Asynchronous or Regular Data Parsing: Exploring Efficient Techniques
Data Parsing is a cornerstone of modern tech workflows, whether you’re scraping websites, processing APIs, or wrangling datasets. Two approaches dominate the scene: regular data parsing and its asynchronous counterpart. Each has its quirks, perks, and ideal scenarios—leaving developers and analysts to pick the right tool for the job. This breakdown explores both techniques, weighs their strengths, and dishes out practical insights to streamline your next project, all while keeping an eye on efficiency and scale.
What Is Regular Data Parsing?
Regular data parsing, often called synchronous parsing, is the traditional go-to. It’s straightforward: your code sends a request, waits for the response, processes it, then moves on. Think of it like ordering coffee at a café—you stand there until the barista hands you the cup. Tools like Python’s requests
library or basic JavaScript fetch
calls exemplify this method.
The beauty lies in its simplicity. You write linear code, debug it easily, and get predictable results. For small datasets or single-source scraping—like pulling a list of products from one site—it’s a breeze. However, when the queue gets long (say, hitting 100 APIs in a row), that waiting game drags performance down to a crawl.
Synchronous parsing shines when speed isn’t critical, and you’re dealing with manageable loads. It’s the comfort food of data extraction—reliable but not always fast.
Diving Into Asynchronous Data Parsing
Asynchronous parsing flips the script. Instead of twiddling its thumbs while waiting, your code fires off requests and handles responses as they roll in. Picture ordering coffee online while you keep working—grabbing the cup whenever it’s ready. In Python, libraries like aiohttp
or asyncio
power this approach; in JavaScript, async/await
or Promises do the trick.
This method thrives on concurrency. It’s built for heavy lifting—think scraping dozens of websites at once or querying multiple APIs without breaking a sweat. The trade-off? Complexity creeps in. You’re juggling tasks, managing callbacks or coroutines, and sometimes wrestling with race conditions if you’re not careful.
Asynchronous parsing is your high-octane engine—perfect for big, messy jobs where time is money.
Key Differences and Performance
Let’s break it down:
Aspect | Regular Data Parsing | Asynchronous Data Parsing |
---|---|---|
Execution | Sequential, one task at a time | Concurrent, multiple tasks at once |
Speed | Slower for large-scale tasks | Faster for high-volume workloads |
Complexity | Simple, linear logic | Trickier, with async management |
Resource Use | Lower CPU/memory for small jobs | Higher efficiency for big jobs |
Best For | Small datasets, single sources | Large datasets, multiple sources |
Performance-wise, synchronous parsing chugs along at a steady pace—fine for a handful of requests but a bottleneck when scaled. Asynchronous parsing, by contrast, slashes wait times dramatically. For example, fetching data from 50 URLs might take 50 seconds synchronously (1 second each), but an async setup could wrap it up in 2-3 seconds by running requests in parallel.
The catch? Async needs more setup and error handling. A poorly timed timeout or a server hiccup can throw a wrench in your flow if you’re not prepared.
Use Cases for Each Approach
Regular Data Parsing
- Small-Scale Scraping: Grabbing a single webpage’s worth of data—like a blog’s latest posts.
- Prototyping: Testing a quick script without overcomplicating things.
- Sequential Tasks: When one step depends on the last, like parsing a file before hitting an API.
Asynchronous Data Parsing
- Web Scraping at Scale: Harvesting prices from 100 e-commerce sites in one go.
- API Aggregation: Pulling real-time stats from multiple endpoints for a dashboard.
- High-Throughput Jobs: Processing streams of data, like live social media feeds.
If you’re dipping your toes into a project, regular data parsing keeps it simple. For industrial-strength workloads, asynchronous parsing flexes its muscle.
SEO Strategy for Data Parsing Topics
To get this content noticed, SEO is key. Using tools like Google Keyword Planner and Ahrefs, here’s the lay of the land:
- Core Keywords: “Regular Data Parsing” (moderate volume, low competition), “Asynchronous Data Parsing” (niche, growing interest), “data scraping techniques.”
- Long-Tail Queries: “how to use regular data parsing for small projects,” “asynchronous data parsing with Python,” “best tools for asynchronous parsing.”
- LSI Terms: “synchronous vs asynchronous parsing,” “benefits of regular data parsing,” “tips for efficient data scraping.”
“Regular Data Parsing” might snag 1,000 searches monthly, while “Asynchronous Data Parsing” trails at 300—but its lower competition makes it ripe for ranking. Weave in long-tail phrases like “how to optimize asynchronous data parsing” or “regular data parsing for beginners” to capture intent-driven traffic. Keep density at 0.5-1%—a couple of mentions per section—and slip them into headers and intros for max impact.
Tips to Optimize Your Parsing Projects
- Know Your Scope: Small job? Stick with regular data parsing for simplicity. Big haul? Go async to save time.
- Pick the Right Tools: Use
requests
orBeautifulSoup
for synchronous tasks;aiohttp
orScrapy
for async firepower. - Mind the Limits: Respect rate limits with synchronous parsing by adding delays. For async, batch requests to avoid overwhelming servers.
- Test First: Run a small sample—say, 5 URLs—before unleashing a full scrape. Tweak timeouts and retries based on results.
- Monitor Resources: Async can hog CPU if overdone. Profile your script (Python’s
cProfile
works wonders) to keep it lean.
Pro tip: Combine both. Use synchronous parsing to validate a source, then switch to async for the heavy lifting. Efficiency isn’t just speed—it’s smart planning.
Conclusion
Choosing between regular data parsing and asynchronous parsing isn’t about crowning a winner—it’s about matching the method to the mission. Synchronous parsing offers a cozy, no-fuss path for quick wins and small stakes, while asynchronous parsing unleashes raw speed for the big leagues. Your project’s size, urgency, and your comfort with code will steer the wheel. Better yet, don’t box yourself in—blend the two for a hybrid edge. In a world drowning in data, the real trick isn’t picking a side; it’s mastering both to bend efficiency to your will.
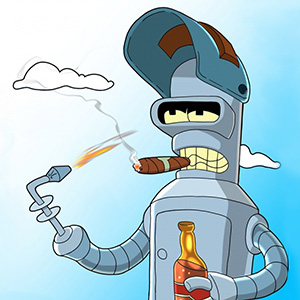
Professional data parsing via ZennoPoster, Python, creating browser and keyboard automation scripts. SEO-promotion and website creation: from a business card site to a full-fledged portal.