10 Must-Know Techniques for Mastering Page Parsing with Python
Introduction to Page Parsing with Python
For professionals looking to extract data from websites efficiently, page parsing with Python offers a powerful solution. Whether you’re a data analyst scraping market trends, a developer building automation tools, or a researcher gathering insights, this guide dives into actionable techniques tailored to your needs. Python’s versatility and robust ecosystem make it a go-to choice for transforming raw HTML into structured data, saving time and unlocking opportunities across industries.
This article explores everything from foundational tools to advanced strategies, ensuring you can tackle real-world projects with confidence. Expect practical tips, code snippets, and insights that cater to global professionals, adaptable to any region’s unique demands. Let’s unlock the potential of web data together.
Why Python Excels for Page Parsing
Python stands out for page parsing due to its simplicity and extensive library support. Its readable syntax allows professionals to focus on logic rather than wrestling with code, making it ideal for both beginners and seasoned coders. Libraries like BeautifulSoup and Scrapy streamline the process, turning complex web structures into manageable datasets with minimal effort.
Beyond ease of use, Python’s community-driven ecosystem ensures constant updates and solutions to emerging challenges. According to a 2023 Stack Overflow survey, Python ranks as the most popular language for data-related tasks, with over 60% of developers favoring it for its flexibility. This makes it a reliable choice for parsing tasks worldwide, no matter the scale or complexity.
Essential Tools and Libraries
To succeed in page parsing with Python, you need the right tools. Here’s a breakdown of the most effective libraries professionals rely on, each offering unique strengths for different scenarios.
These tools simplify everything from basic HTML extraction to large-scale web scraping. Let’s explore them in detail.
Library | Purpose | Best For |
---|---|---|
BeautifulSoup | Parsing HTML and XML documents | Small to medium projects, quick prototyping |
Scrapy | Full-scale web crawling and scraping | Large datasets, complex websites |
Requests | Fetching web pages | Simple HTTP requests |
lxml | Fast HTML/XML processing | Performance-critical tasks |
Selenium | Dynamic content parsing | JavaScript-heavy sites |
Description of an image: A screenshot of Python code using BeautifulSoup to parse a webpage, highlighting extracted data in a terminal window. Alt text: “Python code parsing a webpage with BeautifulSoup, displaying results globally.”
Core Techniques for Effective Parsing
Mastering page parsing with Python requires a mix of foundational and advanced techniques. Below are the must-know methods to extract data efficiently, tailored for professionals seeking practical solutions.
1. Fetching Pages with Requests
The first step in parsing is retrieving the webpage. The Requests library makes this straightforward. Send a GET request, check the status, and you’re ready to parse the HTML content.
import requests
response = requests.get('https://example.com')
if response.status_code == 200:
html_content = response.text
This method is lightweight and perfect for static pages. Pair it with a parser for the next step.
2. Parsing with BeautifulSoup
BeautifulSoup turns messy HTML into a navigable structure. Use it to target specific tags, classes, or IDs. Here’s how to extract all links from a page:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
links = [a['href'] for a in soup.find_all('a', href=True)]
It’s intuitive and handles poorly formatted code well, making it a favorite for quick tasks.
3. Handling Dynamic Content with Selenium
For pages loaded with JavaScript, Selenium simulates a browser. It’s slower but essential for dynamic sites.
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
html = driver.page_source
driver.quit()
Use this when BeautifulSoup alone can’t access rendered content.
4. Scaling with Scrapy
Scrapy shines for large-scale projects. Define a spider to crawl multiple pages and extract structured data efficiently.
import scrapy
class MySpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
yield {'title': response.css('h1::text').get()}
It’s robust and built for speed, ideal for professionals managing big datasets.
5. API Scraping with Requests
Many sites offer APIs for data access. Use Requests to fetch JSON directly, bypassing HTML parsing when possible.
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json()
for item in data['items']:
print(item['name'])
This is faster and cleaner for structured data, though it requires API documentation or reverse-engineering.
6. Precision with XPath and lxml
For speed and precision, lxml with XPath targets elements directly. It’s less forgiving than BeautifulSoup but blazing fast.
from lxml import html
page = html.fromstring(html_content)
titles = page.xpath('//h2/text()')
Use this for performance-critical tasks or when CSS selectors aren’t enough.
Practical Examples and Use Cases
Let’s put these techniques into action with detailed examples. These scenarios show how page parsing with Python solves professional challenges globally.
Example 1: Scraping Product Prices (Step-by-Step)
Imagine tracking e-commerce prices. Here’s a detailed breakdown:
- Step 1: Fetch the Page – Use Requests to get the HTML.
- Step 2: Parse with BeautifulSoup – Identify product containers.
- Step 3: Extract Data – Pull names and prices.
- Step 4: Output Results – Print or save the data.
import requests
from bs4 import BeautifulSoup
response = requests.get('https://example-shop.com/products')
soup = BeautifulSoup(response.text, 'html.parser')
products = soup.select('.product')
for product in products:
name = product.find('h2').text.strip()
price = product.find('.price').text.strip()
print(f'{name}: {price}')
This is perfect for market analysis. Add error handling for robustness.
Example 2: Extracting News Headlines (Step-by-Step)
For a media tool, grab headlines with this process:
- Step 1: Request the Site – Get the raw HTML.
