Advanced Regular Expression Techniques: Master Text Parsing
Overview
While basic regular expressions are powerful, mastering Advanced Regular Expression Techniques can transform your ability to parse and manipulate text. These methods tackle complex patterns, improve efficiency, and handle edge cases. Below, we explore key concepts with examples to take your regex skills to the next level.
1. Lookahead and Lookbehind Assertions
Lookahead and lookbehind assertions let you check for patterns before or after your match without including them in the result—perfect for conditional matching.
- Positive Lookahead
(?=...)
: Ensures a pattern follows.import re text = "100 dollars 200 euros" matches = re.findall(r'\d+(?=\s*dollars)', text) print(matches) # Output: ['100']
Matches numbers followed by “dollars.”
- Negative Lookahead
(?!...)
: Ensures a pattern does not follow.matches = re.findall(r'\d+(?!\s*dollars)', text) print(matches) # Output: ['200']
Matches numbers not followed by “dollars.”
2. Non-Capturing Groups
Non-capturing groups (?:...)
group patterns without capturing them, ideal for applying quantifiers cleanly.
text = "hello world hello universe"
matches = re.findall(r'(?:hello\s)+(\w+)', text)
print(matches) # Output: ['world', 'universe']
Matches words after one or more “hello ” without capturing the prefix.
3. Named Capture Groups
Named capture groups (?P<name>...)
assign names to groups, improving code readability and access.
text = "John Doe, Jane Smith"
matches = re.finditer(r'(?P<first>\w+)\s(?P<last>\w+)', text)
for match in matches:
print(f"First: {match.group('first')}, Last: {match.group('last')}")
# Output: First: John, Last: Doe
# First: Jane, Last: Smith
Extracts first and last names with named references.
4. Conditional Patterns
Conditional patterns (?(condition)true-pattern|false-pattern)
adapt matching based on prior conditions.
text = "123-456-7890 (123) 456-7890"
pattern = r'(\()?\d{3}(?(1)\)|-)\d{3}-\d{4}'
matches = re.findall(pattern, text)
print(matches) # Output: ['', '(']
Matches phone numbers with or without parentheses, adjusting separators.
5. Greedy vs. Non-Greedy Matching
Quantifiers like *
are greedy by default, but adding ?
makes them non-greedy, matching minimally.
text = "contentmore content"
greedy = re.findall(r'.*', text)
non_greedy = re.findall(r'.*?', text)
print(greedy) # Output: ['contentmore content']
print(non_greedy) # Output: ['content', 'more content']
Non-greedy matching splits tags individually.
6. Unicode and Multilingual Support
Regex supports Unicode with \p{}
, perfect for multilingual text processing.
text = "Café 北京"
matches = re.findall(r'\p{L}+', text, re.UNICODE)
print(matches) # Output: ['Café', '北京']
Matches words in any language using Unicode letters.
7. Recursive Patterns
Recursive patterns (?R)
match nested structures like parentheses or tags.
text = "(1 + (2 * (3 + 4)))"
pattern = r'\(([^()]+|(?R))*\)'
matches = re.findall(pattern, text)
print(matches) # Output: ['1 + (2 * (3 + 4))']
Captures content within balanced parentheses.
8. Verbose Mode
Verbose mode with re.VERBOSE
makes complex regex readable with comments and spacing.
pattern = r'''
^ # Start of string
[A-Za-z0-9._%+-]+ # Local part
@ # At symbol
[A-Za-z0-9.-]+ # Domain
\.[A-Z|a-z]{2,}$ # TLD
matches = re.findall(pattern, "support@example.com", re.VERBOSE)
print(matches) # Output: ['support@example.com']
Validates email addresses with clarity.
9. Backreferences
Backreferences \1
reuse captured groups within the pattern.
text = "hello hello world world"
matches = re.findall(r'(\b\w+\b)\s\1', text)
print(matches) # Output: ['hello', 'world']
Finds repeated words.
10. Atomic Groups
Atomic groups (?>...)
prevent backtracking, boosting performance.
text = "aaaaab"
pattern = r'(?>a+)ab'
matches = re.findall(pattern, text)
print(matches) # Output: []
No match occurs as backtracking is disabled.
Conclusion
Advanced regular expression techniques unlock powerful text processing capabilities. From lookahead regex to recursive patterns, these methods handle complex scenarios, optimize performance, and enhance maintainability. Experiment with these in Python to become a regex expert, tackling tasks from data validation to multilingual parsing with confidence.
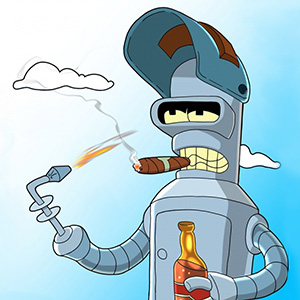
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.