Convert CSV to Excel with Python: Easy Script Guide
Introduction to CSV to Excel Conversion
Manually opening CSV files in Excel, adjusting delimiters, tweaking encodings, and reformatting data can be a time-consuming hassle. What if you could skip all that and convert CSV to Excel with just a few lines of code? That’s where our beginner-friendly Python script comes in—designed to streamline the process effortlessly. Whether you’re dealing with one file or many, this solution saves time and ensures a clean, readable Excel output.
In this guide, we’ll walk you through a simple Python script using Pandas to automate CSV file conversion. No more fiddling with Excel settings—just quick, reliable results!
Why Use Python for CSV Conversion?
Python’s simplicity and powerful libraries like Pandas make it ideal for CSV to Excel conversion. Unlike manual methods, Python automates the process, handling delimiters and encodings behind the scenes. It’s fast, scalable, and perfect for beginners and pros alike. Plus, it saves the output with the original file name, keeping your workflow organized without extra renaming.
Simple Python Script to Convert CSV to Excel
Here’s a beginner-friendly Python CSV script to convert your CSV files to Excel format. It’s straightforward—just import the necessary libraries and specify your file path. The script automatically saves the result in the same folder as your source file.
Step-by-Step Code Breakdown
import pandas as pd
import os
# Set the directory of the project
base_dir = os.path.dirname(__file__)
csv_file = r'C:\DIRECTORY01\your_file_name.csv'
# Create an Excel file name based on the CSV file
excel_file = os.path.splitext(csv_file)[0] + '.xlsx'
# Load the CSV file
data = pd.read_csv(csv_file)
# Save the data in Excel format
data.to_excel(excel_file, index=False) # index=False removes row indexes from the Excel file
How It Works:
- Import Libraries:
pandas
handles the conversion, andos
manages file paths. - Set Paths: Replace
C:\DIRECTORY01\your_file_name.csv
with your CSV’s full path. - Generate Output Name: The script uses the CSV’s name, swapping “.csv” for “.xlsx”.
- Convert:
read_csv
loads the data, andto_excel
saves it as an Excel file.
Run this script, and you’ll get a polished .XLSX file in seconds—faster than any manual method!
Customizing the Script for Multiple Files
Need to process multiple CSV files? Here’s how to tweak the script for batch conversion:
import pandas as pd
import os
# Set the directory containing CSV files
base_dir = os.path.dirname(__file__)
# Loop through all CSV files in the directory
for filename in os.listdir(base_dir):
if filename.endswith('.csv'):
csv_file = os.path.join(base_dir, filename)
excel_file = os.path.splitext(csv_file)[0] + '.xlsx'
data = pd.read_csv(csv_file)
data.to_excel(excel_file, index=False)
print(f"Converted {filename} to {os.path.basename(excel_file)}")
This version scans the folder for all .CSV files, converting each to Excel automatically. It’s perfect for bulk tasks, saving hours of repetitive work.
Benefits of Automating CSV to Excel Conversion
Using a Python script for CSV conversion offers clear advantages:
- Speed: Convert files in seconds, not minutes.
- Ease: No need to adjust delimiters or encodings manually.
- Scalability: Handle one file or hundreds with minimal changes.
- Consistency: Retain original file names for organization.
Whether you’re a data analyst, student, or small business owner, this automation boosts productivity and simplifies workflows.
Conclusion
Converting CSV to Excel doesn’t have to be a chore. With this simple Pandas CSV to Excel script, you can transform files quickly and effortlessly—even if you’re new to Python. From single conversions to batch processing, this solution adapts to your needs, delivering clean, usable Excel files every time.
Try it out, tweak it for your projects, and say goodbye to tedious manual conversions. Automate today and reclaim your time for what matters most!
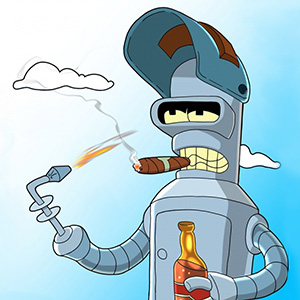
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.