Mastering 5 Key Secrets of Parsing Modules for Smarter Code
Developers and enthusiasts diving into the world of code often stumble upon a hidden gem: parsing modules. These powerful tools transform raw data—be it text, JSON, or sprawling XML—into structured, actionable insights, saving hours of manual labor. Whether you’re crafting a web scraper, debugging a data pipeline, or just tinkering with a side project, understanding how these modules work is a game-changer. This article unpacks expert strategies, hands-on examples, and practical tips tailored for those hungry to sharpen their skills.
Parsing isn’t just about splitting strings; it’s about bending complexity to your will. From hobbyists experimenting with personal projects to professionals optimizing enterprise systems, mastering this skill unlocks efficiency and precision. Expect no fluff—only actionable advice grounded in real-world experience, spiced with examples to spark your creativity. Let’s dive into what makes these tools tick and how you can wield them like a pro.
What Are Parsing Modules, Really?
At their core, parsing modules are libraries or frameworks that dissect input data into manageable pieces. Think of them as translators fluent in chaos, turning messy strings into neat arrays, objects, or trees. Python’s BeautifulSoup
, JavaScript’s Cheerio
, or C++’s Boost.Spirit
—each offers a unique flavor of this magic. They’re indispensable when raw data refuses to play nice, whether it’s a tangled HTML page or a log file screaming for structure.
Beyond splitting text, these tools handle syntax validation, error detection, and structure enforcement. Ever tried manually extracting tags from a 500-line HTML file? It’s a slog—error-prone and exhausting. A parsing module does it in seconds, catching edge cases like mismatched tags or rogue characters you’d miss. For developers and enthusiasts alike, this means less time wrestling with regex and more time building features that matter.
Take a real example: parsing a podcast RSS feed. With xml.etree.ElementTree
in Python, you can extract episode titles, dates, and URLs in a dozen lines. Manually? You’d be knee-deep in string slicing, praying you didn’t miss a closing tag. That’s the power of these tools—efficiency wrapped in simplicity.
- Key Benefit: Automates repetitive data structuring tasks.
- Alternative Solutions: Regex or manual loops—slower but viable for tiny datasets.
- Pro Tip: Pair with a debugger to spot parsing hiccups early.
Why Parsing Modules Beat Manual Methods Every Time
Picture this: you’re handed a 10,000-line CSV file riddled with inconsistencies—extra commas, missing headers, odd quotes. Manual parsing with loops and conditionals might work, but it’s a slog—error-prone, time-sucking, and soul-crushing. Enter parsing modules, slicing through the noise with surgical precision. They’re built to handle scale, complexity, and the unexpected, leaving DIY approaches choking on dust.
Speed isn’t the only perk. These tools offer built-in error handling, reducing those “where did I mess up?” moments. Python’s csv
module doesn’t just read rows; it flags malformed entries without breaking a sweat. For enthusiasts tweaking side projects or pros managing big data, this reliability is pure gold. Imagine processing sales data—manual methods might skip a row; a parser catches it and keeps rolling.
Here’s a quick example: parsing a log file from a server. Without a module, you’re splitting lines, checking timestamps, and hoping no field sneaks in uninvited. With awk
or Python’s linecache
paired with a parser, you extract IPs, errors, and times in minutes. The difference? One’s a chore; the other’s a breeze.
5 Must-Know Tips to Leverage Parsing Modules Like a Pro
Tip 1: Match the Module to Your Data Type
Not all parsing tools are created equal. XML demands heavyweights like lxml
, while JSON shines with lightweight champs like json.parse
. Picking the right one saves memory, boosts speed, and spares you headaches. Mismatched tools? You’re courting crashes, sluggish performance, or worse—parsed gibberish.
For example, scraping a website? BeautifulSoup
paired with requests
is your dream team. Processing a log file? awk
or a custom parser might edge out. Knowing your data’s quirks—nested structures, encoding issues, or funky delimiters—guides the choice. Experiment, tweak, and watch efficiency soar.
Real-world case: extracting headlines from a news site. Using BeautifulSoup
, you target <h2>
tags with a single call—done in 10 lines. Try that with regex, and you’re still debugging pattern matches an hour later. Another scenario: parsing a YAML config file. Python’s PyYAML
handles nested keys effortlessly, while a manual approach drowns in indentation woes.
Tip 2: Handle Errors Without Losing Your Mind
Data is messy—fact of life. Missing tags, broken delimiters, rogue characters—parsing modules catch these, but only if you plan ahead. Wrap your code in try-catch blocks or lean on built-in validation. Python’s xml.etree.ElementTree
, for instance, flags malformed XML before it derails your script, saving you from silent failures.
A real-world fix: logging errors instead of crashing. One developer I know saved a week-long job by catching a single unescaped quote in a JSON feed—json.JSONDecodeError
pointed it right out. Anticipating chaos isn’t paranoia; it’s strategy. Your sanity (and deadlines) will thank you.
Example time: parsing a user-uploaded CSV. Some rows have extra commas; others miss fields. Using csv.DictReader
, you set defaults for missing keys and log oddities—clean output, no fuss. Contrast that with manual parsing: you’d be sifting through stack traces, cursing silently. Error handling isn’t optional—it’s your lifeline.
Tip 3: Optimize for Speed with Streaming
Huge files don’t play nice with memory-hogging parsers. Streaming parsers—like sax
for XML—process data in chunks, keeping resource use low. It’s like sipping from a straw instead of chugging a gallon jug. Perfect for big datasets or low-RAM environments, streaming turns impossible tasks into cakewalks.
