Python Scripts: Unlocking the Power of Automation
The Essence of Python Scripting
Python scripting has revolutionized the way developers and programmers approach task automation. These versatile programs, crafted using the Python programming language, empower users to streamline repetitive tasks, manipulate data, and interact with system resources effortlessly. By harnessing the power of Python’s extensive libraries and intuitive syntax, programmers can create robust solutions to complex problems with remarkable efficiency.
- The Essence of Python Scripting
- Crafting Your First Python Script
- Essential Components of Python Scripts
- Leveraging Python’s Standard Library
- Automating File Operations with Python Scripts
- Web Scraping with Python Scripts
- Data Processing and Analysis Scripts
- Enhancing Scripts with External Libraries
- Best Practices for Python Script Development
- Debugging and Troubleshooting Python Scripts
- Conclusion: The Transformative Power of Python Scripts
The allure of Python scripts lies in their ability to simplify intricate processes, allowing developers to focus on solving high-level challenges rather than getting bogged down in low-level implementation details. This efficiency boost has made Python a go-to language for a wide array of applications, from web development and data analysis to artificial intelligence and scientific computing.
Crafting Your First Python Script
Embarking on your Python scripting journey begins with understanding the fundamental building blocks of a script. At its core, a Python script is a text file containing a sequence of Python statements that execute in order. To create your inaugural script, follow these steps:
- Open a text editor of your choice, such as Visual Studio Code, PyCharm, or even a simple notepad application.
- Begin your script with a shebang line (#!/usr/bin/env python3) if you’re working on a Unix-like system. This line ensures the script runs with the correct Python interpreter.
- Import any necessary modules or libraries that your script will utilize.
- Write your Python code, organizing it into functions and classes as needed for clarity and reusability.
- Save the file with a .py extension, which identifies it as a Python script.
Remember, the key to crafting effective scripts lies in writing clean, well-documented code that others (including your future self) can easily understand and maintain.
Essential Components of Python Scripts
A well-structured Python script typically incorporates several key components that enhance its functionality and readability. These elements include:
- Import statements: Place these at the top of your script to bring in external modules and libraries that extend Python’s capabilities.
- Function definitions: Break your code into logical, reusable units by defining functions that perform specific tasks.
- Main execution block: Use the
if __name__ == "__main__":
idiom to control which code runs when the script is executed directly versus when it’s imported as a module. - Error handling: Implement try-except blocks to gracefully manage potential exceptions and provide informative error messages.
- Command-line arguments: Utilize the
argparse
module to allow users to pass parameters to your script, enhancing its flexibility.
By incorporating these components, you’ll create scripts that are not only functional but also maintainable and adaptable to various use cases.
Leveraging Python’s Standard Library
Python’s standard library is a treasure trove of modules that can significantly enhance your scripting capabilities. These built-in modules provide a wide range of functionalities without the need for external dependencies. Some indispensable modules for script writing include:
- os: For interacting with the operating system, manipulating file paths, and executing system commands.
- sys: For accessing interpreter-specific parameters and functions.
- datetime: For working with dates, times, and time intervals.
- json: For parsing and creating JSON data, which is crucial for web-based scripts and data interchange.
- csv: For reading and writing CSV files, a common format for data storage and exchange.
- re: For working with regular expressions, enabling powerful text processing and pattern matching.
Familiarizing yourself with these modules will dramatically increase your scripting prowess and enable you to tackle a wider range of automation tasks efficiently.
Automating File Operations with Python Scripts
One of the most common applications of Python scripts is automating file operations. Whether you need to organize files, process large datasets, or perform batch operations on multiple files, Python provides powerful tools to streamline these tasks. Here’s an example of a script that organizes files based on their extension:
import os
import shutil
def organize_files(directory):
for filename in os.listdir(directory):
if os.path.isfile(os.path.join(directory, filename)):
file_extension = filename.split('.')[-1]
destination_folder = os.path.join(directory, file_extension)
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
shutil.move(os.path.join(directory, filename), os.path.join(destination_folder, filename))
# Usage
organize_files('/path/to/your/directory')
This script demonstrates how Python can effortlessly handle file operations, making it an invaluable tool for system administrators and data analysts alike.
