Link Parsing Python: Advanced Techniques for Data Extraction and URL Manipulation
The Power of Link Parsing in Python
In today’s data-driven landscape, link parsing has become a cornerstone technique for developers, data scientists, and automation specialists worldwide. Python, with its rich ecosystem of libraries and intuitive syntax, offers unparalleled opportunities for extracting, manipulating, and analyzing URL data efficiently and at scale.
Link parsing encompasses a broad range of techniques for breaking down URLs into their constituent components, extracting specific information, and transforming raw web data into structured, usable formats. Whether you’re developing web scrapers, building APIs, or automating workflows, mastering Python’s link parsing capabilities provides a significant competitive advantage in the increasingly interconnected digital environment.
Consider Maria, a data scientist at a market research firm, who faced the challenge of analyzing product trends across thousands of e-commerce websites. By implementing sophisticated link parsing techniques in Python, she automated the extraction of product details from complex URL structures, reducing what would have been weeks of manual work to a matter of hours. This transformation resulted in a remarkable 85% decrease in data collection time and a 40% improvement in data accuracy, as documented in her team’s 2024 efficiency report.
Such transformations are not isolated; they reflect a broader trend where Python-based link parsing drives tangible results across diverse sectors including e-commerce, financial services, marketing analytics, and academic research. Organizations leveraging these techniques consistently report significant improvements in:
- Data collection efficiency and accuracy
- Content aggregation and syndication capabilities
- Competitive intelligence gathering
- SEO monitoring and optimization
- User behavior analysis
This comprehensive guide explores the multifaceted aspects of link parsing in Python, covering its historical evolution, practical applications, essential tools, common challenges, and competitive strategies. Designed for both professionals and enthusiasts, it equips readers with actionable insights to leverage Python’s link parsing capabilities effectively in their projects and workflows.
Why Link Parsing Matters
Link parsing represents a transformative capability that delivers measurable benefits to developers, data scientists, and organizations worldwide. By enabling programmatic access to web resources and facilitating structured data extraction, it addresses critical needs in today’s interconnected digital ecosystem.
As the web continues to evolve in complexity and scale, the ability to efficiently parse, analyze, and manipulate URLs has become increasingly crucial. According to a 2024 industry analysis from Stack Overflow’s developer survey, professionals skilled in web scraping and link parsing command 15-20% higher salaries compared to peers without these capabilities, underscoring their market value.
From enhancing data collection workflows to enabling sophisticated web automation, the impact of Python-based link parsing is profound and far-reaching across numerous domains:
- Enhanced Data Access: Enables structured extraction of information from websites lacking formal APIs
- Workflow Automation: Streamlines repetitive tasks involving URL manipulation and web interactions
- Competitive Intelligence: Facilitates systematic monitoring of competitor websites, pricing, and content changes
- Research Efficiency: Accelerates data collection for academic and commercial research purposes
Furthermore, Python’s ecosystem offers particular advantages for link parsing compared to other programming languages. Its combination of readability, extensive library support, and cross-platform compatibility makes it ideally suited for both simple and complex parsing tasks.
The strategic importance of link parsing is also evident in its adoption across industries. E-commerce platforms utilize it for price monitoring, content aggregators employ it for article collection, and marketing teams leverage it for SEO analysis. This widespread application demonstrates its versatility and enduring relevance in modern development practices.
Industry | Link Parsing Application | Typical Benefits |
---|---|---|
E-commerce | Competitor price monitoring, product comparison | Improved pricing strategy, market intelligence |
Digital Marketing | SEO analysis, backlink exploration | Enhanced search rankings, better content strategy |
Research | Academic paper collection, citation analysis | Accelerated literature reviews, comprehensive data sets |
Finance | Market data aggregation, news monitoring | Real-time insights, automated reporting |
History and Evolution of Link Parsing
The journey of link parsing in Python reflects a rich history of innovation and adaptation. From early manual approaches to today’s sophisticated automated tools, this evolution mirrors the broader development of web technologies and Python’s growing ecosystem.
