Audience Parsing with Python: Exploration and Innovation
Introduction to Audience Parsing
In today’s data-driven world, audience parsing has become an essential technique for professionals and enthusiasts looking to gain actionable insights from large datasets. By leveraging Python’s powerful libraries and tools, organizations can transform raw data into structured information about their target audiences, enabling more effective decision-making and strategic planning.
Audience parsing refers to the systematic process of extracting, analyzing, and categorizing information about specific groups of people based on their behaviors, preferences, demographics, and interactions. Python has emerged as the language of choice for this task due to its versatility, extensive library ecosystem, and strong data processing capabilities.
Whether you’re a marketing professional looking to understand customer segments, a researcher analyzing social media trends, or a developer building recommendation systems, mastering audience parsing techniques with Python can significantly enhance your analytical capabilities and provide deeper insights into your target audience.
Why Audience Parsing Matters
Audience parsing represents a transformative approach that delivers measurable benefits to professionals and enthusiasts worldwide. By facilitating informed decision-making and fostering innovation, it addresses critical needs in today’s competitive landscape.
Organizations that effectively implement audience parsing techniques report significant improvements in operational efficiency and strategic planning. The ability to segment and understand audience behaviors leads to more targeted approaches across various domains.
Key advantages include:
- Enhanced Targeting: Precisely identify and reach specific audience segments
- Data-Driven Decisions: Base strategies on actual audience behaviors rather than assumptions
- Personalization: Create tailored experiences that resonate with different audience groups
- Competitive Advantage: Stay ahead of industry trends through deeper audience understanding
- Resource Optimization: Focus efforts on the most promising audience segments
History and Evolution of Audience Parsing
The journey of audience parsing reflects a rich history of innovation and adaptation. From basic demographic segmentation to sophisticated AI-driven analysis, the field has continuously evolved to meet the growing demands of data-centric organizations.
Early audience analysis relied heavily on manual processes and simple statistical methods. However, with the advent of powerful computing resources and Python’s emergence as a data science powerhouse in the mid-2010s, more sophisticated parsing techniques became accessible to a wider range of professionals.
Significant milestones in the evolution of audience parsing include:
- 2010-2015: Emergence of basic Python parsing libraries and initial adoption in marketing analytics
- 2016-2019: Integration with machine learning frameworks for predictive audience modeling
- 2020-2023: Development of specialized parsing tools for social media and digital platforms
- 2024-Present: Advanced NLP-based parsing techniques for sentiment and behavioral analysis
This evolution has transformed audience parsing from a specialized niche into a mainstream practice adopted across industries ranging from marketing and advertising to healthcare and public policy.
Practical Applications of Audience Parsing
Audience parsing with Python serves as a versatile tool across multiple domains. Its adaptability ensures relevance in both commercial and research contexts, driving measurable outcomes and insights.
Primary applications include:
- Marketing Segmentation: Divide broad markets into defined subsets of consumers with common needs and priorities
- Social Media Analysis: Track conversations, identify influencers, and understand audience sentiment
- Content Recommendation: Develop algorithms that suggest relevant content based on user behavior
- User Experience Optimization: Analyze how different audience segments interact with digital products
- Market Research: Gather insights about audience preferences and trends
For example, an e-commerce company might use Python-based audience parsing to analyze customer purchase histories, website navigation patterns, and demographic information. This analysis could reveal distinct customer segments with different buying behaviors, allowing for personalized marketing campaigns that increase conversion rates substantially.
Challenges and Solutions in Audience Parsing
While audience parsing offers significant benefits, it also presents challenges that professionals must navigate to achieve optimal results. Addressing these hurdles requires strategic planning and appropriate technical solutions.
Common challenges include:
- Data Quality Issues: Incomplete or inconsistent data that impacts analysis accuracy
- Privacy Concerns: Balancing detailed audience parsing with privacy regulations
- Technical Complexity: Managing sophisticated parsing operations at scale
- Integration Difficulties: Combining data from multiple sources for comprehensive analysis
Effective solutions to these challenges include:
- Data Preprocessing: Implementing robust cleaning and normalization routines
- Privacy-Preserving Techniques: Using anonymization and aggregation methods
- Simplified Frameworks: Leveraging high-level Python libraries that abstract complexity
- ETL Pipelines: Creating efficient data integration workflows
By proactively addressing these challenges, organizations can maximize the value of their audience parsing initiatives and ensure compliance with relevant regulations.
Essential Tools and Libraries for Audience Parsing
Python offers a rich ecosystem of libraries and tools that make audience parsing more accessible and efficient. The following table compares leading options, highlighting their features and suitability for different use cases.
