News Scraping through Python: Tools, Techniques, and Best Practices
Introduction to News Scraping
In our information age, scraping news has become an essential skill for journalists, researchers, and data analysts. Python, with its rich ecosystem of web scraping libraries, provides the most efficient way to collect and process news data at scale. Whether you’re tracking market trends, monitoring media coverage, or building a news aggregator, Python offers the tools you need.
News scraping involves automatically extracting information from news websites and structuring it for analysis. Unlike manual collection, automated scraping allows you to:
- Process hundreds of articles in minutes
- Track news trends over time
- Create customized news feeds
- Perform sentiment analysis on media coverage
Why Python Dominates News Scraping
Python has become the lingua franca of web scraping for several compelling reasons:
1. Comprehensive Library Support
Python’s scraping ecosystem includes:
- BeautifulSoup for parsing HTML/XML
- Scrapy for large-scale scraping projects
- Requests for handling HTTP requests
- Selenium/Playwright for JavaScript-heavy sites
2. Gentle Learning Curve
Python’s simple syntax means you can start scraping quickly, even if you’re not an expert programmer.
3. Powerful Data Processing
After scraping, Python’s data science stack (Pandas, NumPy) makes cleaning and analyzing news data straightforward.
Essential Python Tools and Libraries
Library | Best For | Difficulty |
---|---|---|
BeautifulSoup + Requests | Basic scraping of static sites | Beginner |
Scrapy | Large-scale, complex projects | Intermediate |
Selenium | JavaScript-rendered content | Intermediate |
Newspaper3k | News-specific extraction | Beginner |
Step-by-Step Guide to Scraping News Websites
1. Setting Up Your Environment
First, install the necessary libraries:
pip install beautifulsoup4 requests pandas
2. Inspecting the Website
Use browser developer tools (F12) to examine the HTML structure. Identify the elements containing headlines, article text, and publication dates.
3. Writing the Scraper
Here’s a basic example using BeautifulSoup:
import requests
from bs4 import BeautifulSoup
import pandas as pd
url = "https://example-news-site.com/latest"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
articles = []
for item in soup.select('.news-item'):
title = item.select_one('h2').text
summary = item.select_one('.excerpt').text
date = item.select_one('.date').text
articles.append({'title': title, 'summary': summary, 'date': date})
df = pd.DataFrame(articles)
df.to_csv('news_data.csv', index=False)
Advanced Scraping Techniques
Handling Pagination
Many news sites spread content across multiple pages. Here’s how to scrape them all:
base_url = "https://example-news.com/page/"
for page in range(1, 6): # Scrape first 5 pages
url = f"{base_url}{page}"
# Add scraping logic here
Dealing with Anti-Scraping Measures
Some sites block scrapers. Countermeasures include:
- Rotating user agents
- Using proxies
- Adding delays between requests
Legal and Ethical Considerations
Always check a website’s robots.txt
file (e.g., example.com/robots.txt
) before scraping. Key guidelines:
- Respect
Disallow
directives - Limit request rate (1 request every 2-3 seconds)
- Don’t scrape copyrighted content for republication
- Consider using official APIs when available
Real-World Case Studies
Case Study 1: Media Monitoring Dashboard
A marketing firm built a Python scraper to track mentions of client brands across 50+ news sites. The system:
- Collected 5,000+ articles monthly
- Used NLP for sentiment analysis
- Generated automated reports
Case Study 2: Financial News Analyzer
A hedge fund created a scraper to extract earnings reports and analyst predictions, helping them:
- Identify market trends earlier
- Correlate news with stock movements
- Make data-driven investment decisions
When to Use Alternatives to Scraping
Sometimes scraping isn’t the best solution. Consider these alternatives:
Scenario | Alternative |
---|---|
Limited technical resources | News API services (NewsAPI, SerpAPI) |
Need historical data | Commercial datasets (LexisNexis, GDELT) |
Strict terms of service | RSS feeds or publisher partnerships |
FAQ
How often should I update my scrapers?
News sites frequently change their layouts. Monitor your scrapers weekly and update as needed.
What’s the difference between scraping and crawling?
Scraping extracts data from pages, while crawling discovers pages to scrape (like search engines do).
Can I scrape social media for news?
Most platforms prohibit scraping in their ToS. Use their APIs instead.
How do I store scraped news data?
CSV is fine for small projects. For larger datasets, consider SQL databases or cloud storage.
Is scraping behind a login legal?
Generally no, unless you have explicit permission. Avoid scraping password-protected content.
Conclusion
News Scraping with Python opens up powerful possibilities for data analysis and business intelligence. By mastering the tools and techniques covered in this guide while respecting legal boundaries, you can transform raw news data into valuable insights. Remember that with great scraping power comes great responsibility – always scrape ethically and consider the impact of your data collection.
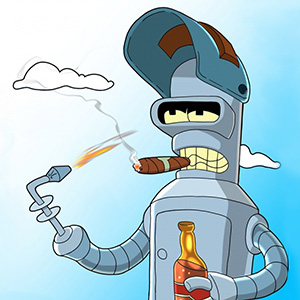
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.