Number Parsing
Number parsing refers to the process of converting a string representation of a number into its integer or floating-point numeric value. It is an essential capability required in many computer programming tasks and applications.
Why is Number Parsing Important?
-
User input – When accepting numeric input from users, the input is provided as a string which needs to be parsed and converted to an actual number for mathematical operations.
-
Reading data – When reading data from files, databases or over the network, numeric data is transmitted as text and needs parsing to be useful in the program.
-
Inter-system communication – Exchanging data between systems requires converting numbers into strings for transmission, requiring parsing on the receiving end.
-
Human readability – Displaying numbers in human readable string form for output requires the opposite conversion from numeric variables to text.
So being able to accurately and efficiently parse string representations into numbers and vice versa is a fundamental requirement in most programming languages and systems.
Challenges in Number Parsing
Number parsing seems straightforward but has some subtle complexities that need to be handled correctly:
-
Leading and trailing whitespace – Input strings may have unwanted whitespace characters around the digits that need to be removed first.
-
Invalid formats – The input may not conform to expected number syntax like containing letters in the string.
-
Regional variations – Different locales use different symbols for decimal point and digit grouping.
-
64-bit precision – Parsing very large numbers might result in a loss of precision in certain languages.
-
Hexadecimal – Parsing hexadecimal numbers like
0xFF
requires recognizing prefixes like0x
-
Scientific notation – Numbers in exponential notation like
1.2e-5
needs special handling. -
Sign handling – The sign for negative numbers may be a leading or trailing minus.
So the parser has to be flexible enough to handle these variations correctly while efficiently converting the text to numeric values.
Approaches for Number Parsing
The various techniques for parsing string numbers are:
Built-in parsers
Most modern languages like Java, Python, JavaScript etc have built-in methods or libraries to parse strings into numbers while handling all the intricacies:
-
Java –
Integer.parseInt()
,Double.parseDouble()
-
Python –
int()
,float()
-
JavaScript –
parseInt()
,parseFloat()
These are the easiest options to use in most cases.
Regular expressions
Regular expressions can match and capture the parts of a number format allowing extraction of the sign, integer and fractional digits to recreate the numeric value.
This gives more control over parsing and validating complex or custom formats.
Character-by-character
The parsing can happen character by character by analyzing each digit and symbol to reconstruct the number. This is more complex but works for custom formats when needed.
Third-party libraries
For special needs like high performance parsing of large data sets or specific number formats, third party libraries provide optimized routines and configuration options.
Best Practices for Number Parsing
To achieve robust and accurate number parsing, some best practices should be followed:
- Trim any leading and trailing whitespace from the input string.
- Use language/locale aware parsers that understand regional number conventions.
- Use radix parameters to indicate decimal vs hexadecimal numbers.
- Allow both e and E in exponential notation.
- Allow both + and – symbols for sign indication.
- Validate that the input string contains only valid digits before attempting conversion.
- Handle edge cases like empty strings, infinity, NaN etc correctly.
- Use precise data types like
BigDecimal
to avoid precision loss for large numbers.
Overall, leveraging inbuilt parsers is the best option for general use cases. But for advanced scenarios with specific needs, custom parsers may be required for flexibility and control.
Conclusion
Number parsing is a key programming capability that enables processing of numeric data from diverse sources. Robust implementations require handling myriad formats and edge cases while delivering high performance. Utilizing language primitives or specialized libraries helps tackle this complex task. Careful use of validation, precision data types and locale awareness results in reliable numeric conversion.
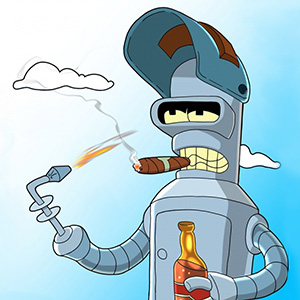
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.