Python for web scraping tasks, highlighting its dominance in the field. Python’s cross-platform compatibility ensures your scraping scripts work seamlessly across Windows, macOS, and Linux.
Another advantage is Python’s ability to handle large datasets efficiently. Libraries like pandas and NumPy enable data cleaning and analysis, transforming raw Reddit posts into structured formats. Python’s asynchronous capabilities, via libraries like aiohttp, allow for faster scraping of multiple subreddits simultaneously. Additionally, Python’s open-source nature means you can access cutting-edge tools without licensing costs, making it ideal for professionals worldwide.
Python’s integration with Reddit’s API is a game-changer. PRAW simplifies authentication and data retrieval, allowing you to fetch posts, comments, and user profiles with minimal code. For those bypassing the API, libraries like BeautifulSoup enable HTML parsing for custom scraping needs. Python’s versatility also extends to error handling, ensuring your scripts remain robust against network issues or API rate limits. This flexibility is crucial for scaling projects from small experiments to enterprise-level solutions.
Global professionals benefit from Python’s extensive documentation and community forums. Whether you’re troubleshooting a bug or seeking optimization tips, platforms like Stack Overflow and GitHub offer solutions tailored to Reddit Scraping. Python’s ecosystem also supports integration with databases like PostgreSQL or MongoDB, enabling seamless storage of scraped data. By choosing Python, you’re investing in a tool that grows with your scraping ambitions.
Essential Python Libraries and Tools
To perform Reddit Scraping, you’ll need a suite of Python libraries tailored to different aspects of the process. Below is a table comparing key tools, followed by a detailed breakdown of their roles.
Library/Tool | Purpose | Pros | Cons |
---|---|---|---|
PRAW | Reddit API interaction | Official Reddit support, easy to use | Rate limits apply |
Scrapy | Web scraping framework | High performance, scalable | Steeper learning curve |
BeautifulSoup | HTML parsing | Simple syntax, great for small projects | Slower for large-scale scraping |
pandas | Data manipulation | Powerful data analysis, CSV/JSON export | Memory-intensive for large datasets |
PRAW is the cornerstone of Reddit Scraping, providing a Pythonic interface to Reddit’s API. It handles authentication, rate limiting, and data retrieval, making it ideal for fetching posts and comments. PRAW’s official documentation offers comprehensive guides for setup and usage.
Scrapy excels in large-scale scraping projects, allowing you to crawl Reddit pages without relying on the API. It’s particularly useful for extracting data from dynamic content or archived posts. BeautifulSoup complements Scrapy for simpler HTML parsing tasks, while pandas organizes scraped data into DataFrames for analysis.
Here’s a basic PRAW setup to fetch posts from a subreddit:
import praw
reddit = praw.Reddit(
client_id="your_client_id",
client_secret="your_client_secret",
user_agent="my_reddit_scraper"
)
subreddit = reddit.subreddit("python")
for post in subreddit.top(limit=10):
print(post.title, post.score)
Install these libraries using pip: pip install praw scrapy beautifulsoup4 pandas
. Always store API credentials securely to avoid unauthorized access.
Common Challenges and Formats in Reddit Scraping
Scraping Reddit presents unique challenges, from API rate limits to parsing diverse content formats. Reddit’s API enforces a 600-request-per-10-minute limit per authenticated user, requiring careful request management. Dynamic content, such as lazy-loaded comments, complicates non-API scraping, while Reddit’s robots.txt restricts certain endpoints. Ethical considerations, like respecting user privacy, are also critical.
Reddit posts vary in format—text, images, videos, or links—each requiring specific parsing logic. Comments are nested, often spanning multiple threads, which demands recursive algorithms to capture. Below are common challenges and solutions:
- Rate Limits: Use PRAW’s built-in rate limiter or implement exponential backoff with Scrapy.
- Nested Comments: Parse comment trees using recursive functions or PRAW’s comment forest.
- Dynamic Content: Use Selenium for browser automation when Scrapy alone isn’t enough.
Handling these challenges ensures your scraping process is robust and efficient, regardless of subreddit complexity.
Advanced Reddit Scraping Techniques
Once you’ve mastered basic scraping, advanced techniques like sentiment analysis, data visualization, and asynchronous scraping can elevate your projects. Sentiment analysis, using libraries like NLTK or TextBlob, helps gauge user opinions in subreddit discussions. For example, analyzing r/investing can reveal market sentiments.