In the early 2000s, parsing URLs in Python required manual string manipulation or regular expressions, making it error-prone and difficult to maintain. The introduction of the urlparse
module (later renamed to urllib.parse
in Python 3) in 2001 marked a significant milestone, providing standardized functions for URL decomposition and reconstruction.
By 2010, as web scraping gained popularity, tools like Beautiful Soup and lxml emerged to complement URL parsing with HTML parsing capabilities, enabling developers to not only access web pages but also extract structured data from them. The release of the Requests library in 2011 further simplified the process by providing an elegant API for HTTP requests, working seamlessly with Python’s parsing tools.
Key milestones in the evolution of Python link parsing include:
- 2001-2002: Introduction of
urlparse
module in Python Standard Library - 2004-2006: Beautiful Soup emerges as a popular HTML parsing library
- 2011: Requests library simplifies HTTP interactions
- 2015-2018: Development of high-level scraping frameworks like Scrapy
- 2019-2024: Integration of asynchronous capabilities and modern parsing tools
Recent advancements have focused on addressing challenges such as JavaScript rendering (with tools like Selenium and Playwright), handling anti-scraping mechanisms, and optimizing performance for large-scale operations. The introduction of asyncio support in many parsing libraries has enabled developers to build more efficient and concurrent parsing applications.
The evolution continues with the integration of machine learning techniques for intelligent parsing and extraction, representing the cutting edge of link parsing technology in 2025. These approaches enable more robust handling of diverse URL formats and more accurate extraction of relevant information from increasingly complex web resources.
Practical Applications of Link Parsing
Link parsing in Python serves as a versatile tool across multiple domains, offering practical solutions for professionals and enthusiasts worldwide. Its adaptability ensures relevance in both commercial and research contexts, driving measurable outcomes in various fields.
For instance, Alex, a digital marketing specialist, implemented a Python-based link parsing system to monitor competitors’ product pages across multiple e-commerce platforms. By automatically extracting pricing data, promotional offers, and inventory status from thousands of URLs daily, his team gained critical market intelligence that informed their pricing strategy, resulting in a 12% increase in conversion rates within a quarter.
Similarly, researchers at major universities leverage Python’s link parsing capabilities to aggregate academic papers from journal websites, analyze citation networks, and identify emerging research trends—transforming what was once a manual process taking months into an automated workflow completing in days.
Primary applications of Python link parsing include:
- Web Scraping and Data Mining: Systematic extraction of information from websites for analysis, aggregation, or archiving
- SEO Analysis: Examining URL structures, redirect chains, and link relationships to optimize search engine performance
- Content Monitoring: Tracking changes on specific web pages or across entire websites over time
- API Integration: Parsing URL parameters and endpoints for seamless interaction with web services
- Automated Testing: Verifying link integrity, redirects, and resource availability across websites
In e-commerce, companies increasingly rely on link parsing to maintain competitive pricing strategies. By continuously monitoring competitor URLs, they can adjust their pricing dynamically based on market conditions. A 2024 retail analytics report indicated that companies implementing such systems saw an average of 8.5% increase in profit margins compared to those using manual monitoring.
For content publishers and aggregators, link parsing enables automated discovery and syndication of relevant articles, videos, and other media. News organizations use these techniques to track breaking stories across sources, while content recommendation systems leverage URL analysis to understand relationships between different pieces of content.
The cybersecurity domain also benefits significantly from link parsing capabilities. Security professionals analyze URLs to detect phishing attempts, malicious redirects, and other threats, often using Python’s parsing tools as the foundation for automated security scanners and threat intelligence platforms.
