7 Proven Steps to Master Checking Sitemap with Python Like a Pro
Introduction: Why Checking Sitemaps Matters for Developers and SEO Enthusiasts
Sitemaps serve as a roadmap for search engines, directing them through the sprawling lanes of a website’s content. For developers and SEO enthusiasts, mastering checking sitemap techniques with Python isn’t just a skill—it’s a game-changer. This process saves hours, catches errors, and ensures sites remain search-engine-friendly. Tailored for professionals and hobbyists alike, this article delivers practical steps to conquer sitemap analysis with confidence.
Python stands out for its flexibility, wielding libraries like requests
and xml.etree.ElementTree
to slice through sitemap data effortlessly. From spotting broken links to optimizing crawl efficiency, these tools empower you to dig deeper. Expect code snippets, real-world tips, and a dash of creativity to keep you hooked. Let’s dive into the seven steps that’ll transform how you handle sitemaps.
Step 1: Setting Up Your Python Environment for Sitemap Success
A rock-solid setup lays the groundwork for success. Before writing a single line of code, ensure your Python environment is primed for checking sitemap tasks.
Start by installing Python (3.8+ recommended)—most systems have it, but double-check. Then, fetch key libraries: run pip install requests
for downloading sitemaps and pip install lxml
for parsing XML with speed. For quirky HTML sitemaps, toss in pip install beautifulsoup4
. These form your toolkit, versatile yet lightweight.
Isolate your project with a virtual environment: python -m venv sitemap_env
. Activate it—source sitemap_env/bin/activate
on Unix or sitemap_env\Scripts\activate
on Windows—and install your packages. This keeps dependencies tidy, dodging version clashes down the road.
Step 2: Fetching the Sitemap with Python—Where It All Begins
Grabbing a sitemap kicks off the process. Python’s requests
library makes this a breeze, turning a URL into raw data.
Here’s a starter script:
import requests
url = "https://example.com/sitemap.xml"
response = requests.get(url, headers={"User-Agent": "SitemapChecker/1.0"})
if response.status_code == 200:
sitemap_content = response.text
print("Sitemap fetched successfully!")
else:
print(f"Failed to fetch sitemap: {response.status_code}")
This fetches the sitemap and confirms success with a 200 status. A custom User-Agent keeps servers happy. Wrap it in a try-except
block to handle timeouts or 403 errors gracefully. For compressed sitemaps, add response.raw.decode_content = True
. This step exposes a site’s structure—crucial for audits or fixes.
Step 3: Parsing the Sitemap—Turning XML into Actionable Data
With the sitemap in hand, parsing it unlocks its secrets. Python’s xml.etree.ElementTree
is your go-to for this.
Try this:
import xml.etree.ElementTree as ET
root = ET.fromstring(sitemap_content)
urls = [elem.text for elem in root.findall(".//{http://www.sitemaps.org/schemas/sitemap/0.9}loc")]
print(f"Found {len(urls)} URLs!")
This extracts every <loc>
tag into a list. Want more? Grab <lastmod>
or <priority>
tags too. If it’s a sitemap index, loop through <sitemap>
entries and fetch sub-sitemaps. Parsing transforms XML into insights—spotting outdated pages or bloated sections becomes second nature.
Step 4: Validating URLs—Ensuring Your Sitemap Holds Up
A sitemap’s value hinges on its URLs. Validate them to catch issues before they bite.
Use requests.head()
for speed:
for url in urls[:10]: # Sample first 10
try:
resp = requests.head(url, timeout=5)
print(f"{url}: {resp.status_code}")
except requests.RequestException as e:
print(f"{url}: Failed - {e}")
Look for 200s (good), 404s (broken), or 301s (redirects). Log results to a CSV with csv
. For big sitemaps, speed it up with concurrent.futures
. Cross-check with Google’s URL Inspection Tool. Validation weeds out typos or dead links, keeping SEO tight.
Step 5: Analyzing Sitemap Health—Beyond the Basics
Checking sitemap health digs deeper than status codes. Analyze patterns and metadata for a fuller view.
Use pandas
to break it down:
import pandas as pd
df = pd.DataFrame(urls, columns=["URL"])
df["Category"] = df["URL"].str.extract(r"(?:https?://[^/]+)?/([^/]+)")
print(df["Category"].value_counts())
Too many /blog/
URLs? Overloaded priorities? Stale <lastmod>
dates? These signal areas to tweak. This step aligns sitemaps with crawl efficiency, boosting search engine love.
Step 6: Automating the Process—Work Smarter, Not Harder
Manual checks are a slog. Automate them to reclaim your time.
Here’s a framework:
def check_sitemap(url):
# Combine fetch, parse, validate here
return results
import schedule
import time
schedule.every().monday.at("09:00").do(check_sitemap, url="https://example.com/sitemap.xml")
while True:
schedule.run_pending()
time.sleep(60)
Add email alerts with smtplib
for updates. Automation scales effortlessly, letting you focus on strategy over grunt work.
Step 7: Visualizing Results—Making Data Pop
Raw data can bore. Charts make it sing. Use matplotlib
:
import matplotlib.pyplot as plt
status_counts = {"200": 50, "404": 5, "301": 2}
plt.bar(status_counts.keys(), status_counts.values())
plt.title("Sitemap URL Status")
plt.show()
A bar chart highlights 404s instantly. Share it with teams or clients for impact.
Image Description: Bar chart titled ‘Sitemap URL Status’ showing counts of 200, 404, and 301 responses. Alt text: ‘Bar chart visualizing URL status codes from checking sitemap with Python.’
Key Benefits of Checking Sitemaps with Python
Why bother? Here’s the upside:
- Efficiency: Automate grunt work, cutting audit time.
- Precision: Code catches errors humans miss.
- Flexibility: Customize beyond tools like Screaming Frog.
These perks make Python a standout for sitemap mastery.
Conclusion: Elevate Your Sitemap Game with Python
Checking sitemaps with Python isn’t just technical—it’s strategic. Each step sharpens your ability to debug, optimize, and impress. Developers gain precision; SEO pros unearth gold. The real magic? Turning data into decisions that stick. So, tweak these scripts, run them, and watch your workflow soar—because outsmarting the system beats fumbling through it any day.
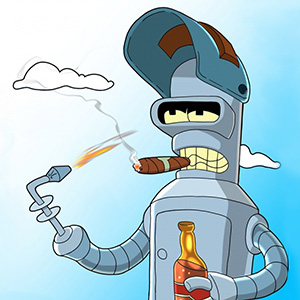
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.