Parsing Protection
Parsing protection refers to various methods and techniques utilized to prevent web scraping and automated data extraction from websites and web applications. As information on the internet becomes increasingly valuable, many companies aim to protect their data assets from being copied or accessed without authorization. Implementing robust parsing protection is crucial for maintaining control over proprietary data sets and ensuring compliance with data regulations.
This article provides an overview of common parsing protection mechanisms, their purpose, and how they function to block scrapers and bots. We will also discuss some of the motivations behind scraping activity and the importance of balancing security with user experience. By the end, readers will have a foundational understanding of key concepts and leading practices in implementing parsing protection.
Motivations Behind Web Scraping
Before exploring techniques to prevent scraping, it is helpful to understand why scraping occurs in the first place. Here are some of the most common reasons:
-
Competitive intelligence – Companies may scrape competitor sites to collect pricing data, product info, or other market intelligence. This can provide strategic insights.
-
Research and journalism – Web scraping enables researchers and journalists to rapidly gather large amounts of data for analysis. Proper citations are always required.
-
Price monitoring – Apps and services scrape ecommerce sites to track price changes and inform consumers when prices drop.
-
Aggregation – Travel sites scrape airline and hotel sites to display comparative listings in one place for users.
There are certainly legitimate and legal use cases for web scraping. However, indiscriminate, large-scale scraping can violate a company’s terms of service and present security or compliance risks, highlighting the need for robust parsing protection.
Technical Approaches to Parsing Protection
There are a variety of technical methods sites can implement to identify bots and automated scraping activity and prevent such efforts from succeeding. Here are some leading approaches:
Blocking Known Scrapers
-
Maintain lists of IP addresses known to be associated with scrapers to automatically block requests from those sources.
-
Identify and block specific user agents that are commonly associated with scrapers.
-
Ban proxies, VPNs, and data centers often used by scrapers to mask origins.
Analysis of Access Patterns
-
Profile typical human user behavior patterns such as pages visited, actions taken, mouse movements, etc.
-
Detect access patterns that diverge from the norm and appear bot-like such as unusually high traffic volume, fast speeds between pages, or crawling instead of visiting deep pages.
-
Implement progressive response delays or CAPTCHAs when suspicious patterns are observed to deter bots.
Obfuscation and Scrambling
-
Dynamically generate DOM elements and scramble ID attributes to make site structure less predictable.
-
Implement session-specific tokens that must be present to access certain data assets.
-
Leverage cryptographic techniques to obfuscate and frequently rotate predictability-reducing values.
Legal Terms of Service
-
Implement clear Terms of Service that prohibit automated scraping without permission.
-
Pursue legal action against aggregators or commercial entities that violate terms at scale.
-
Require free API access or formal data licensing so scraping is not necessary.
Balancing Parsing Protection with User Experience
While robust parsing protection is crucial, it’s important not to implement these measures in a way that excessively degrades performance or usability for legitimate human users. Here are some tips for balancing security and user experience:
-
Phase in detection mechanisms gradually and selectively rather than all at once.
-
Focus blocking on large-scale, systematic bot activity vs one-offscrapers.
-
Allow exceptions for research institutions and journalists through an access request process.
-
Do not impose CAPTCHAs, delays, or other friction without sufficient confidence of bot activity.
-
Provide easy to access and affordable data licensing options as an alternative to scraping.
-
Monitor site analytics for changes in bounce rates, conversion funnel drop off, or other indicators of usability issues.
Conclusion
As the internet economy continues to grow, effective parsing protection is essential to secure proprietary business data. By leveraging a layered combination of technical mechanisms ranging from IP blocks to traffic pattern analysis and obfuscation techniques, companies can significantly curtail unauthorized scraping. However, these measures require thoughtful implementation that minimizes negative impacts to legitimate users. The guidelines provided offer a starting point for organizations seeking to strike the right balance.
With a nuanced strategy grounded in monitoring scraper innovations and continuous security improvements, companies can stay ahead of the data extraction curve while also delivering excellent customer experiences. The parsing protection landscape will continue to evolve in parallel with advances in scraping tactics, ensuring this cat-and-mouse game remains an important point of emphasis for years to come.
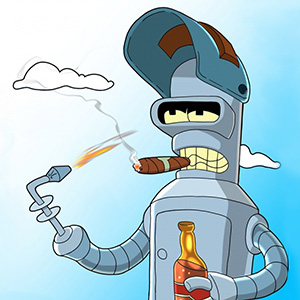
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.