Expression Parsing
Introduction to Expression Parsing
As an experienced developer, I often get asked to explain expression parsing and how it works under the hood. In essence, expression parsing involves analyzing an entered expression or formula and breaking it down into tokens that can then be evaluated. This allows applications to support complex expressions as input and process them correctly.
In this article, I’ll cover the key concepts around expression parsing and evaluation, common use cases, challenges, and best practices gleaned from years of work in this area. Whether you’re looking to implement expression support in your own app or just better understand this useful technique, read on for an in-depth guide.
Fundamentals of Expression Parsing
Expression parsing starts by taking an input formula and splitting it into individual tokens. This is known as lexical analysis or tokenization. For example, the expression 2 + 3 * (5 - 2)
would be split into tokens such as numbers, operators, parentheses and so on.
These tokens then need to be organized into an abstract syntax tree (AST) which represents the structure of the expression. This is done using a set of grammar rules – similar to those used by programming languages – that define valid syntax.
With the AST in hand, we can begin evaluation. This involves traversing the tree and following the order of operations to ultimately calculate a result. Expressions can contain various elements like math operators, boolean logic, conditional statements, functions calls and more, so the evaluation logic can get quite complex.
Overall this process allows even long, arbitrarily complex expressions to be decoded, organized and processed in an efficient way at runtime.
Use Cases for Expression Evaluation
There are a variety of scenarios where interpreting and evaluating complex expressions is highly useful:
-
Spreadsheets – Formulas provide extremely versatile functionality through expressions. Users can create calculations, reference cells dynamically, leverage built-in functions and more.
-
Dynamic Scripting – Many apps feature scripting languages that harness expressions to add programmatic capabilities without needing compilation or IDEs.
-
Query Languages – Query and filtering mechanisms often interpret expressions for dynamic data analysis without requiring static upfront definitions.
-
Financial Calculations – Interest rate computations, loan cost forecasting and other financial math relies heavily on formulaic expressions.
-
Custom Forms & Wizards – User interfaces frequently leverage expressions to enable dynamic form behavior without custom code.
And many more. Any time an application needs to allow formulas as input and evaluate them on the fly, expression parsing is required under the hood.
Challenges of Expression Evaluation
However, accurately interpreting expressions poses some unique challenges:
-
Lexical Analysis – Recognizing and classifying individual tokens in an expression Parse according to grammar rules requires significant upfront effort.
-
Syntax Verification – Once tokenized, validating syntax trees according various structural rules and constraints grows exponentially more difficult at scale.
-
Order of Operations – Following proper precedence during evaluation – respecting parentheses, exponents, multiplication/division before addition/subtraction – avoids incorrect calculations.
-
Efficient Processing – AST traversal and expression interpretation needs to happen quickly, even for large, deeply nested formulas.
-
Security & Safety – Allowing dynamic expressions as input opens potential vulnerability to code injection or recursion attacks if not careful.
Thankfully decades of research in compiler theory provide frameworks and best practices for handling these issues.
Best Practices for Expression Parsing
When tackling expression parsing and evaluation, I recommend keeping several best practices in mind:
-
Leverage Existing Libraries – Mature parsing libraries handle much of the heavy lifting and edge cases for you. Focus custom logic at the evaluation layer.
-
Build up from a Formal Grammar – Defining tokens, syntax rules, AST format and evaluation semantics formally is essential to robustness.
-
Validate Inputs – Restrict expression length, limit recursion depth, blacklist dangerous functions to prevent abuse.
-
Sandbox Evaluation – Executing dynamic code safely requires tightly controlling the evaluation environment.
-
Optimize Performance – Cache parsed tokens and ASTs when possible, implement efficient tree traversal, and more.
-
Provide Safety Nets – Allow default values, error handling and safe failure to avoid crashing on bad input.
No one said supporting expressions is simple! But following robust practices rooted in parsing theory makes taming this complexity tractable.
Conclusion
In closing, expression parsing underpins countless applications we use every day by empowering dynamic, formulaic input. Doing this properly involves lexically analyzing input strings, structuring them into abstract syntax trees, and carefully evaluating results step-by-step at runtime.
It’s a challenging but rewarding feat of engineering. I hope this breakdown gives you a head start at tackling expression parsing in your own software. Reach out if you have any other questions!
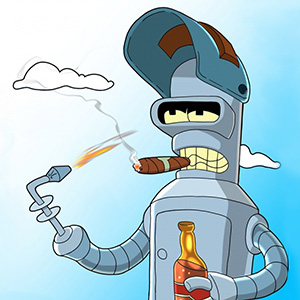
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.