JSON Parsing Data
JavaScript Object Notation (JSON) has emerged as a prevalent lightweight data structure that maintains simplicity for human reading and authoring while enabling effortless machine parsing and production. Although incorporating JavaScript semantic elements, JSON’s language-agnostic nature allows adoption across programming languages. Its streamlined syntax and bandwidth economy compared to Extensible Markup Language (XML) have fueled its commonplace integration into data transmission between servers and web apps. Capitalizing on intricacy avoidance in both machine and human comprehension, JSON delivers a widely embraced structured data rendering optimized for interchanging information. The minimalist composition empowers efficient analysis and simplifies decoding across systems, cementing JSON as a ubiquitous portable data standard.
JSON Format and Syntax
The JSON format is text only and uses human-readable text to transmit data objects made up of attribute–value pairs. Here are some key characteristics of the JSON syntax:
- Data is represented in name/value pairs
- Data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
- Data is indexed by key values
For example:
json
{
"name": "John",
"age": 30,
"isEmployed": true,
"hobbies": ["hiking", "swimming", "reading"]
}
A JSON object’s elemental components incorporate rudimentary data categories such as numeric values, textual strings, and Boolean logical variables. Absent are the complex data formats possible in full programming languages. This simplification to basic building blocks circumscribes the range of data types for streamlined machine parsing while enlarging human comprehensibility. By constraining the scope to these core primitives rather than intricate or opaque data structures, JSON fulfills its role as a universal data specification well-suited for transportation and clarity. The inclusion solely of widespread, easily decoded rudiments in its definition eliminates extra decoding effort and enables straightforward usage across systems, upholding interoperability. The spartan syntax eases not only exchange between disparate platforms but also rapid manual interpretation for debugging or edification.
Why Use JSON for Data Interchange
There are several key advantages that make JSON a widely adopted data interchange format:
- Lightweight – files are very small compared to other formats like XML. This improves transmission speeds and efficiency.
- Readable – is easier for humans to read and debug because it looks similar to JavaScript objects.
- Popular – is supported by nearly all modern programming languages and platforms. There are JSON libraries available for most environments.
- Fast Parsing – The simple syntax of JSON allows for quick parsing and generation of JSON data. Parsing JSON is generally faster than parsing XML.
- Flexible – data can be used for simple data sets, but also complex hierarchical data. It works for ad hoc data exchange, but also formal data protocols.
Usage for Web APIs
JSON is commonly used for transmitting data in web APIs and web services. For example:
- An API request will hit the server and return a JSON object with the requested data
- The client-side code will parse this JSON response to work with the data
When client-side code transfers data to an application programming interface (API), it structures the payload utilizing JavaScript Object Notation (JSON) syntax. This formatting as a JSON object furnishes data standardization for communication from consumer to provider. The receiving API can efficiently decode the structured details encapsulated within the compact JSON package. This widespread exchange convention circumvents proprietary protocols, facilitating decoupled integration regardless of backend implementation. JSON’s language-independent paradigm institutes an interoperable data rendering optimized for transit across systems. By conforming to known parsing logic, JSON-formatted data enables seamless interpretation, allowing the client-supplied information to integrate smoothly into the server environment.
This allows web services to focus on business logic, while outsourcing the data serialization to proven JSON libraries.
JSON vs XML for Data Interchange
JSON serves a similar role to XML for data interchange. Here are some key differences:
- Size – files are smaller because they lack the extra markup and elements of XML.
- Readability – is more concise and easier to read than XML’s formal structure.
- Parsing – can be parsed directly to JavaScript objects. XML requires conversion code.
- Popularity – is now more widely used in web services and APIs. XML maintains use in document-focused formats.
So in most cases, JSON provides a lighter and faster alternative to XML when exchanging data between systems or over the web.
Conclusion
JSON’s simple syntax has helped it become a widespread format for serializing and transmitting structured data over the web and between applications. It strikes a balance between human readability and machine parsability that makes JSON a smart choice for common data interchange tasks and web-based APIs.
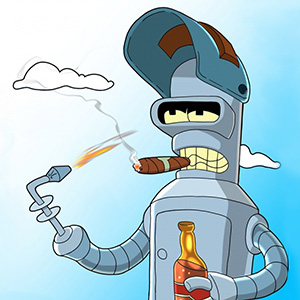
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.