7 Proven Steps to Master Parsing Data with Python Like a Pro
Introduction: Why Parsing Data Matters for Enthusiasts
For hobbyists and coding enthusiasts, diving into data analysis opens a world of possibilities—whether it’s scraping websites, processing files, or wrangling messy datasets. Parsing data with Python stands out as a critical skill, blending creativity with technical precision. This article unravels expert insights and actionable techniques, tailored for those eager to transform raw information into meaningful results. From beginners tinkering with scripts to seasoned coders refining their craft, mastering this process promises efficiency and empowerment.
Data parsing isn’t just about reading files; it’s about unlocking hidden patterns and solving real-world problems. With Python’s versatility, you’re equipped to tackle diverse formats—CSV, JSON, XML, or even unstructured text. Ready to elevate your skills? Let’s explore seven proven steps, peppered with practical tips and tools, to help you excel.
Step 1: Understand Your Data’s Structure
Before writing a single line of code, dissect your data’s anatomy. Is it a neatly organized CSV or a chaotic HTML page? Knowing the structure shapes your approach. For hobbyists, this step is like studying a map before a treasure hunt—skip it, and you’re lost.
Start by inspecting the file manually. Open it in a text editor or browser to spot headers, delimiters, or tags. Python’s built-in open()
function lets you peek at the first few lines programmatically. Pair it with print()
to preview without overwhelm. Understanding whether your data is tabular, hierarchical, or freeform dictates your parsing strategy.
- Tools to Use: Notepad++, VS Code, or Python’s
head
-like snippet:with open('data.csv', 'r') as file:
print(file.read(100)) # First 100 characters - Key Tip: Look for patterns—commas, tabs, or nested brackets—to identify the format.
Step 2: Pick the Right Python Libraries
Python shines because of its libraries, turning complex tasks into simple scripts. Choosing the right one depends on your data type. For enthusiasts, experimenting with these tools builds confidence and speeds up projects.
Consider these options:
- CSV: Use
csv
for lightweight, comma-separated files. It’s built-in and beginner-friendly. - JSON: Leverage
json
for structured, nested data—perfect for APIs or config files. - XML/HTML: Try
BeautifulSoup
(withrequests
) for web scraping or XML parsing. Install viapip install beautifulsoup4
. - Unstructured Text: Opt for
re
(regular expressions) when patterns get tricky.
For example, parsing a CSV looks like this:
import csv
with open('data.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
print(row['column_name'])
Mix and match libraries as needed—flexibility is Python’s strength.
Step 3: Handle Messy Data with Grace
Real-world data is rarely pristine. Missing values, inconsistent formats, or encoding errors can derail your script. Hobbyists often learn this the hard way, but cleaning data is half the parsing battle.
Start with error handling. Wrap file operations in try-except
blocks to catch issues like FileNotFoundError
. For encoding woes (think UTF-8 vs. Latin-1), specify the encoding in open()
:
with open('data.txt', 'r', encoding='utf-8') as file:
content = file.read()
Next, normalize data. Strip whitespace with .strip()
, convert strings to numbers with float()
or int()
, and handle nulls with conditionals. Libraries like pandas
(install via pip install pandas
) simplify this:
import pandas as pd
df = pd.read_csv('data.csv').fillna(0) # Replace NaN with 0
Step 4: Extract What You Need
Parsing isn’t about grabbing everything—it’s about pinpointing what matters. Whether it’s specific columns or keywords, filtering saves time and memory. For enthusiasts, this step feels like panning for gold.
With tabular data, use list comprehensions or pandas
slicing:
import pandas as pd
df = pd.read_csv('data.csv')
key_data = df[df['age'] > 25]['name'].tolist()
For text, regular expressions shine. Extract emails with:
import re
text = "Contact: alice@example.com, bob@domain.com"
emails = re.findall(r'[\w\.-]+@[\w\.-]+', text)
Pro tip: Test patterns on regex101.com—a DoFollow gem for regex learners.
Step 5: Transform Data into Usable Formats
Raw data rarely fits your needs straight away. Transforming it—say, into dictionaries, lists, or even visualizations—makes it actionable. This is where hobbyists turn data into projects.
Convert parsed JSON into a Python dictionary:
import json
with open('data.json', 'r') as file:
data = json.load(file)
print(data['key'])
Or pivot CSV data with pandas
:
df = pd.read_csv('data.csv')
pivot_table = df.pivot(index='date', columns='category', values='sales')
Experiment with outputs—save to Excel, plot with matplotlib
, or prep for a database. Flexibility fuels creativity.
Step 6: Automate and Scale Your Parsing
Why parse once when you can automate? Enthusiasts love efficiency, and Python’s scripting power delivers. Build reusable functions or scripts to handle recurring tasks.
Wrap your logic in a function:
def parse_file(filename, format='csv'):
if format == 'csv':
with open(filename, 'r') as file:
return list(csv.DictReader(file))
elif format == 'json':
with open(filename, 'r') as file:
return json.load(file)
data = parse_file('data.csv')
Scale up with loops or multiprocessing for big datasets. Libraries like concurrent.futures
speed up batch processing—ideal for parsing multiple files.
Step 7: Validate and Debug Like an Expert
Even pros make mistakes. Validating your output ensures accuracy, while debugging sharpens your skills. For hobbyists, this step separates amateurs from experts.
Check data integrity—does the row count match expectations? Use assertions:
data = parse_file('data.csv')
assert len(data) > 0, "Empty dataset detected!"
Debug with print()
statements or Python’s pdb
module. For larger projects, log issues with the logging
module:
import logging
logging.basicConfig(level=logging.INFO)
logging.info("Parsing started...")
Mistakes teach more than successes—embrace them.
Practical Example: Parsing a Webpage
Let’s tie it together with a real example: scraping a webpage. Using BeautifulSoup
, fetch and parse HTML:
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
titles = soup.find_all('h2')
for title in titles:
print(title.text.strip())
Image Description: Screenshot of Python code parsing HTML, with alt text: “Code snippet showing parsing data with Python using BeautifulSoup.”
This snippet grabs all <h2>
tags—tweak it for your needs. Explore BeautifulSoup’s docs for advanced tricks (DoFollow).
Key Benefits of Mastering Data Parsing
Why invest time in this? Beyond the thrill of coding, parsing unlocks:
- Efficiency: Automate repetitive tasks.
- Insight: Reveal trends in messy data.
- Versatility: Apply skills to web scraping, file processing, or APIs.
Alternative solutions—like GUI tools—exist, but Python offers unmatched control. Hobbyists gain a superpower: turning chaos into order.
Conclusion: Parsing as a Creative Edge
Parsing data with Python isn’t just a technical chore—it’s a canvas for creativity. Each dataset hides a story, and these seven steps equip you to tell it. From dissecting structures to automating workflows, you’ve got the tools to bend data to your will. What sets enthusiasts apart? It’s not just parsing—it’s the curiosity to ask, “What’s next?” So, grab a dataset, fire up your editor, and see where the code takes you. The journey’s as rewarding as the destination.
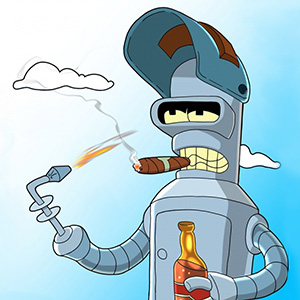
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.