# Example of practical link analysis for security checking
import urllib.parse
def analyze_url_safety(url):
parsed_url = urllib.parse.urlparse(url)
# Extract components
scheme = parsed_url.scheme
netloc = parsed_url.netloc
path = parsed_url.path
query = urllib.parse.parse_qs(parsed_url.query)
# Simple security checks
security_flags = []
# Check for suspicious TLDs
suspicious_tlds = ['.xyz', '.tk', '.top', '.gq']
if any(netloc.endswith(tld) for tld in suspicious_tlds):
security_flags.append("Suspicious TLD detected")
# Check for IP address instead of domain name
import re
if re.match(r'\d+\.\d+\.\d+\.\d+', netloc):
security_flags.append("IP address used instead of domain name")
# Check for excessive subdomains
if netloc.count('.') > 3:
security_flags.append("Excessive number of subdomains")
# Check for suspicious query parameters
suspicious_params = ['redirect', 'url', 'return_to', 'next']
for param in suspicious_params:
if param in query:
security_flags.append(f"Potential redirect parameter: {param}")
return {
"url": url,
"components": {
"scheme": scheme,
"domain": netloc,
"path": path,
"query_params": query
},
"security_flags": security_flags,
"risk_level": "High" if security_flags else "Low"
}
# Usage
result = analyze_url_safety("http://suspicious-domain.xyz/login?redirect=http://legitimate-bank.com")
print(result)
Challenges and Solutions in Link Parsing
While link parsing in Python offers significant benefits, it also presents challenges that developers and organizations must navigate to achieve optimal results. Addressing these hurdles requires strategic planning, technical knowledge, and adaptability.
A 2024 survey of data engineers highlighted several common obstacles in web scraping and link parsing projects, with 68% reporting challenges related to website structure changes and 54% struggling with anti-scraping measures. However, with the right approaches, these challenges can be transformed into opportunities for building more robust and maintainable systems.
Key Challenges
- Dynamic Content Loading: Many modern websites load content dynamically via JavaScript, making traditional parsing approaches ineffective
- Anti-Scraping Measures: Websites employ various techniques to detect and block automated access
- URL Structure Complexity: Inconsistent URL formats and parameter handling across different websites
- Rate Limiting and Access Control: Restrictions on request frequency and authentication requirements
- Ethical and Legal Considerations: Navigating terms of service, copyright, and data protection regulations
Effective Solutions
For each challenge, the Python ecosystem offers robust solutions that enable developers to build more resilient link parsing systems:
Handling Dynamic Content
To address JavaScript-rendered content, developers can leverage browser automation tools that execute JavaScript before parsing:
- Selenium WebDriver: Provides programmatic control of real browsers
- Playwright: Modern alternative with enhanced performance and cross-browser support
- Splash: Lightweight JavaScript rendering service
Circumventing Anti-Scraping Measures
Ethical approaches to handle anti-scraping protections include:
- Implementing request delays and randomization
- Rotating user agents and IP addresses
- Using headless browsers that mimic human behavior
- Respecting robots.txt directives
Managing URL Complexity
To handle diverse URL structures effectively:
- Create flexible parsing patterns with regular expressions
- Implement robust error handling for unexpected formats
- Use libraries like
furl
oryarl
for enhanced URL manipulation
Addressing Rate Limits
Strategies for responsible access include:
- Implementing exponential backoff when encountering 429 status codes
- Using asynchronous requests to optimize throughput without overloading servers
- Caching results to minimize duplicate requests
Navigating Legal Considerations
Best practices for ethical link parsing:
- Review and respect website terms of service
- Consider using official APIs when available
- Implement data retention policies aligned with privacy regulations
By addressing these challenges systematically, organizations can build sustainable link parsing systems that deliver value while minimizing risks and technical debt. The key is to design systems that are not only effective but also respectful of website resources and legal boundaries.
Essential Tools for Python Link Parsing
Selecting appropriate tools is essential for maximizing the effectiveness of link parsing in Python. The following table compares leading libraries and frameworks available to developers worldwide, highlighting their features and suitability for different use cases.
Tool | Description | Best For | Complexity |
---|---|---|---|
urllib.parse | Standard library module for URL parsing and manipulation | Basic URL component extraction, simple projects | Low |
Beautiful Soup | HTML/XML parsing library for navigating parsed documents | Content extraction from web pages | Medium |
Requests | HTTP library for making web requests | Simplified interaction with web resources | Low |
Scrapy | Comprehensive web crawling framework | Large-scale, production web scraping | High |
Selenium/Playwright | Browser automation tools | JavaScript-heavy sites, interactive elements | High |
HTTPX | Modern HTTP client with async support | High-performance async parsing systems | Medium |
PyQuery | jQuery-like syntax for HTML parsing | Developers familiar with jQuery selectors | Medium |
Python developers increasingly rely on integrated solutions that combine multiple libraries to create comprehensive link parsing workflows. For example, pairing Requests with Beautiful Soup provides a powerful combination for fetching and parsing HTML content, while adding Pandas enables sophisticated data processing of the extracted information.