Asynchronous scraping with aiohttp speeds up data collection across multiple subreddits. Here’s an example of asynchronous post fetching:
import aiohttp
import asyncio
import json
async def fetch_subreddit(session, subreddit):
url = f"https://www.reddit.com/r/{subreddit}/top.json?limit=10"
async with session.get(url) as response:
return await response.json()
async def main():
async with aiohttp.ClientSession() as session:
tasks = [fetch_subreddit(session, sub) for sub in ["python", "dataisbeautiful"]]
results = await asyncio.gather(*tasks)
for result in results:
print(result["data"]["children"][0]["data"]["title"])
asyncio.run(main())
Data visualization with Matplotlib or Seaborn can highlight trends in scraped data, such as post frequency or upvote distributions. These techniques are particularly valuable for global professionals seeking actionable insights.
Best Practices for Scalability
Scalable Reddit Scraping requires careful planning to handle large datasets and comply with Reddit’s policies. Below are key best practices:
- Respect Rate Limits: Use PRAW’s rate limiter and monitor API quotas.
- Store Data Efficiently: Save scraped data to databases like MongoDB for quick retrieval.
- Automate Monitoring: Implement logging to track errors and performance.
- Ethically Scrape: Avoid overloading Reddit’s servers and respect user privacy.
Containerizing your scraping scripts with Docker ensures portability across environments, ideal for teams in the US, EU, or Asia. Regular updates to your scripts, based on Reddit’s API changes, maintain long-term reliability.
Real-World Applications of Reddit Scraping
Reddit Scraping has diverse applications across industries. Marketers use it to monitor brand sentiment, as seen in a 2023 case study where a tech company analyzed r/gadgets to refine product launches. Researchers leverage Reddit data to study social trends, such as political polarization in r/politics. Developers build bots that aggregate subreddit content for newsletters or dashboards.
In Asia, e-commerce firms scrape r/deals to identify trending products, while EU-based analysts track r/europe for policy discussions. These applications demonstrate Reddit’s global relevance and Python’s role in unlocking its potential.
Frequently Asked Questions
What is Reddit Scraping?
Reddit Scraping involves extracting data from Reddit posts, comments, or user profiles using tools like Python’s PRAW or Scrapy. It’s used for market research, sentiment analysis, and trend tracking.
Which Python library is best for Reddit Scraping?
PRAW is ideal for API-based scraping due to its simplicity and official Reddit support. Scrapy is better for large-scale, non-API projects requiring custom crawling logic.
Is Reddit Scraping legal?
Scraping Reddit is legal if you adhere to its API terms and robots.txt. Always respect rate limits, user privacy, and Reddit’s policies to ensure ethical scraping.
Conclusion
Scraping Reddit posts with Python unlocks a wealth of opportunities for professionals worldwide. From tracking consumer trends to analyzing niche communities, Reddit Scraping empowers you to transform raw data into actionable insights. This guide has equipped you with the tools, techniques, and best practices to navigate Reddit’s API, overcome challenges, and scale your projects effectively. By leveraging Python’s robust ecosystem, you can tailor your scraping approach to diverse goals, whether you’re in the US, EU, or Asia.
Start by setting up PRAW for simple subreddit scraping, then explore advanced techniques like sentiment analysis or asynchronous data collection. Always prioritize ethical scraping to respect Reddit’s community and policies. Apply these strategies in your next project, and share your insights with colleagues or online forums to contribute to the global data community. Your journey into Reddit Scraping begins now—dive in and discover the power of Reddit’s data!
The versatility of Reddit Scraping makes it a skill worth mastering. Python’s libraries, combined with Reddit’s open API, provide a foundation for projects of any scale. Whether you’re building a sentiment dashboard or aggregating niche content, the techniques here are designed for long-term relevance. Regular updates to your scripts, based on Reddit’s evolving API, will keep your workflows robust. Consider joining communities like r/learnpython to stay informed about new tools and trends.
Global professionals can adapt these methods to their local contexts. For example, EU-based analysts might focus on GDPR-compliant scraping, while Asian marketers could target region-specific subreddits. The key is to align your scraping goals with ethical and technical best practices. By doing so, you’ll not only achieve your objectives but also contribute to a responsible data ecosystem. Take the first step today—experiment with the code snippets provided and see the results for yourself.
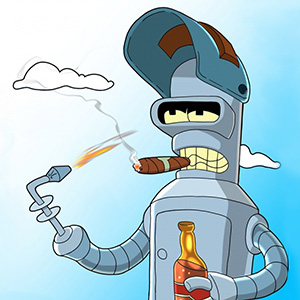
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.