Parsing Bot for Telegram
Understanding Parsing Bots
A parsing bot is a specialized software application designed to extract specific data from web pages or other online sources. These bots leverage advanced algorithms and techniques to navigate through websites, identify relevant information, and collect it in a structured format. In the context of Telegram, a messaging app renowned for its robust bot ecosystem, parsing bots have emerged as powerful tools for data acquisition and automation.
Advantages of Parsing Bots on Telegram
Efficient Data Collection
Parsing bots streamline the process of gathering data from various online sources, saving users significant time and effort. By automating the extraction process, these bots can quickly collect and organize large volumes of information, which would otherwise be a tedious and time-consuming task if performed manually.
Access to Real-Time Updates
Many parsing bots are designed to continuously monitor specific websites or data sources, ensuring that users have access to the latest updates and information. This real-time data acquisition capability is particularly valuable in industries or applications where timely information is crucial, such as news, financial markets, or e-commerce.
Customizable and Versatile
Telegram’s bot ecosystem allows for the development of highly customizable parsing bots tailored to specific user needs. These bots can be programmed to extract data based on predefined parameters, filters, or keywords, ensuring that users receive only the most relevant information.
Key Features of Parsing Bots on Telegram
Web Scraping Capabilities
At the core of parsing bots is their web scraping functionality, which enables them to navigate through websites and extract data from HTML or other structured formats. These bots can be programmed to recognize specific patterns, tags, or data structures, making it possible to precisely target and collect the desired information.
Data Parsing and Restructuring
Once the data is extracted, parsing bots employ advanced parsing algorithms to restructure and organize the information into a more user-friendly format. This can include formatting the data into tables, lists, or other structured representations, making it easier for users to analyze and interpret the collected information.
Scheduled Updates and Notifications
Many parsing bots offer the ability to schedule data extraction tasks or set up notifications for specific events or updates. Users can configure the bot to periodically check for new information or receive alerts when certain conditions are met, ensuring they stay up-to-date with the latest developments.
Potential Applications of Parsing Bots on Telegram
- Price Monitoring: Track and compare prices across multiple e-commerce platforms, enabling users to find the best deals.
- News Aggregation: Collect and curate news articles from various sources based on specific topics or keywords.
- Job Listings: Extract and organize job postings from multiple job boards or career websites.
- Social Media Monitoring: Monitor social media platforms for mentions, hashtags, or specific keywords relevant to a brand or industry.
- Research and Analysis: Gather data from academic databases, journals, or online repositories for research purposes.
Conclusion
Parsing bots on Telegram provide a powerful and versatile solution for automated data extraction and organization. By leveraging advanced web scraping and parsing techniques, these bots enable users to efficiently collect and structure information from various online sources. As the demand for real-time data continues to grow, parsing bots will play an increasingly important role in streamlining information gathering processes across diverse industries and applications.
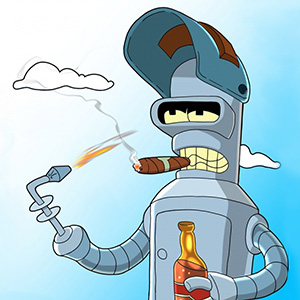
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.