- Step 2: Parse Structure – Use BeautifulSoup to find headlines.
- Step 3: Clean Data – Strip excess whitespace.
import requests
from bs4 import BeautifulSoup
response = requests.get('https://example-news.com')
soup = BeautifulSoup(response.text, 'html.parser')
headlines = [h.text.strip() for h in soup.find_all('h3', class_='headline')]
for headline in headlines:
print(headline)
Simple yet effective for aggregating content worldwide.
Example 3: Crawling a Blog with Scrapy
To collect blog titles, Scrapy scales effortlessly:
import scrapy
class BlogSpider(scrapy.Spider):
name = 'blog'
start_urls = ['https://example-blog.com']
def parse(self, response):
for title in response.css('.post-title::text'):
yield {'title': title.get()}
next_page = response.css('a.next::attr(href)').get()
if next_page:
yield response.follow(next_page, self.parse)
Run with scrapy crawl blog -o output.json
to save results.
Example 4: Social Media Scraping (Twitter-like Platform)
For sentiment analysis, scrape posts from a social platform:
- Step 1: Simulate Browser – Use Selenium for dynamic content.
- Step 2: Scroll Page – Load more posts.
- Step 3: Extract Posts – Parse with BeautifulSoup.
from selenium import webdriver
from bs4 import BeautifulSoup
driver = webdriver.Chrome()
driver.get('https://example-social.com')
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
html = driver.page_source
soup = BeautifulSoup(html, 'html.parser')
posts = [p.text for p in soup.select('.post-content')]
driver.quit()
print(posts)
This handles JavaScript-heavy sites, critical for social media data.
Overcoming Common Challenges
Page parsing isn’t without hurdles. Here’s how to tackle frequent issues.
1. Blocked Requests
Websites may block scrapers. Use headers:
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get('https://example.com', headers=headers)
Proxies or delays help further.
2. Dynamic Content
For JavaScript-loaded data, use Selenium or find API endpoints via browser tools.
Balance speed and completeness based on your needs.
3. Data Inconsistency
Layouts change. Add error handling:
try:
price = soup.find('.price').text
except AttributeError:
price = 'N/A'
This ensures reliability.
Legal and Ethical Considerations
Parsing web data comes with responsibilities. Here’s what professionals need to know.
First, check a site’s robots.txt
. It signals what’s off-limits. Ignoring it isn’t illegal but may violate terms of service, risking bans or legal action. Second, respect copyright—scraped content isn’t yours to republish without permission.
Ethically, consider impact. Heavy scraping can overload servers, so limit requests. A 2022 study by Webhose found 15% of sites block aggressive scrapers, highlighting the need for moderation. Use public APIs when available, and always attribute data sources where required.
Optimizing Your Parsing Workflow
Efficiency matters. Here’s how to streamline your process.
1. Multithreading
Speed up fetching:
from concurrent.futures import ThreadPoolExecutor
urls = ['https://example1.com', 'https://example2.com']
def fetch(url):
return requests.get(url).text
with ThreadPoolExecutor() as executor:
results = list(executor.map(fetch, urls))
Cuts time on multi-page tasks.
2. Caching Results
Avoid redundant requests:
import requests_cache
requests_cache.install_cache('parse_cache', expire_after=3600)
response = requests.get('https://example.com')
Saves bandwidth and time.
3. Regular Expressions
Refine text extraction:
import re
text = 'Price: $19.99'
price = re.search(r'\$\d+\.\d{2}', text).group()
Boosts precision.
4. Profiling with cProfile
Identify bottlenecks:
import cProfile
def parse_page():
response = requests.get('https://example.com')
soup = BeautifulSoup(response.text, 'html.parser')
cProfile.run('parse_page()')
Optimize slow sections based on output.
5. Cloud Integration with AWS Lambda
Scale parsing with serverless computing:
import requests
def lambda_handler(event, context):
url = event['url']
response = requests.get(url)
return {'status': response.status_code, 'content': response.text}
Deploy on AWS Lambda for distributed tasks.
FAQ
What is page parsing in Python?
Page parsing in Python involves extracting and processing data from web pages using libraries like BeautifulSoup or Scrapy to turn HTML into usable information.
Which library is best for beginners?
BeautifulSoup is beginner-friendly due to its simplicity and clear documentation, making it perfect for starting out globally.
Can I parse dynamic websites?
Yes, tools like Selenium or Scrapy with middleware can handle dynamic content, though they require more setup.
How do I avoid getting blocked?
Use headers, proxies, and rate limiting to mimic human behavior and stay under the radar.
Is Scrapy worth learning?
Absolutely, especially for large-scale projects needing speed and structure.
Conclusion
Page parsing with Python isn’t just about extracting data—it’s a strategic skill that empowers professionals to turn the web into a goldmine of insights. By mastering these techniques, you’re not only saving time but also building a foundation for innovation, adaptable to any region’s needs.
From APIs to cloud scaling, this guide equips you to handle diverse challenges. Experiment with these tools, respect ethical boundaries, and watch your projects soar.
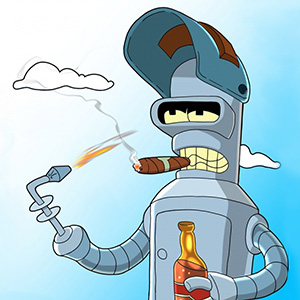
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.