Scraping Data Python: A Practical Guide to Mastering Web Data Extraction
Web data is everywhere—think product prices, social media trends, or even weather forecasts. Extracting it efficiently can unlock insights, automate workflows, or fuel your next big project. That’s where scraping data with Python comes in: a powerful, flexible way to gather info from the internet without breaking a sweat. This guide dives deep into how you can use Python to scrape data like a pro, avoid common pitfalls, and keep your code clean and legal.
Whether you’re a developer looking to streamline data collection or an analyst hunting for actionable insights, you’ll find actionable tips here. We’ll cover tools, techniques, and real-world examples—no fluff, just stuff that works.
Why Python for Scraping Data?
Python’s dominance in scraping data isn’t random. Its simplicity lets you write readable code fast, while a rich ecosystem of libraries—like BeautifulSoup, Scrapy, and Requests—handles the heavy lifting. Need to parse HTML? There’s a tool for that. Want to scale up to thousands of pages? Python’s got you covered.
Compared to alternatives like JavaScript or R, Python strikes a balance: it’s beginner-friendly yet robust enough for enterprise-level tasks. Plus, its community is massive—Stack Overflow is packed with solutions to any scraping snag you might hit. For web scraping with Python, you’re not just picking a language; you’re tapping into a toolkit built for the job.
Essential Tools for Web Scraping
Before you start, you need the right gear. Here’s what powers most data scraping projects in Python:
- Requests: Fetches web pages with minimal fuss. Think of it as your browser’s “open URL” button.
- BeautifulSoup: Parses HTML and XML, turning messy code into something you can navigate. Perfect for beginners.
- Scrapy: A full-fledged framework for large-scale scraping. It’s fast, handles retries, and manages multiple requests like a champ.
- Selenium: Drives a real browser, ideal for dynamic sites heavy on JavaScript (e.g., infinite scroll pages).
- Pandas: Not a scraper, but a lifesaver for structuring scraped data into tables or CSVs.
Each tool has its vibe. For quick scripts, Requests and BeautifulSoup are your go-to. For big projects—like scraping an e-commerce site—Scrapy or Selenium might save the day. Pick based on your goal: speed, simplicity, or scale.
How to Scrape Data with Python: Step-by-Step
Let’s get hands-on. Here’s a practical walkthrough to scrape a simple site—say, a list of book titles and prices from a mock bookstore. We’ll use Requests and BeautifulSoup for this one.
Step 1: Set Up Your Environment
Install the basics:
pip install requests beautifulsoup4
Got Python 3.8+? You’re golden.
Step 2: Fetch the Page
Start by grabbing the HTML:
import requests
url = "https://example-bookstore.com/books"
response = requests.get(url)
html_content = response.text
Check response.status_code
—200 means success. Anything else? Trouble’s brewing.
Step 3: Parse the Data
Now, let BeautifulSoup do its magic:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, "html.parser")
books = soup.find_all("div", class_="book-item")
Here, find_all
hunts for <div>
tags with a book-item
class. Adjust based on the site’s structure—use your browser’s “Inspect” tool to peek at the HTML.
Step 4: Extract What You Need
Loop through and grab titles and prices:
for book in books:
title = book.find("h2").text.strip()
price = book.find("span", class_="price").text.strip()
print(f"Title: {title}, Price: {price}")
This spits out something like: Title: Python 101, Price: $29.99
.
Step 5: Save It
Dump it into a CSV with Pandas:
import pandas as pd
data = [{"Title": book.find("h2").text.strip(), "Price": book.find("span", class_="price").text.strip()} for book in books]
df = pd.DataFrame(data)
df.to_csv("books.csv", index=False)
Boom—your data’s ready for analysis.
This is scraping data 101. For trickier sites, tweak the approach—more on that later.
Handling Challenges in Data Scraping
Scraping isn’t always smooth sailing. Websites fight back, and tech hiccups happen. Here’s how to tackle the big ones.
Dynamic Content
JavaScript-heavy pages won’t load fully with Requests alone. Enter Selenium:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://dynamic-site.com")
html = driver.page_source
soup = BeautifulSoup(html, "html.parser")
It’s slower but gets the job done. Pro tip: run it headless (no GUI) for speed.
Anti-Scraping Measures
Blocked by a CAPTCHA or IP ban? Slow down your requests with time.sleep(2)
between hits. Use proxies or rotate user agents:
headers = {"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) Chrome/91.0.4472.124"}
response = requests.get(url, headers=headers)
Tools like cloudscraper
can bypass Cloudflare protection too.
Messy HTML
Nested tags or broken markup? BeautifulSoup’s forgiving, but double-check your selectors. If find_all
fails, try select
with CSS selectors: soup.select(".book-item h2")
.
Best Practices for Efficient Scraping
Want clean, fast, reliable results? Stick to these:
- Respect Robots.txt: Check the site’s rules (e.g.,
example.com/robots.txt
). It’s not law, but it’s polite. - Throttle Requests: Space out hits to avoid overwhelming servers—think 1–2 seconds between calls.
- Log Errors: Wrap code in
try/except
blocks and log failures to debug later. - Cache Data: Save raw HTML locally to avoid re-fetching during testing.
- Scale Smart: For big jobs, use Scrapy’s built-in concurrency or split tasks across threads.
Efficiency isn’t just speed—it’s about not getting banned and keeping your data usable.
Legal and Ethical Considerations
Scraping’s powerful, but it’s a gray area. Public data’s usually fair game, but terms of service matter. Scraping Twitter? Their API’s stricter than their site suggests. Private data or copyrighted content? Steer clear unless you’ve got permission.
Ethically, don’t slam servers with requests—be a good netizen. If you’re unsure, ask yourself: “Would I be okay with someone scraping my site like this?” When in doubt, consult a legal pro.
Conclusion: Level Up Your Scraping Game
Scraping data with Python isn’t just a skill—it’s a superpower for unlocking the web’s secrets. From quick scripts with BeautifulSoup to industrial-strength crawlers with Scrapy, you’ve got options to fit any project. The trick? Match your tools to the task, dodge the roadblocks, and keep it ethical.
What sets great scrapers apart isn’t just code—it’s curiosity. Dig into the HTML, experiment with selectors, and tweak your approach. The web’s a messy, evolving beast, and mastering it takes practice. So grab a site, fire up your IDE, and start pulling data. What will you uncover?
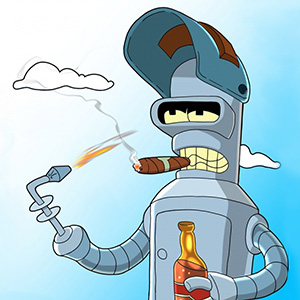
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.