Parsing CSV
Delving into the world of data, Comma-Separated Values (CSV) is a term frequently encountered. This familiar format, named for its delineation of data points, desirably delivers datasets in a simple, human-readable form. Herein lies an exploration of the process of parsing CSV files, a procedure which sometimes appears tricky but can ultimately be made to unfold smoothly and intuitively.
Understanding the concept fully is vital for data professionals and program developers. So, with an authoritative tone and nuanced understanding of the topic, we now delve into the heart of the matter.
Dissecting CSV Data
The CSV format assembles data as structured text, with comma marking the margins between individual data points, hence the notation ‘Comma-Separated Values.’ Ample information is neatly laid out in rows and columns, akin to spreadsheet data.
CSV Files – The Basics
CSV files are essentially plain text files with a .csv extension, a benchmark for data exchange due to their simplicity and broad compatibility, notably with spreadsheet applications.
Components of CSV
CSV files are composed of various elements:
- Records: Each line in a CSV file corresponds to a different record.
- Fields:Individual data values in records are delineated by commas.
- Delimiter: A character, often a comma, used to segregate fields.
Parsing CSV— Step by Step Illustration
Parsing CSV files involves reading and processing these files to convert their content into a usable format. Let us enunciate the main phase divisions involved in this intriguing procedure.
Step 1: Read the File
The primary step requires opening and reading the CSV file always ensures closing the file after completion. This standard procedure is universally applicable across different programming languages.
Step 2: Parse the File
The most critical piece of the puzzle lies in parsing the contents of the file. Delimiters are the basis for parsing or breaking down the data into discrete elements. In essence, parsing turns a series of text strings into tactile bits of data.
Step 3: Iterate through the Data
Having divided the file into digestible parts, the next stage involves looping or iterating through the data. The resulting data array can then be utilized for various data manipulation tasks.
Step 4: Output the Data
This final phase hinges on the desired result of parsing. Data could be written to a new file, passed to a function, or visually represented in a graph.
Note: Handling Errors
Errors can occur during parsing. It is crucial to include error-handling provisions in your code, as these inconsistencies can lead to parsing interruptions, compromising the integrity of the outcome.
Handy Tools and Libraries
Common programming languages have in-built libraries and functions to facilitate CSV parsing; Python’s ‘csv’ or Node.js’s ‘csv-parser’ for example, so check them out!
Conclusion
In this world of ever-growing data, the ability to parse CSV files is a powerful toolkit addition for any data professional or developer. This guide, a comprehensive breakdown of CSV parsing, is intended to assist beginners and professionals alike in mastering this task.
Parsing CSV may initially seem intimidating, but approaching it step by step, being aware of common problems and their solutions, will help navigate this journey effectively. Mastery takes practice, and parsing CSV files is no exception!
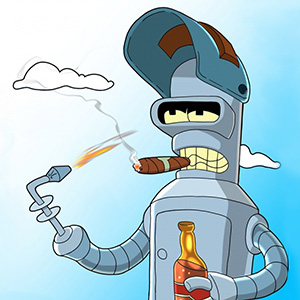
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.