Request Parsing process
Request parsing is the process of breaking down an incoming request to a web server into parts that can be analyzed and processed. This allows the server to understand the intent of the request and generate an appropriate response.
Overview of Request Parsing
When a request comes in to a web server, it consists of different components that need to be examined. The two main parts are:
- The request line
- Request headers
Additionally, the request may contain:
- Cookies
- URL parameters
- POST data
By parsing out these different elements, the web server can determine crucial information like:
- The HTTP method being used (GET, POST, etc)
- The endpoint being requested
- Headers that provide additional context
- Any cookies or data being sent along
This all allows the server to handle the request properly by routing it, extracting parameters, authenticating the user, etc. Request parsing is the critical first step that makes web applications functional.
Components of Request Parsing
Let’s look at the key components that need to be parsed from an incoming HTTP request:
Request Line
The request line is the first line of the request which contains vital information. A typical request line would look like:
GET /users/123 HTTP/1.1
Here the server can parse out the HTTP method (GET), the endpoint (/users/123) and protocol version (HTTP/1.1). This indicates what kind of request this is and how to interpret it.
Headers
Headers provide additional metadata about the request such as:
- Host – Domain or host being requested
- User-Agent – Client software making the request
- Accept – Acceptable response formats
- Authorization – Credentials for authentication
Headers contain a lot of useful context that can influence how the request is handled.
Cookies
Cookies are sent along with requests as a way to maintain session state. The server parses these out to identify the current user session.
URL Parameters
For GET requests, any query parameters appended to the URL need to be parsed out. These provide extra input data for GET requests.
POST Data
For POST requests, any form data or JSON payload sent in the body needs to be parsed out to retrieve submitted parameters.
Parsing the Request in Web Frameworks
Most web development frameworks and servers handle request parsing automatically. For example:
- In Express.js, the
req
object provides access to URL, query params, body, etc - In Django, the
HttpRequest
object parses everything from the request - PHP frameworks parse data into
$_GET
,$_POST
, etc
So request parsing is done under the hood. But it’s still important to understand what’s happening when that request comes into the server.
Why Request Parsing Matters
Request parsing seems simple, but is critical to building functional web applications. Without parsing:
- Servers wouldn’t know how to route requests properly
- Parameters, headers, and other data would get lost
- Security vulnerabilities like CRLF injection can occur
Careful request parsing also allows tracking important metrics about requests like endpoint usage, response times, traffic volume, etc.
So while request parsing happens invisibly, it powers much of the functionality and observability of web applications. It transforms a raw request into structured data that can drive logic and business value.
Conclusion
Request parsing extracts meaningful information from raw HTTP requests. It allows separating out key components like the method, endpoint, headers, and body data. This powers routing, security, and monitoring in web apps and APIs. Understanding it helps developers build more robust services and unlock capabilities provided by the request data.
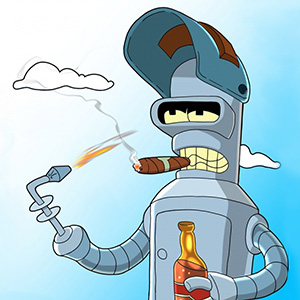
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.