Node.js developers swear by stream-json
for massive JSON files. It’s not just fast; it’s scalable. Pair it with async operations, and you’ve got a lean, mean parsing machine. Test it on a gigabyte-sized log—you’ll feel the difference when your laptop doesn’t melt.
Practical scenario: parsing a 2GB server log. Loading it all with json.load
? Crash city. Switch to stream-json
, and you process entries line-by-line, extracting errors or IPs without breaking a sweat. Another use: streaming XML from an API—sax
grabs elements as they arrive, no waiting for the full download.
Tip 4: Debug with Visual Tools
Stuck on a parsing snag? Tools like JSON Formatter or jq
for command-line fans turn gibberish into clarity. Seeing your data’s structure visually cuts debugging time in half. No more guessing where that nested array broke or why that tag vanished.
Pros often lean on IDE extensions too. PyCharm’s data viewer, for instance, maps parsed output live—hover over a variable, see the tree. It’s less about fancy gadgets and more about spotting patterns fast. Add this to your toolkit, and parsing headaches shrink to mere blips.
Example: debugging a JSON API response. The output’s a mess—nested objects galore. Paste it into JSON Formatter, and boom: collapsible sections reveal a missing key. Or use jq
in terminal—jq '.data[] | .name'
pulls names instantly. Compare that to console logging and squinting—night and day.
Tip 5: Test Edge Cases Relentlessly
Your parser works on clean data? Great. Now throw it a curveball—empty files, corrupt inputs, weird encodings. Robust parsing modules shine when tested hard. A hobbyist scraping forums once found his script died on emoji-heavy posts—until he stress-tested with Unicode and patched the gap.
Build a test suite. Use libraries like pytest
or mocha
to automate it. Cover the obvious (valid JSON) and the ugly (malformed CSV with extra commas). The payoff? Code that doesn’t buckle under pressure, whether it’s a weekend hack or a production pipeline.
Case study: parsing IoT sensor data. Normal JSON? Smooth sailing. But toss in a null byte or a truncated file, and it’s chaos—unless you test. With pytest
, you simulate bad inputs: empty strings, half-parsed arrays, garbled UTF-8. Result? A parser that laughs at adversity.
How to Achieve Flawless Data Extraction with Parsing Modules
Extraction isn’t just parsing—it’s parsing with intent. Say you’re pulling product prices from an e-commerce site. A module like Cheerio
lets you target DOM elements with CSS selectors—$('.price').text()
—skipping the noise. Precision here beats brute-force string slicing any day, delivering clean data fast.
Start by mapping your data’s structure. Sketch the hierarchy—tags, keys, delimiters—then code accordingly. One trick: chain parsers for multi-format files (e.g., JSON inside HTML). It’s like peeling an onion, layer by layer, until you hit the good stuff—structured, usable output.
Example walkthrough: scraping a blog. You fetch HTML with axios
, then use Cheerio
to grab <article>
tags. Inside each, a JSON blob hides in a <script>
tag—parse it with JSON.parse
. Two parsers, one goal: titles, dates, and metadata in a tidy array. Manual? You’d be lost in tag soup.
Tool | Best For | Key Feature | Example Use |
---|---|---|---|
BeautifulSoup | HTML Scraping | Tag navigation | Extracting forum posts |
csv module | Tabular Data | Error flagging | Parsing sales reports |
sax | Large XML | Streaming | Processing API feeds |
Advanced Parsing: Beyond the Basics
Ready to level up? Custom parsers—built with tools like Boost.Spirit — tackle niche formats no off-the-shelf module handles. Think proprietary log files, quirky APIs, or that one-off binary dump. It’s more work upfront, but the control is unmatched—tailor-made parsing perfection.
Another gem: parallel parsing for massive datasets. Libraries like multiprocessing
in Python split the load across cores. A data engineer once halved processing time on a 50GB dump this way—chunks parsed simultaneously, results merged seamlessly. Combine with streaming, and you’re cooking with gas—fast, efficient, and scalable.
Deep dive example: parsing a custom game log. The format’s odd—timestamps, player IDs, and actions in a semi-colon mess. Boost.Spirit
lets you define a grammar: time >> ';' >> id >> ';' >> action
. Run it, and you’ve got a structured dataset. Off-the-shelf tools? They’d choke. Parallelize it across 10 cores, and a 100MB file parses in seconds.
Conclusion: Parsing as an Art Form
Parsing modules aren’t just tools—they’re a mindset. They teach patience, precision, and a knack for taming the wildest data. What separates the good from the great isn’t raw skill; it’s curiosity—poking at edge cases, tweaking performance, finding joy in the puzzle. Next time you face a tangled dataset, don’t groan—grab a module and carve out something brilliant.
Reflect on this: every line parsed is a problem solved, a story uncovered. Whether you’re a hobbyist chasing a passion project or a pro wrangling enterprise chaos, these insights shift the game. The real win? Knowing you’ve got the chops to bend code—and data—to your vision, turning raw bytes into beauty.
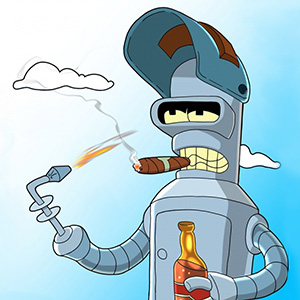
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.