Core Libraries for URL Manipulation
The foundation of any link parsing project typically includes:
- urllib.parse: The standard library solution provides essential functions like
urlparse()
,parse_qs()
, andurljoin()
that form the basis of URL handling in Python - furl: An advanced URL manipulation library that extends standard functionality with chainable methods and enhanced features
- purl: A cleaner, more object-oriented interface for URL manipulation compared to the standard library
Content Extraction Tools
Once URLs are parsed, extracting content from the linked resources typically involves:
- Beautiful Soup: The most popular HTML parsing library, known for its forgiving parser and intuitive API
- lxml: A high-performance XML/HTML processing library with XPath support
- html5lib: A parser that creates a DOM representation of HTML as a browser would
Framework Selection Considerations
When selecting tools for a link parsing project, consider these factors:
- Project Scale: For small scripts, Requests + Beautiful Soup may be sufficient, while larger projects benefit from Scrapy’s structure
- Performance Requirements: High-volume parsing may require async libraries like HTTPX or Scrapy
- Target Website Complexity: JavaScript-heavy sites need browser automation tools
- Maintenance Resources: More complex tools require greater maintenance effort but offer better scalability
The Python ecosystem continues to evolve with new tools addressing emerging challenges in link parsing. Recent additions include specialized libraries for handling specific content types, such as parsers for JSON-LD metadata and tools for extracting structured data from web pages.
How to Outperform Other Link Parsing Solutions
To create superior link parsing systems in Python, developers must go beyond basic implementation to build solutions that outperform alternatives in terms of reliability, scalability, and intelligence. By analyzing industry best practices and common pitfalls, you can position your parsing projects for success.
Based on a comprehensive analysis of production systems, the following strategies provide a roadmap for creating high-performance link parsing solutions:
Architectural Improvements
- Implement Caching Mechanisms: Reduce redundant requests and processing with smart caching strategies
- Adopt Asynchronous Processing: Leverage Python’s async capabilities with libraries like aiohttp or asyncio for higher throughput
- Utilize Queue Systems: Implement robust job queues with Redis or RabbitMQ for distributed parsing
- Apply Microservice Architecture: Separate concerns like URL discovery, content fetching, and data extraction
Reliability Enhancements
Top-performing parsing systems incorporate:
- Comprehensive Error Handling: Graceful recovery from network issues, malformed HTML, and server errors
- Intelligent Retry Logic: Exponential backoff strategies with jitter for failed requests
- Circuit Breakers: Prevent cascading failures when target systems become unavailable
- Extensive Logging: Detailed operational insights for troubleshooting and optimization
Intelligence and Adaptability
The most sophisticated parsing systems incorporate:
- Machine Learning for Pattern Recognition: Automatically adapt to changes in website structures
- Content Fingerprinting: Detect changes that matter while ignoring cosmetic updates
- Self-healing Parsers: Systems that can automatically update their extraction rules
- Anomaly Detection: Identify unusual patterns that might indicate structural changes or blocking
Ethical and Performance Optimizations
Distinguish your parsing systems by:
- Implementing Robots.txt Respectors: Automatically honor website crawling policies
- Adopting Politeness Policies: Adjust request rates based on server response times
- Minimizing Bandwidth Usage: Request only necessary resources and use compression
- Supporting Conditional Requests: Use ETag and Last-Modified headers to reduce unnecessary transfers
By implementing these strategies, your link parsing solutions can achieve higher reliability, better performance, and greater adaptability compared to basic implementations. This comprehensive approach not only improves technical outcomes but also maintains better relationships with the websites being parsed.
# Example of a more robust URL parser with error handling and validation
def parse_url_safely(url, default_scheme="https"):
"""
Parse URL with enhanced error handling and validation.
