7 Compelling Reasons to Master HTML Scraping Today
What Is HTML Scraping and Why It Matters
For enthusiasts and professionals, HTML Scraping offers a gateway to extract valuable data from websites effortlessly. Whether you’re tracking market trends, gathering research, or automating tasks, this technique unlocks a world of possibilities. It involves parsing HTML code to pull specific information—like prices, articles, or contact details—without manual copying. With the right tools and know-how, you can turn chaotic web pages into structured data tailored to your needs.
The beauty of this skill lies in its versatility. Businesses use it to monitor competitors, researchers rely on it for data collection, and developers automate workflows. Mastering this method can set you apart in a digital world. Curious about how it works or why it’s worth your time? Let’s dive into the reasons and practical tips that make HTML Scraping a must-learn skill.
Top Benefits of HTML Scraping
Why should you care about HTML Scraping? It’s more than a tech trick—it’s a competitive edge. Imagine saving hours by automating data collection or uncovering insights your peers overlook. This section explores the top advantages, grounded in real-world applications.
From boosting efficiency to enabling data-driven decisions, the benefits are tangible. Many businesses leveraging web data see improved ROI—a trend that highlights its value. Here’s a breakdown of why it’s a game-changer:
- Efficiency: Cut down manual work by automating repetitive tasks.
- Insights: Access real-time data for market analysis or research.
- Scalability: Handle large datasets without breaking a sweat.
- Customization: Tailor outputs to fit your goals.
Best Tools for HTML Scraping
Ready to dive into HTML Scraping? The right tools can make or break your experience. For enthusiasts and professionals, choosing solutions that balance power, ease, and affordability is key. This section highlights top options, each with unique strengths to suit your goals.
No single tool fits all, but these stand out for their reliability and community support. Whether you’re coding in Python or prefer a no-code approach, there’s something here for you. Here’s a quick comparison:
Tool | Type | Best For | Cost |
---|---|---|---|
Beautiful Soup | Python Library | Developers needing flexibility | Free |
Scrapy | Framework | Large-scale projects | Free |
Octoparse | No-Code Software | Beginners or pros avoiding code | Free tier / Paid plans |
ParseHub | No-Code Tool | Visual scraping users | Free tier / Paid plans |
Beautiful Soup, paired with Python, is a favorite for its simplicity and control. Scrapy shines for bigger tasks, while Octoparse and ParseHub cater to those skipping code entirely. Test them out—your perfect fit depends on your project.
Practical Examples of HTML Scraping
Wondering how to effectively use HTML Scraping? Real-world examples bring the concept to life. From e-commerce to research, this technique solves problems and creates opportunities. Let’s explore a few scenarios.
Picture a small business owner tracking competitor prices. Using Scrapy, they scrape product pages daily, building a dataset to adjust their pricing strategy. Or consider a researcher pulling public data from government sites for a report—Beautiful Soup makes it quick and clean. These cases show the power of practical examples of HTML Scraping.
- E-commerce: Monitor prices or stock levels on rival sites.
- Research: Collect stats from public portals.
- Marketing: Scrape social media for trends or leads.
Strategies to Excel at HTML Scraping
Success with HTML Scraping isn’t just about tools—it’s about strategy. Smart approaches save time and boost results. This section shares strategies for HTML Scraping that work, whether you’re a newbie or a pro.
First, plan your target. Identify the data you need—tags, classes, or IDs—before scraping. Next, respect robots.txt to stay ethical and avoid blocks. Many failed scrapes stem from poor planning or rate limits. Here’s how to nail it:
- Map the Site: Use browser dev tools to find data locations.
- Rotate IPs: Avoid bans with proxies or delays.
- Test Small: Scrape a page before scaling up.
- Handle Errors: Build retries for timeouts or changes.
Another tip? Use headless browsers like Puppeteer for dynamic sites. They render JavaScript, grabbing data static tools miss. Adapt these tactics for top results.
Common Challenges and Solutions
Scraping isn’t always smooth sailing. Websites vary in complexity, and you’ll face hurdles. This section tackles common issues and offers best solutions for HTML Scraping to keep you on track.
One big challenge? Anti-scraping measures like CAPTCHAs or IP bans. Another is dynamic content—think JavaScript-heavy pages. Data inconsistency, like shifting HTML structures, can also trip you up. Here’s how to overcome them:
Challenge | Solution | Tool/Tip |
---|---|---|
Anti-Scraping Blocks | Use proxies or CAPTCHA solvers | Proxy services |
Dynamic Content | Deploy headless browsers | Puppeteer, Selenium |
HTML Changes | Build flexible parsers | Regular expressions |
Legal Concerns | Check terms of service | Review site policies |
Patience and testing are your allies. Start small, tweak as needed, and stay ethical to keep things running smoothly.
Frequently Asked Questions
Is HTML Scraping Legal?
It depends. Scraping public data is often fine, but private or copyrighted content can raise issues. Check site terms to stay compliant.
How Do I Start with HTML Scraping?
Pick a tool like Beautiful Soup or Octoparse. Start with a simple site, inspect its HTML, and extract a small dataset. Practice builds confidence.
What’s the Best Tool for Beginners?
Octoparse or ParseHub. They’re no-code, user-friendly, and perfect for those new to scraping.
Can I Scrape Dynamic Sites?
Yes! Tools like Puppeteer or Selenium handle JavaScript-rendered pages. They’re ideal for modern sites.
Conclusion
HTML Scraping isn’t just about convenience—it’s a strategic lever for success. It empowers you to harness data others ignore, turning raw web pages into actionable insights. Whether you’re a hobbyist or a pro, the tools and tactics here can transform how you work. In a data-driven world, this skill isn’t optional—it’s essential.
Start small, experiment with tools, and adapt to challenges. The payoff? A sharper edge in your projects. What will you scrape first?
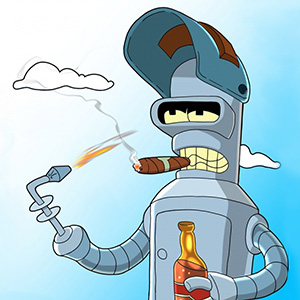
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.