Contact Parsing
Contact parsing refers to the process of extracting contact information from various sources and converting it into a structured format. This allows companies to streamline importing contacts into their CRM or marketing systems. Here’s an in-depth look at what contact parsing involves and its benefits.
What is Contact Parsing
Contact parsing software analyzes unstructured data from documents, webpages, and other sources to identify contact details like names, phone numbers, addresses, and email addresses. It then converts this information into a standardized, structured format.
For example, contact parsing could extract a name, phone number, and email address from a business card or resume PDF. It would identify the relevant information and output it as structured data that could be directly imported into a database.
The parsed contact details typically include:
- Full name – first, middle, last
- Job title
- Company
- Physical addresses – billing, shipping, office locations
- Email addresses
- Phone numbers – cell, home, work
- Social media profiles – Twitter, LinkedIn, Facebook
Why Use Contact Parsing
There are several key reasons companies utilize contact parsing:
Import Leads into CRM
Sales and marketing teams need to constantly add new prospects into their CRM or marketing automation platforms. However, manually entering contact details for each lead is tedious and prone to human error.
Contact parsing allows bulk importing of leads by automatically extracting details from multiple sources and mapping them to the appropriate CRM fields. This saves teams countless hours of manual data entry.
Enrich Existing CRM Records
Many CRM records have incomplete contact data like missing phone numbers or addresses. Contact parsing helps fill in the gaps by pulling additional details from external documents and webpages.
Rather than repeatedly asking customers to update their information, critical contact details can be automatically updated in the background via parsing. This results in more complete and accurate records.
Create Targeted Email Lists
Marketers frequently need to segment their contacts database and create targeted email lists. For example, they may want to send campaigns specifically to IT directors or retail store managers.
By extracting job titles and company names, contact parsing enables marketers to dynamically build lists based on parameters like seniority, department, industry, and so on. This level of segmentation allows highly customized and relevant messaging.
Standardize Inconsistent Data
Contact records from disparate sources often contain inconsistent or invalid data. For example, phone numbers may be formatted differently or names could be incomplete.
Powerful data standardization is a key benefit of contact parsing. It cleans up records by validating and normalizing all extracted contact details into a uniform format. This eliminates duplication and ensures high data quality.
How Contact Parsing Works
Contact parsing relies on sophisticated natural language processing (NLP) and machine learning capabilities. Here is an overview of how it works:
-
Ingest source documents and webpages. These can be PDFs, Word docs, web forms, HTML pages, etc.
-
Identify sections likely to contain contact data using NLP algorithms. For example, focuses on areas with name patterns, address blocks, email addresses, etc.
-
Extract raw contact elements from text via entity recognition and regular expressions.
-
Validate and normalize extracted details. Fixes invalid or duplicate data.
-
Map details to predefined output fields. Aligns names, companies, emails, etc. with target CRM or database fields.
-
Export final parsed contacts into output database or file format like CSV.
Under the hood, contact parsers utilize complex linguistic rules, contextual clues, statistical models, and validation frameworks to accurately identify and extract contacts. The raw output is post-processed to guarantee cleanly structured data.
Customization is often available to target industry-specific contact data or unique source formats. Overall, an advanced parsing engine combines automation with adaptability to handle diverse contact harvesting needs.
Conclusion
Contact parsing automates the laborious process of collecting and standardizing contact data from documents, webpages, and other unstructured sources. For companies looking to enrich their CRM, nurture more leads, and improve marketing efforts, intelligent contact parsing is an invaluable asset. Implementing this technology accelerates lead generation and provides cleaner data to activate sales and marketing strategies.
Summary of Benefits
- Bulk import leads into CRM
- Fill in missing details in records
- Build targeted email lists
- Standardize inconsistent data
- Eliminate manual data entry
By leveraging contact parsing, organizations can populate their databases with new B2B prospects at scale while also improving the quality and completeness of existing contact data. This enables more personalized and effective engagement throughout the customer lifecycle. Contact records stay up-to-date without manual overhead, leading to healthier pipelines and revenue growth.
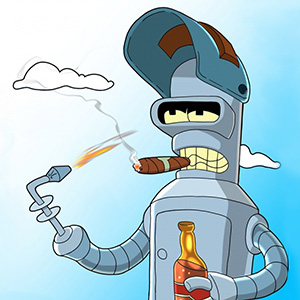
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.