Understanding Beautiful Soup
Beautiful Soup is a Python library that is commonly used for web scraping purposes to extract data from HTML and XML documents. As an experienced web scraper, I often rely on Beautiful Soup to parse through problematic markup and pull out the information I need for projects.
An Overview of Beautiful Soup’s Capabilities
The BeautifulSoup library provides a variety of helpful features for navigating, searching, and modifying parse trees when analyzing web pages. It’s designed to operate on badly formatted code, making it very versatile for handling real-world HTML that doesn’t always follow standards.
Some key things Beautiful Soup allows you to do include:
- Parse documents – Beautiful Soup provides functions and methods for iterating over different tags, extracting text, pulling attribute values and more as you analyze an HTML or XML document that you feed into it.
- Search code – You can home in on specific parts of the parse tree using Beautiful Soup search capabilities based on tags, CSS classes, ID strings, textual content or attributes and values. This helps quickly find what you need.
- Modify documents – Not only can you extract data with Beautiful Soup, but you can manipulate the parse tree – adding/modifying tags and structures as needed.
The main benefits here are that Beautiful Soup automatically handles incorrectly formatted tags that a browser’s rendering engine would fix, provides Pythonic idioms for navigating trees, and allows for rapid experimenting during data extraction.
Installing and Importing Beautiful Soup
Before starting any web scraping project, the first step is making sure Beautiful Soup is available to utilize.
The easiest installation approach is to use pip on the command line:
pip install beautifulsoup4
This will download and configure the latest release.
Then in your Python script, you simply need to import Beautiful Soup with:
python
from bs4 import BeautifulSoup
This allows you to leverage all functionality through the BeautifulSoup
object.
Parsing an HTML Document
Once imported, the primary way you’ll interact with Beautiful Soup is by parsing HTML to create a navigable soup data structure.
This involves just a few lines of code:
import requests
from bs4 import BeautifulSoup
page = requests.get("http://...")
soup = BeautifulSoup(page.content, 'html.parser')
Here we:
- Import
requests
to retrieve page content - Import
BeautifulSoup
- Use
requests
to download the page content - Feed the content into
BeautifulSoup
, specifying thehtml.parser
And that gives us a soup
object to start searching and traversing the DOM!
Searching the Parse Tree
With Beautiful Soup, searches are done using methods like:
find()
– Returns one result matching criteriafind_all()
– Returns list of all matches in iterable
For example:
Search by css class
results = soup.find_all("div", class_="article")
Search by element id
element = soup.find("div", id="introduction")
Search by string
paragraphs = soup.find_all(string="References")
You can also search by other properties like tags, attributes, and custom filters. This allows quickly honing in on pertinent parts of docs.
Extracting Data with Beautiful Soup
Once you’ve searched for patterns and located elements, common next steps are extracting information.
Let’s say we want the text from paragraphs identified earlier:
for p in paragraphs:
print(p.get_text())
This iterates over the matches, calling get_text()
to extract just the text of each.
There are similar methods like:
get()
– Get attribute value from a tagname
– Get tag namestrings
– Iterate over stringscontents
– Iterate child elements
And many more! With these, Beautiful Soup makes pulling data you need out quite straightforward.
Modifying the Parse Tree
In addition to searching and extracting, you may want to adjust or alter parts of the parsed document.
Beautiful Soup allows this through methods like:
append()
– Add tag, string, or other BS object as childnew_tag()
– Create and append new tag into treeinsert()
– Insert a tag/string before another elementwrap()
– Wrap element(s) in another tag
For instance:
new_tag = soup.new_tag("div", id="modification")
soup.body.append(new_tag)
This flexibility helps adjust documents between extraction steps.
Conclusion
In this article, we’ve explored some of Beautiful Soup’s immense capabilities for parsing, traversing, searching, and modifying HTML and XML documents. It’s an indispensable tool for web scraping and programmatically extracting information from the web.
With robust handling of malformed markup and Pythonic idioms for navigating trees, Beautiful Soup makes it easy to isolate and collect data – even from notoriously messy live pages.
Whether mining data for analytics, conducting research, or gathering structured content for other applications, Beautiful Soup delivers. I utilize it daily in my web scraping work to get just what I need from complex sites.
So for sturdy web extraction that handles real-world situations, Beautiful Soup is a go-to solution. Its ability to programmatically rip through problematic HTML makes information gathering simple.
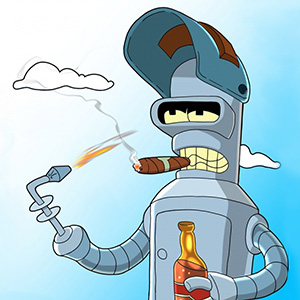
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.