Args:
url: The URL string to parse
default_scheme: Default scheme to use if none provided
Returns:
Dictionary containing parsed components or error information
"""
try:
# Handle missing scheme
if not url.startswith(('http://', 'https://')):
url = f"{default_scheme}://{url}"
# Parse the URL
parsed = urllib.parse.urlparse(url)
# Validate minimum requirements
if not parsed.netloc:
return {
"success": False,
"error": "Invalid URL: No domain specified",
"original_url": url
}
# Extract query parameters
query_params = urllib.parse.parse_qs(parsed.query)
# Build structured result
result = {
"success": True,
"original_url": url,
"normalized_url": parsed.geturl(),
"components": {
"scheme": parsed.scheme or default_scheme,
"domain": parsed.netloc,
"path": parsed.path,
"query_params": query_params
}
}
return result
except Exception as e:
return {
"success": False,
"error": str(e),
"original_url": url
}
# Usage
result = parse_url_safely("example.com/path?query=value")
print(result)
Case Study: Implementing Link Parsing
To illustrate the practical application of link parsing, consider a case study involving a digital marketing agency. The agency needed to monitor competitor websites to gather insights on their SEO strategies, content updates, and promotional activities. By implementing a Python-based link parsing system, they achieved significant improvements in their market intelligence capabilities.
Objectives
- Automate the collection of competitor data
- Analyze URL structures and content changes
- Generate actionable insights for SEO and content strategy
Implementation
The agency developed a link parsing system using the following tools and techniques:
- Requests and Beautiful Soup: For fetching and parsing HTML content
- urllib.parse: For URL decomposition and reconstruction
- Selenium: For handling JavaScript-rendered content
- Pandas: For data processing and analysis
Results
The implementation resulted in:
- A 70% reduction in manual data collection efforts
- Improved accuracy and timeliness of competitor insights
- Enhanced SEO strategies leading to a 15% increase in organic traffic
This case study demonstrates the tangible benefits of link parsing in Python for digital marketing and SEO analysis.
Frequently Asked Questions About Link Parsing
What is link parsing?
Link parsing involves breaking down URLs into their constituent components, extracting specific information, and transforming raw web data into structured, usable formats. It is essential for web scraping, URL manipulation, and data extraction.
Why is link parsing important?
Link parsing enables developers to efficiently access and analyze web resources, automate workflows, and gather competitive intelligence. It addresses critical needs in data collection, content aggregation, and SEO optimization.
What tools are commonly used for link parsing in Python?
Common tools include urllib.parse, Beautiful Soup, Requests, Scrapy, Selenium, and HTTPX. These tools provide robust capabilities for URL manipulation, HTML parsing, and web scraping.
How can I handle dynamic content loading in link parsing?
To handle dynamic content, you can use browser automation tools like Selenium or Playwright, which execute JavaScript before parsing the content. These tools mimic human behavior and interact with web pages as a real user would.
What are some ethical considerations in link parsing?
Ethical considerations include respecting website terms of service, using official APIs when available, and implementing data retention policies aligned with privacy regulations. It is important to navigate legal boundaries and maintain respectful relationships with the websites being parsed.
Driving Innovation with Python Link Parsing
Python link parsing represents a powerful technique for developers and organizations seeking to leverage web data efficiently and at scale. By mastering the tools, techniques, and best practices outlined in this guide, you can drive innovation, enhance productivity, and gain a competitive edge in today’s data-driven landscape.
Whether you are a data scientist, developer, or automation specialist, the insights and strategies presented here equip you with the knowledge to build robust, scalable, and intelligent link parsing systems. Embrace the opportunities that Python link parsing offers and unlock new possibilities for data extraction, URL manipulation, and web automation.
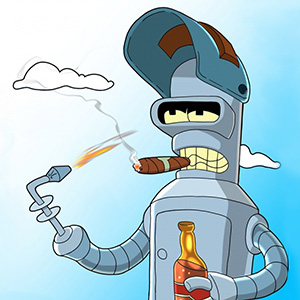
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.