Image Generation Script with PicLumen and Botasaurus
Introduction
For bulk image generation—crucial for mass publishing—you need efficient automation. This image generation script uses PicLumen, a free tool, and the Botasaurus library in Python to create images from text queries listed in a file. We’ll walk through the setup and code step-by-step.
Setup
Create a project folder and set up a virtual environment:
py -m venv .venv
.venv\Scripts\activate
Install dependencies:
pip install python-dotenv botasaurus requests pillow pyperclip
Create queries.txt
with image prompts, one per line (e.g., “sunset over mountains”). Then, add a .env
file with your PicLumen credentials after registering:
LOGIN=your_email
PASSWORD=your_password
The Script (main.py)
Here’s the optimized Botasaurus image generator script for PicLumen scraping:
from botasaurus.browser import browser, Driver, Wait
import os
import time
from dotenv import load_dotenv
import requests
from io import BytesIO
from PIL import Image
# Load environment variables
load_dotenv()
login = os.getenv("LOGIN")
password = os.getenv("PASSWORD")
# Global settings
global_image_counter = 0
num_images_to_download = 4 # Limit per query
# Paths
base_dir = os.path.dirname(__file__)
images_dir = os.path.join(base_dir, 'images')
os.makedirs(images_dir, exist_ok=True)
# URLs and headers
login_url = 'https://piclumen.com/app/account'
dashboard_url = 'https://piclumen.com/app/image-generator/create'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) Chrome/85.0.4183.121'
}
def read_queries_from_file(file_path):
"""Read text queries from a file."""
try:
with open(file_path, 'r', encoding='utf-8') as f:
return [line.strip() for line in f if line.strip()]
except FileNotFoundError:
print(f"Error: {file_path} not found.")
return []
def process_image_links(driver, images_dir):
"""Extract image URLs from the page."""
images = driver.select_all('img[src^="https://cdn.piclumen.com"]', wait=Wait.LONG)
return [img.get_attribute('src') for img in images if img.get_attribute('src')]
@browser(
reuse_driver=True, # Reuse browser instance
wait_for_complete_page_load=False,
headless=True # Optional: run without UI
)
def piclumen(driver: Driver, data=None):
global global_image_counter
# Login
driver.get(login_url, wait=5)
driver.type('input[placeholder="Email"]', login, wait=1)
driver.type('input[placeholder="Password"]', password, wait=1)
driver.click('#app > div > div.content > div.absolute > div.w-full > div > div.w-80 > div > div.mt-8 > button', wait=5)
driver.get(dashboard_url, wait=10)
# Open settings once
driver.click('.tool-bar-item', wait=3) # Adjust selector if needed
# Load queries
queries = read_queries_from_file(os.path.join(base_dir, 'queries.txt'))
print(f"Processing {len(queries)} queries")
for query in queries:
print(f"Generating images for: {query}")
try:
# Set to generate 4 images
driver.click('#confExtendArea > div > div.flex.gap-4 > div:nth-child(3) > div > div:nth-child(4)', wait=3)
# Input query
driver.type('textarea', query, wait=2)
driver.click('#confExtendArea > div > div.mt-3 > div > div.flex.gap-3 > button > span', wait=3)
# Wait for generation (adjust time based on observation)
time.sleep(80)
# Extract and download images
image_urls = process_image_links(driver, images_dir)
for url in image_urls[:num_images_to_download]:
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
img = Image.open(BytesIO(response.content))
global_image_counter += 1
output_path = os.path.join(images_dir, f"image_{global_image_counter}.png")
img.save(output_path, 'PNG')
print(f"Saved: {output_path}")
except Exception as e:
print(f"Error saving {url}: {e}")
except Exception as e:
print(f"Error processing '{query}': {e}")
if __name__ == "__main__":
piclumen()
How it works:
- Logs into PicLumen with credentials from
.env
. - Reads queries from
queries.txt
. - Generates 4 images per query, downloads them as PNGs.
- Saves images with a global counter in the
images
folder.
Conclusion
This image generation script leverages PicLumen’s free service and Botasaurus for a cost-effective Python image automation solution. Perfect for bulk content creation in 2025, it simplifies generating images from text queries. Adjust the wait time or image count as needed, and enjoy free, automated image production!
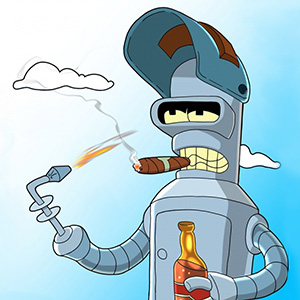
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.