Mastering Dynamic Python Parsing: 7 Proven Techniques to Boost Your Skills
Python developers and enthusiasts often grapple with unpredictable data—messy JSON files, shifting APIs, or convoluted HTML structures begging for order. Dynamic Python Parsing swoops in as the hero, empowering you to wrangle chaos with finesse and precision. This article unpacks expert strategies, serving up actionable insights for those hungry to level up their coding chops. Whether you’re scraping websites, processing real-time streams, or taming rogue datasets, these techniques will transform your approach.
This isn’t just about slapping together some code—it’s about cultivating a mindset. You’ll unearth tools, patterns, and real-world examples that turn parsing from a tedious slog into an art form. Curious about bending Python to your will? Let’s dive into the deep end.
Why Dynamic Parsing Matters in Python
Data doesn’t play nice. APIs morph overnight, user inputs defy logic, and file formats twist into knots—static parsing scripts buckle under the strain. Dynamic parsing, though, thrives in the mess, adapting to surprises without skipping a beat. It’s the backbone of resilient, future-proof code.
For developers, this adaptability slashes debugging time. Picture scraping a site where tags flip daily or parsing logs with rogue delimiters. Nail this skill, and your scripts won’t just survive—they’ll scale. The key benefit? Flexibility that keeps projects humming along, no matter the curveballs.
Beyond survival, it’s about efficiency. Hardcoded solutions crumble when requirements shift, but a dynamic approach bends with the wind. Mastering this isn’t optional—it’s essential for anyone serious about Python.
Unpacking the Basics of Dynamic Python Parsing
So, what’s Dynamic Python Parsing all about? It’s the craft of dissecting and extracting data from structures that refuse to sit still. Unlike rigid parsers tethered to fixed layouts, dynamic methods harness Python’s strengths—think list comprehensions, exception handling, and runtime introspection. Libraries like ast
, json
, and BeautifulSoup
are your trusty sidekicks.
Take a JSON blob with optional keys. A static script chokes on missing fields, but a dynamic one shrugs it off with a quick .get()
check. It’s less about brute force and more about finesse—solving problems with elegance.
Why does this matter? Because real-world data laughs at predictability. From APIs dropping fields to CSV files with surprise headers, dynamic parsing keeps you in control.
- Flexibility: Gracefully handles missing or bonus fields.
- Speed: Cuts manual fixes with smart automation.
- Scalability: Tackles diverse sources without a rewrite.
Technique 1: Leveraging Python’s Built-in Tools
Python’s standard library is a treasure trove for parsing wizards. The json
module, for starters, excels at unpredictable payloads. Toss in try-except
blocks, and you’ve got a safety net for wonky data.
Imagine parsing API responses where fields vanish randomly. Hardcoding keys is a recipe for disaster—use .get()
to pluck values safely, with defaults as backup. Simple, clean, and oh-so-effective.
Don’t sleep on csv
either. It’s perfect for wrangling tabular data with quirks—like extra commas or missing columns. And for text-heavy tasks? re
(regex) slices through patterns like a hot knife.
Here’s a quick win: parsing a log file with re.split(r'\s+', line)
to handle variable whitespace. Built-ins aren’t flashy, but they’re the foundation of dynamic magic.
Technique 2: Harnessing Third-Party Libraries
When built-ins hit their limit, third-party libraries like BeautifulSoup
and lxml
take the stage. Scraping HTML? Soup’s lenient parsing tames even the sloppiest markup. Need raw speed? lxml
chews through XML like it’s nothing.
Picture a webpage rejigging its layout mid-project. A dynamic script with BeautifulSoup
pivots effortlessly, targeting classes or IDs instead of fragile tag paths. It’s forgiving yet powerful—check out BeautifulSoup’s documentation to see why it’s a staple.
Then there’s pandas
for structured data. Parsing a CSV with inconsistent rows? It normalizes chaos into tidy DataFrames. These tools aren’t just add-ons—they’re force multipliers.
Pro tip: Pair requests
with BeautifulSoup
for web scraping. Fetch the page, parse dynamically, and adapt to whatever the server throws your way.
Technique 3: Building Adaptive Regex Patterns
Regex gets a bad rap, but it’s a parsing powerhouse when done right. Crafting patterns that flex with input—like optional prefixes or shifting delimiters—turns raw text into structured gold.
Consider log files with erratic formats. A pattern like r"(\d+)?\s*-\s*(.+)"
snags optional numbers and text, adjusting without a hiccup. It’s not about memorizing syntax—it’s about anticipating variability.
Testing’s key here. Tools like Regex101 let you tweak patterns live, spotting flaws before they bite. Dynamic regex isn’t guesswork—it’s precision engineering.
Start small: parse a phone number with r"\d{3}-?\d{3}-?\d{4}"
to catch dashes or not. Build from there, and you’ll wield regex like a scalpel.