Web Scraping with Python Scripts
Python’s versatility shines in web scraping tasks, where scripts can extract valuable data from websites. Utilizing libraries like requests
for making HTTP requests and BeautifulSoup
for parsing HTML content, developers can create powerful scrapers to gather information automatically. Here’s a basic example of a web scraping script:
import requests
from bs4 import BeautifulSoup
def scrape_website(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract all paragraph texts
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.text)
# Usage
scrape_website('https://example.com')
This script demonstrates the fundamental concept of web scraping, which can be expanded to collect specific data points, navigate through multiple pages, or even interact with web forms.
Data Processing and Analysis Scripts
Python’s robust ecosystem of data processing libraries, such as pandas and NumPy, makes it an excellent choice for creating data analysis scripts. These scripts can handle large datasets, perform complex calculations, and generate insightful visualizations. Here’s a simple example using pandas to analyze a CSV file:
import pandas as pd
import matplotlib.pyplot as plt
def analyze_sales_data(file_path):
df = pd.read_csv(file_path)
# Calculate total sales by product
sales_by_product = df.groupby('Product')['Sales'].sum().sort_values(descending=True)
# Plot the results
sales_by_product.plot(kind='bar')
plt.title('Total Sales by Product')
plt.xlabel('Product')
plt.ylabel('Total Sales')
plt.tight_layout()
plt.show()
# Usage
analyze_sales_data('sales_data.csv')
This script showcases how Python can effortlessly load, process, and visualize data, making it an invaluable tool for data analysts and business intelligence professionals.
Enhancing Scripts with External Libraries
While Python’s standard library is extensive, external libraries can further amplify your scripting capabilities. Popular libraries like requests
for HTTP operations, pandas
for data manipulation, and pytest
for testing can significantly enhance your scripts’ functionality. To incorporate these libraries, use pip, Python’s package installer:
pip install requests pandas pytest
After installation, you can import these libraries in your scripts, opening up a world of advanced features and optimized performance.
Best Practices for Python Script Development
To create maintainable and efficient Python scripts, adhere to these best practices:
- Follow PEP 8 guidelines for consistent code style.
- Write modular code by breaking functionality into small, reusable functions.
- Use meaningful variable and function names that clearly convey their purpose.
- Implement proper error handling to make your scripts robust and user-friendly.
- Add comments and docstrings to explain complex logic and function purposes.
- Utilize version control systems like Git to track changes and collaborate with others.
- Implement logging to aid in debugging and monitoring script execution.
- Write unit tests to ensure your script functions correctly as you make changes.
By adhering to these practices, you’ll create scripts that are not only functional but also maintainable and scalable.
Debugging and Troubleshooting Python Scripts
Effective debugging is crucial for developing reliable Python scripts. Utilize Python’s built-in pdb
module for interactive debugging, or leverage IDE features for step-by-step execution. Implementing logging with the logging
module can provide valuable insights into script execution:
import logging
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
def complex_function(x, y):
logging.debug(f"Inputs: x={x}, y={y}")
result = x * y
logging.info(f"Result calculated: {result}")
return result
# Usage
complex_function(5, 3)
This approach allows you to track the flow of your script and quickly identify issues when they arise.
Conclusion: The Transformative Power of Python Scripts
Python scripts have emerged as a cornerstone of modern software development and automation. Their versatility, coupled with Python’s readability and vast ecosystem of libraries, enables developers to craft solutions for an extensive range of problems with remarkable efficiency. From automating mundane tasks to processing complex datasets and building sophisticated web scrapers, Python scripts empower programmers to achieve more with less code.
As you delve deeper into the world of Python scripting, remember that the journey of mastery is ongoing. Continually explore new libraries, experiment with different scripting techniques, and stay abreast of the latest Python developments. By doing so, you’ll not only enhance your programming skills but also unlock new possibilities for innovation and problem-solving in your personal and professional endeavors.
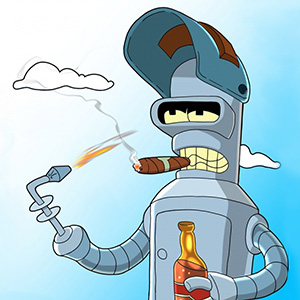
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.