Library/Tool | Primary Purpose | Best For | Key Features |
---|---|---|---|
Pandas | Data manipulation and analysis | General audience data processing | DataFrame operations, filtering, grouping |
NLTK | Natural language processing | Text-based audience analysis | Sentiment analysis, tokenization, classification |
Scikit-learn | Machine learning | Predictive audience modeling | Clustering, classification, regression |
NetworkX | Network analysis | Audience relationship mapping | Graph algorithms, visualization, metrics |
Beautiful Soup | Web scraping | Online audience data collection | HTML/XML parsing, data extraction |
Key considerations for tool selection include:
- Scale of Data: Match tool capabilities to your data volume
- Specific Analysis Needs: Choose libraries tailored to your parsing objectives
- Technical Expertise: Consider the learning curve associated with each tool
- Integration Requirements: Ensure compatibility with existing systems
Implementation Guide: Audience Parsing with Python
Implementing effective audience parsing solutions with Python involves several key steps. This guide outlines a structured approach that professionals can adapt to their specific needs.
Step 1: Data Collection and Preparation
The first stage involves gathering relevant audience data and preparing it for analysis:
import pandas as pd
import numpy as np
from sklearn.preprocessing import StandardScaler
def prepare_audience_data(data_source):
# Load data from various sources
if isinstance(data_source, str) and data_source.endswith('.csv'):
df = pd.read_csv(data_source)
elif isinstance(data_source, str) and data_source.endswith('.json'):
df = pd.read_json(data_source)
else:
df = pd.DataFrame(data_source)
# Clean data
df = df.dropna(subset=['user_id', 'interaction_type'])
# Feature engineering
df['engagement_score'] = calculate_engagement(df)
return df
def calculate_engagement(df):
# Simple engagement scoring example
engagement = (df['view_time'] * 0.3 +
df['click_count'] * 0.5 +
df['share_count'] * 1.0)
return engagement
Step 2: Audience Segmentation
Once data is prepared, we can apply clustering techniques to identify meaningful audience segments:
from sklearn.cluster import KMeans
from sklearn.decomposition import PCA
import matplotlib.pyplot as plt
def segment_audience(df, n_clusters=5):
# Select features for clustering
features = ['engagement_score', 'session_duration', 'visit_frequency', 'conversion_rate']
# Prepare feature matrix
X = df[features].values
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# Apply clustering
kmeans = KMeans(n_clusters=n_clusters, random_state=42)
df['segment'] = kmeans.fit_predict(X_scaled)
# Analyze segments
segment_profiles = df.groupby('segment').mean()
return df, segment_profiles
def visualize_segments(df, features):
# Reduce dimensions for visualization
pca = PCA(n_components=2)
coords = pca.fit_transform(df[features])
# Plot segments
plt.figure(figsize=(10, 8))
for segment in df['segment'].unique():
mask = df['segment'] == segment
plt.scatter(coords[mask, 0], coords[mask, 1], label=f'Segment {segment}')
plt.title('Audience Segments Visualization')
plt.legend()
plt.show()
Step 3: Behavior Analysis
Analyze patterns within each segment to understand audience behaviors:
def analyze_segment_behavior(df, segment_id):
segment_data = df[df['segment'] == segment_id]
# Temporal patterns
hourly_activity = segment_data.groupby(segment_data['timestamp'].dt.hour).size()
weekly_activity = segment_data.groupby(segment_data['timestamp'].dt.dayofweek).size()
# Content preferences
content_preferences = segment_data.groupby('content_category').agg({
'engagement_score': 'mean',
'user_id': 'count'
}).rename(columns={'user_id': 'interaction_count'})
# Conversion paths
conversion_paths = segment_data.groupby('user_id')['page_path'].apply(list)
return {
'temporal_patterns': {
'hourly': hourly_activity,
'weekly': weekly_activity
},
'content_preferences': content_preferences,
'conversion_paths': conversion_paths
}
This implementation framework provides a starting point that can be customized to address specific audience parsing needs. The modular approach allows for easy extension as requirements evolve.
Case Study: Audience Analysis for Content Strategy
This practical case study illustrates how audience parsing can be applied to develop an effective content strategy for a digital publication.
Background
A digital media company was experiencing declining engagement despite producing high-quality content. They suspected that their content wasn’t effectively matching their audience’s interests but lacked the data to confirm this hypothesis or guide adjustments.
