Request Parsing
Decoding requests is a crucial step in server-side programming. When a request hits an endpoint, the server needs to break it down to grasp what is being asked of it. This entails examining the request components like method, endpoint, headers, cookies, payload etc. to derive significance. Unless requests are parsed thoroughly, the server cannot comprehend what clients want nor respond fittingly.
There are several approaches to request parsing:
Leveraging frameworks – Most frameworks like Express, Django etc. include built-in parsing methods, extracting request details for developers.
Manual parsing – Servers can directly parse raw request data, extracting bits like method, headers manually to form request objects. More control but laborious.
Middleware – Middleware placed before routes can parse requests e.g. body-parser in Express. Parsed data is made available to routes.
Modules – Some servers like Apache have modules that analyze requests. Provides parsing at the server level.
Certain request elements need focused parsing:
Method – GET, POST etc. Dictates request nature.
URL – Endpoint and parameters being queried.
Headers – Metadata like content types.
Cookies – Sent back by clients.
Body – Data in body like forms, JSON.
Uploads – File uploads in body.
Parsing challenges:
URL extraction – Path and query strings need separation.
Headers parsing – No standard format, needs careful handling.
Bodies – JSON, XML, forms etc. need specialized parsers.
Multipart – Complex parsing for files and forms.
Streaming – Chunked data makes parsing tricky.
Security – Malicious data can trigger vulnerabilities.
Best practices:
Validate methods, headers, URLs against injection.
Use size limits, timeouts for large requests.
Leverage solid frameworks and libraries.
Use well-tested modules like Node’s querystring.
Offload parsing to middleware.
Rate limit to avoid parser overload.
Stream parsing for large requests.
Thoroughly test edge cases.
Careful parsing is imperative for robust servers. Frameworks and libraries simplify much of it. For custom parsers, tight security is critical – sanitize inputs, validate thoroughly and test rigorously. Well-designed parsing logic results in reliable and secure servers.
Methods for Parsing Requests
There are a few common approaches employed for accurately parsing incoming requests:
-
Using a web framework – Most web frameworks like Express.js for Node.js or Django for Python come with built-in methods to easily parse requests. The framework extracts all the required information and makes it available for the application to work with.
-
Manual parsing – Servers can parse requests manually by directly working with the raw request data. This involves looking at the request string, extracting the method, headers, etc. and constructing request objects to represent this information. This gives fine-grained control but requires more effort.
-
Using middleware – Middleware can be used to parse requests before they reach route handlers. For example, in Express body-parser middleware can be used to parse request bodies. The parsed body is then available to routes.
-
Web server modules – Some web servers like Apache httpd have modules like mod_rewrite that can parse and manipulate request information. These provide request parsing abilities at the web server level.
Important Parts of a Request
Some key parts of an HTTP request that need to be extracted and analyzed:
-
Method – GET, POST, PUT etc. Defines the nature of the request.
-
URL – The endpoint being requested and any query parameters.
-
Headers – Contain metadata for the request like content type.
-
Cookies – Cookies stored by the client that need to be sent back.
-
Body – Data like form fields or JSON that may be sent in the request body.
-
Uploads – File uploads are often included in request bodies.
Challenges with Parsing
There are some common challenges faced when parsing HTTP requests:
-
Extracting URLs – The URL contains the path and query string which need to be separately extracted.
-
Parsing headers – Headers do not follow a standard format and need to be carefully parsed.
-
Handling bodies – Request bodies can be in many different formats like JSON, XML or forms. Each needs specialized parsing.
-
Multpart bodies – Requests can have multipart bodies with files and form data. They require multipart parsing.
-
Streaming data – Request data can be streamed chunk-by-chunk making parsing more complex.
-
Security issues – Requests may contain malicious data and vulnerabilities can arise during parsing. Strict validation and sanitization is necessary.
Best Practices
To ensure robust and secure request parsing, some best practices should be followed:
-
Validate request method, headers, URLs to prevent vulnerabilities like CRLF injection.
-
Use size limits and timeouts to manage unconstrained requests.
-
Employ a good web framework or library to handle most parsing automatically.
-
For custom parsing code, use tried and tested modules like Node’s
querystring
module. -
Use middleware to handle parsing of bodies, cookies, uploads etc.
-
Implement rate limiting to prevent overwhelming the parser.
-
Stream parsing can help manage large requests and uploads.
-
Test parsers thoroughly for edge cases using automated tests.
Conclusion
Careful request parsing is needed to build robust server applications. Using appropriate frameworks and tools can ease much of the parsing process. For custom parsers, following security best practices is essential. Proper validation, sanitization and testing ensures requests are handled correctly. Implementing request parsing thoughtfully will lead to servers that function reliably and securely.
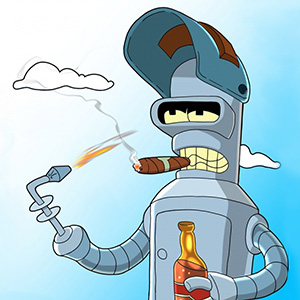
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.