Technique 4: Error Handling Done Right
Errors are inevitable—APIs flake, files corrupt, inputs defy reason. Dynamic parsing shines with smart error handling, keeping your script afloat amid the storm.
Wrap dicey operations in try-except
. Parsing a batch of CSVs where headers vanish? Detect it, log the issue, and soldier on. It’s not about dodging errors—it’s about dancing with them.
Take a JSON feed with rogue encoding. A static script crashes; a dynamic one tries utf-8
, then falls back to latin-1
. Robustness comes from planning for the worst.
Here’s a gem: use logging
over print
for errors. Track issues without cluttering output—your future self will thank you.
Error Type | Dynamic Fix |
---|---|
KeyError | .get(key, default) |
UnicodeDecodeError | Try multiple encodings |
IndexError | Check lengths first |
Technique 5: Recursive Parsing for Nested Data
Nested structures—JSON trees, XML hierarchies—cry out for recursion. A dynamic parser dives through layers, adapting to depth and type without breaking a sweat.
Think of a config file with unknown nesting. A recursive function checks each node, plucking values no matter how deep they’re buried. It’s clean, reusable, and beats manual unrolling hands down.
Here’s the trick: base case first (is it a leaf?), then recurse. Parsing a dict? If it’s got sub-dicts, dive in—otherwise, grab the value. It’s like peeling an onion, but less tearful.
Real-world use? API responses with nested metadata. Recursion turns a tangle into a flat, usable structure—efficiency meets elegance.
Technique 6: How to Achieve Efficiency with Dynamic Parsing
Speed’s non-negotiable. Profiling with time
or cProfile
pinpoints sluggish spots—maybe redundant loops or heavy regex. Optimize smartly, and your parser flies.
Parsing a million-line log? Generators sip memory, processing line-by-line instead of gulping the whole file. Compare that to a list gobbling RAM—night and day.
Caching’s another ace. Repeatedly parsing the same keys? Store them once. Efficiency isn’t a luxury—it’s the difference between a script that runs and one that soars.
Try this: use itertools
for batch processing. It’s Pythonic, fast, and keeps your code lean—perfect for big data headaches.
Technique 7: Testing and Validation Strategies
A parser’s only golden if it’s reliable. Unit tests with unittest
or pytest
catch edge cases—empty files, garbled tags, weird inputs.
Build a test suite with sample data. Validate outputs against expectations, tweaking until it’s bulletproof. Debugging live is a fool’s errand—test early, test often.
Here’s a workflow: mock an API response, parse it, assert the result. Add a malformed version—does it fail gracefully? That’s the gold standard.
Tools like hypothesis
take it further, generating random inputs to stress-test your logic. Reliability isn’t luck—it’s engineered.
Real-World Applications and Examples
Dynamic parsing isn’t academic—it’s your Swiss Army knife. Scraping e-commerce prices from sites with shifting layouts? It adapts, extracts, delivers.
Or ETL pipelines: extracting messy logs, transforming them into clean datasets, loading them into databases. Each step leans on dynamic techniques to handle the unexpected.
APIs are prime turf too. A feed dropping fields mid-flight? Your script shrugs, adjusts, keeps rolling. These aren’t hypotheticals—they’re your next project.
Case in point: parsing Twitter JSON. Nested, inconsistent, wild—yet with recursion and error handling, you’ll tame it into tidy rows.
Alternative Solutions and Trade-Offs
Dynamic parsing isn’t the only game in town. Static parsers—like pyyaml
for fixed YAML—run faster when data’s predictable. But when inputs wobble, they flop.
Manual parsing’s another route—line-by-line, custom logic. It’s precise but slow, and maintenance is a nightmare. Dynamic methods trade setup time for adaptability.
Third-party tools like Scrapy
offer prebuilt power, but they’re heavier than a lean BeautifulSoup
script. Weigh flexibility versus overhead—your call.
Key takeaway? Match the tool to the chaos. Dynamic parsing wins when predictability’s out the window.
Conclusion: Parsing as an Art Form
Dynamic parsing isn’t just a skill—it’s a lens. Marrying adaptability with precision, you sculpt raw data into meaning, turning noise into signal. Each technique here sharpens your craft, arming you for the wildest datasets.
What’s striking is the parallel to problem-solving itself: iterative, curious, relentless. These tools aren’t endpoints—they’re springboards. So, snatch them up, tinker fiercely, and watch your Python mastery ignite. The data’s out there, restless and waiting—go give it a voice.
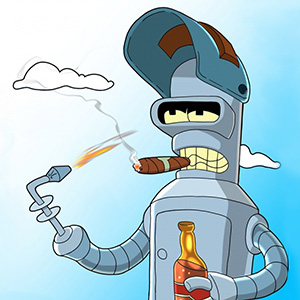
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.