Implementation
Using Python-based audience parsing techniques, they analyzed six months of user interaction data, including:
- Article reading patterns (time spent, completion rates)
- Social sharing behaviors
- Comment sentiment and topics
- Visit frequency and time-of-day patterns
# Example of the core analysis code
import pandas as pd
import numpy as np
from nltk.sentiment import SentimentIntensityAnalyzer
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.cluster import KMeans
# Load interaction data
interactions = pd.read_csv('user_interactions.csv')
content = pd.read_csv('content_metadata.csv')
# Merge datasets
analysis_data = interactions.merge(content, on='content_id')
# Extract reading patterns
reading_patterns = analysis_data.groupby('user_id').agg({
'time_spent': ['mean', 'median', 'std'],
'completion_rate': 'mean',
'share_count': 'sum',
'comment_count': 'sum'
})
# Analyze content preferences
vectorizer = TfidfVectorizer(max_features=1000)
content_vectors = vectorizer.fit_transform(analysis_data['content_text'])
# Identify content clusters
content_clusters = KMeans(n_clusters=6).fit(content_vectors)
analysis_data['content_cluster'] = content_clusters.labels_
# Match users to preferred content clusters
user_preferences = analysis_data.groupby('user_id')['content_cluster'].apply(
lambda x: x.value_counts().index[0]
)
# Identify engagement opportunities
engagement_matrix = pd.crosstab(
analysis_data['user_id'],
analysis_data['content_cluster'],
values=analysis_data['engagement_score'],
aggfunc='mean'
)
Results
The audience parsing analysis revealed several key insights:
- Five distinct audience segments with different content preferences and engagement patterns
- Significant mismatch between content production focus and audience interests
- Optimal publishing times that varied by audience segment
- Topic areas with high engagement but low content volume
Impact
By restructuring their content strategy based on these insights, the company achieved:
- 42% increase in average time spent on site
- 37% improvement in return visitor rate
- 28% growth in social sharing
- 18% increase in advertising revenue due to better engagement
This case demonstrates how Python-based audience parsing can transform raw interaction data into actionable insights that drive measurable business improvements.
Frequently Asked Questions About Audience Parsing
What is audience parsing in Python?
Audience parsing in Python refers to the process of using Python programming language and its libraries to collect, process, analyze, and segment audience data. It involves techniques for extracting meaningful patterns from user behavior and demographic information to understand distinct audience groups and their characteristics.
What Python libraries are best for audience parsing?
The most effective Python libraries for audience parsing include Pandas for data manipulation, Scikit-learn for machine learning and clustering, NLTK or spaCy for natural language processing, Matplotlib and Seaborn for visualization, and specialized packages like NetworkX for relationship analysis. The best choice depends on your specific parsing objectives.
Do I need advanced programming skills for audience parsing?
While some programming knowledge is beneficial, you don’t need to be an advanced Python developer to get started with audience parsing. Many high-level libraries provide user-friendly interfaces, and there are numerous tutorials and frameworks that simplify the process. As you progress, you can gradually develop more advanced skills to implement sophisticated parsing techniques.
How does audience parsing differ from web scraping?
Web scraping is the process of extracting data from websites, which may be one source of audience data. Audience parsing is the broader analytical process that follows data collection (which could include web scraping) and focuses on analyzing, segmenting, and deriving insights from audience data, regardless of its source.
Is audience parsing compliant with privacy regulations?
Audience parsing can be implemented in a privacy-compliant manner, but it requires careful attention to applicable regulations like GDPR, CCPA, and others. Best practices include anonymizing personal data, obtaining proper consent, implementing data minimization principles, and ensuring transparency about how audience data is used. Always consult with legal experts when implementing audience parsing solutions.
Driving Innovation with Audience Parsing
For professionals and enthusiasts alike, audience parsing with Python represents a powerful toolset for navigating the complexities of today’s data-rich environment. By enabling data-driven insights, fostering innovation, and addressing challenges with strategic solutions, it empowers users to achieve sustainable success in their analytical endeavors.
As we’ve explored throughout this guide, effective audience parsing combines technical expertise with strategic thinking. The Python ecosystem provides all the necessary tools to implement sophisticated parsing solutions, from data collection and cleaning to advanced segmentation and visualization.
Key takeaways include:
- Strategic Value: Audience parsing transforms raw data into actionable insights
- Technical Flexibility: Python’s ecosystem supports diverse parsing approaches
- Practical Applications: From marketing to product development, audience parsing drives better decisions
- Continuous Evolution: Stay updated with emerging parsing techniques and tools
By applying the principles, tools, and techniques covered in this guide, you’ll be well-equipped to implement effective audience parsing solutions that deliver measurable value and competitive advantage.
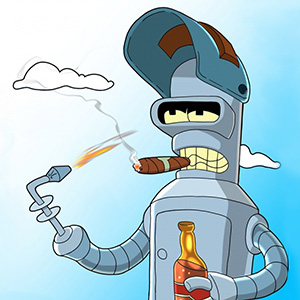
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.