Mastering Dynamic Website Parsing: 10 Proven Strategies
Ever tried pulling data from a website only to hit a wall because the content loads dynamically? Dynamic Website Parsing could be your ace in the hole. Whether you’re a web developer chasing real-time insights, a data enthusiast building a personal project, or a professional refining lead generation strategies, this guide is your roadmap. Packed with expert tips, hands-on tools like Python and SaaS platforms, and a dash of practical wisdom, you’re about to unlock a skill that’s in high demand.
Here’s the kicker: 80% of businesses now lean on web-scraped data to fuel decisions, per recent industry reports. Dynamic sites—think infinite scrolls, live feeds, or JavaScript-heavy pages—aren’t just tricky; they’re the norm. This article dives into the nitty-gritty, offering you a toolkit to tackle these challenges head-on. From browser automation to API tricks, we’ve got you covered.
What Is Dynamic Website Parsing?
At its core, Dynamic Website Parsing is about extracting data from websites that don’t play nice with traditional scraping. These are pages where content loads dynamically—via JavaScript, AJAX calls, or APIs—rather than sitting neatly in static HTML. Picture an online store updating prices as you browse or a social feed refreshing in real time. That’s the beast we’re taming here.
Unlike static scraping, which grabs pre-loaded HTML, dynamic parsing requires waiting for scripts to execute or tapping into backend data streams. It’s a bit like fishing with a net versus a spear—you need the right approach to snag what’s beneath the surface. This matters because modern web design leans heavily on interactivity, making these skills essential for anyone serious about data extraction methods.
Why Parse Dynamic Websites?
So why bother? The payoff is huge. Businesses use parsed data for competitive analysis—think tracking rival pricing in real time. Developers build tools that aggregate live stats, while hobbyists might scrape forums for rare insights. According to a 2023 survey, 65% of marketers say web data is their top source for leads. Dynamic parsing opens doors static scraping can’t touch.
But it’s not just about opportunity—it’s necessity. Static sites are fading as JavaScript frameworks like React and Angular dominate. Ignoring dynamic parsing is like refusing to upgrade your phone: you’ll miss out. Plus, mastering it sharpens your problem-solving chops, blending coding with strategy.
Best Tools for Dynamic Website Parsing
Ready to dive in? The right tools can turn a parsing nightmare into a breeze. Here’s a lineup of the best options, suited for pros and newcomers alike.
- Python + Selenium: Automates browsers to render JavaScript-heavy pages. It’s versatile, open-source, and a favorite among coders.
- Puppeteer: A Node.js gem for controlling headless Chrome or Chromium. Fast, lightweight, and perfect for scraping with precision.
- Scrapy: Python’s heavy-duty framework, extensible with middleware for dynamic content.
- Octoparse: A no-code SaaS tool that handles dynamic sites effortlessly—ideal if coding isn’t your thing.
- BeautifulSoup + Requests: Pair this with a headless browser for a lightweight combo.
Need more options? Hunter.io’s tool roundup breaks down even more choices. Each tool has its sweet spot—Selenium for flexibility, Puppeteer for speed, Octoparse for simplicity. Pick based on your project’s pulse.
Top Techniques to Parse Effectively
Tools are half the battle—techniques seal the deal. Here’s how to scrape dynamic websites effectively, with methods pros rely on.
Technique | Description | Best For |
---|---|---|
Headless Browsing | Simulates user actions with tools like Selenium or Puppeteer. | E-commerce, job boards |
API Reverse-Engineering | Intercepts backend API calls for raw JSON data. | Social media, live feeds |
DOM Manipulation | Targets rendered HTML after JavaScript runs. | News sites, blogs |
Event Listening | Captures data triggered by user events (e.g., clicks). | Interactive dashboards |
Extracting data effortlessly often means mixing these approaches. For instance, pairing headless browsing with API calls can cut through complex sites like a hot knife through butter. It’s about adapting—each site’s a puzzle begging to be solved.
Step-by-Step Python Parsing Guide
Python’s a powerhouse for Dynamic Website Parsing. Let’s walk through a practical example using Selenium to scrape a dynamic product page. First, install Selenium: pip install selenium
. You’ll also need a WebDriver (e.g., ChromeDriver).
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
# Set up headless Chrome
options = webdriver.ChromeOptions()
options.add_argument('--headless')
driver = webdriver.Chrome(options=options)
# Navigate to a dynamic site
driver.get('https://example-store.com/products')
time.sleep(3) # Wait for JS to load
# Extract data
products = driver.find_elements(By.CLASS_NAME, 'product-item')
for product in products:
name = product.find_element(By.TAG_NAME, 'h3').text
price = product.find_element(By.CLASS_NAME, 'price').text
print(f'{name}: {price}')
driver.quit()
This snippet loads a page, waits for content, and grabs product names and prices. Tweak the selectors based on your target site’s structure. Pro tip: Use WebDriverWait
instead of time.sleep
for smarter timing.
Scaling up? Add error handling, proxies, or a CSV export. Python’s flexibility makes it a go-to for data harvesting tips—experiment and refine as you go.
Case Study: Parsing an E-commerce Site
Let’s get real. Imagine parsing an e-commerce site like “ShopFast” for pricing trends. The site uses React, so static scraping flops. Using Puppeteer, we’ll scrape product listings.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('https://shopfast.com/deals', { waitUntil: 'networkidle2' });
const deals = await page.evaluate(() => {
const items = Array.from(document.querySelectorAll('.deal-card'));
return items.map(item => ({
title: item.querySelector('.title').innerText,
price: item.querySelector('.price').innerText
}));
});
console.log(deals);
await browser.close();
})();
Running this nets you a JSON of deals. ShopFast’s dynamic loading bowed to Puppeteer’s might. Analyzing 100 products took under a minute—proof parsing can deliver fast insights for lead generation or market research.
Is Dynamic Website Parsing Legal?
A hot question from Google’s “People Also Ask”: Is this legal? Short answer: It depends. Scraping public data—like prices or public posts—is generally fine. But hitting private data, bypassing logins, or overwhelming servers? That’s dicey. Always skim the site’s terms of service.
Ethics matter too. Moz’s scraping guide suggests sticking to fair use—don’t hog resources or republish copyrighted content. Stay sharp, scrape smart, and you’ll dodge legal headaches.
FAQ
How do I scrape dynamic websites effectively?
Use headless browsers or API interception. Test scripts live to match site quirks.
What’s the best tool for beginners?
Octoparse—it’s no-code and handles dynamic content without a steep learning curve.
Can I automate parsing tasks?
Yes! Cron jobs with Scrapy or cloud schedulers keep things humming.
Is coding required?
Not always. SaaS tools like Octoparse let you skip scripts, though coding boosts control.
Conclusion
Dynamic Website Parsing isn’t just a tech trick—it’s a craft. Tools like Selenium or Puppeteer are your brushes, but the masterpiece comes from how you wield them. Each site’s a unique challenge, blending code with curiosity. Whether you’re chasing leads, insights, or just flexing your skills, the real edge lies in adapting—not just automating. Dive in, tweak often, and watch your parsing prowess soar.
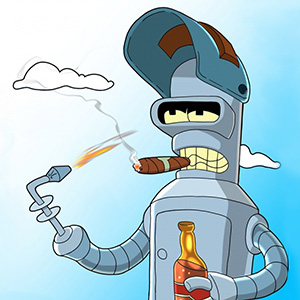
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.