BS4 Parsing
BeautifulSoup (BS4) is a popular Python library used for web scraping purposes. It provides simple methods and Pythonic idioms for navigating, searching, and modifying a parse tree generated from HTML or XML documents. Understanding how to properly parse and extract data using BeautifulSoup is a key skill for web scrapers.
Overview of BeautifulSoup
The BeautifulSoup library allows Python developers to parse HTML and XML documents so they can extract and manipulate data from websites. Key features include:
- Simple API for navigating and searching the parse tree
- Built-in methods for common tasks like extracting tags and attributes
- CSS selectors support for finding elements
- Support for parsing malformed markup
- Integration with popular Python web scraping libraries like Requests and lxml
The name “BeautifulSoup” refers to fixing “ugly” HTML by creating a parsable “beautiful” DOM tree. It provides a robust way of manipulating these trees.
Why Use BeautifulSoup for Parsing?
There are several good reasons why BeautifulSoup is one of the most widely used Python libraries for parsing HTML and XML:
- It has a simple, Pythonic API that is easy to learn. Methods like
.find()
,.find_all()
, and.select()
feel intuitive. - Handling of malformed markup is excellent. BeautifulSoup can create a parsable DOM even for “broken” HTML pages.
- Integration with parsers like lxml and html5lib allows it to parse documents quickly and correctly.
- Methods like
prettify()
help with debugging parsed content. - Active development and community support helps fix bugs and add new features.
Overall, the focus on an easy-to-use API along with robust parsing capabilities has made BeautifulSoup a go-to choice for web scraping.
Parsing HTML and XML with BeautifulSoup
Basic usage of BeautifulSoup involves creating a BeautifulSoup
object, parsing some content, and then extracting information.
Some simple parsing operations like:
- Creating a
BeautifulSoup
object from a HTML document - Using
.find()
to extract elements like<h1>
and<a>
tags - Accessing tag attributes like
href
andclass
BeautifulSoup supports both searching by CSS selectors and traversing/filtering the tree using methods like .find_all()
. Overall, it provides a very handy API for common parsing and extraction tasks.
Tips for Effective Web Scraping with BS4
Here are some tips for writing effective web scrapers using BeautifulSoup:
- Use lxml for fast parsing of large documents.
- Cache/save parsed pages instead of re-parsing on every run.
- Scope searches within meaningful tag sections instead of searching full document.
- Extract data into Python data structures like dicts, lists for easier manipulation.
- Use CSS selectors for clean, targeted extraction.
- Validate extracted content – don’t assume it will be formatted correctly.
- Use methods like
unwrap()
andget_text()
to extract visible text. - Employ throttling, proxies, rotation to avoid overloading target servers.
Mastering tools like BeautifulSoup provides a scalable way to extract large amounts of web data for analytics, monitoring, and automation.
Conclusion
In summary, BeautifulSoup is an essential Python library for parsing, traversing, searching, and modifying HTML and XML documents from the web. It has an intuitive, idiomatic API that allows developers to analyze and extract data from websites with minimal hassle. Combined with other tools like Requests and Selenium, BeautifulSoup makes Python one of the best languages for robust web scraping and automation.
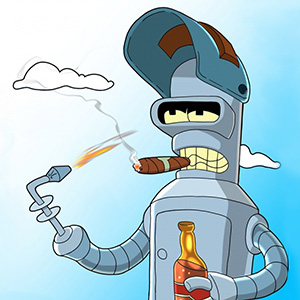
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.